How to send Basic Auth with Axios in React & Node ?
Last Updated :
15 Mar, 2024
Basic Auth is a simple authentication scheme. It involves sending a username and password with each request to the server through HTTP. Axios is a popular HTTP client for making requests in JavaScript.
In this article, we will create a React App to demonstrate sending basic Auth with Axios and discuss the following approaches:
In this approach, we use the Axios library to request an HTTP POST to a login endpoint in the components.
Syntax:
const response = await axios.post('http://localhost:5000/login', { username, password });
// This statement should be wrapped inside an async function
Approach 2: Creating an Instance of Axios:
In this approach, we don’t use the axios directly in the component, rather we make an instance of Axios with basic backend details such as base API string and basic headers. This helps avoid rewriting the base URL and headers repeatedly. Then we export the instance to make it usable in other components.
Syntax:
const instance = axios.create({
baseURL: 'http://localhost:5000',
headers: {
'Content-Type': 'application/json',
},
});
export default instance;
// import axios from the exported instance
const response = await axios.post('/login', { username, password });
Steps to Create Basic Express Server to Receive Basic Auth:
We will send the basic auth from frontend using axios. But it will require a server setup to receieve the test request. We will build that server first.
Step 1: Create a folder, enter into it and initialize a node app. Run the following commands to do that
mkdir server
cd server
npm init
Step 2: Install the dependencies to create a server
npm install cors express
Folder Structure(Backend):
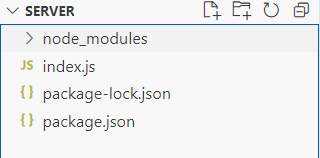
express backend project structure
The updated dependencies in package.json file will look like:
"dependencies": {
"cors": "^2.8.5",
"express": "^4.18.2",
"nodemon": "^3.1.0"
}
Step 3: Open index.js file and write the following code to create a server.
Node
const express = require('express');
const bodyParser = require('body-parser');
const cors = require('cors');
const app = express();
const PORT = process.env.PORT || 5000;
app.use(bodyParser.json());
app.use(cors()); // Add this line to enable CORS
// Endpoint to receive username and password
app.post('/login', (req, res) => {
const { username, password } = req.body;
if (username === 'admin' && password === 'password') {
res.status(200).json({ message: 'Login successful' });
} else {
res.status(401).json({ message: 'Invalid credentials' });
}
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Step 4: Run the server by running the following command in the terminal
node index.js
Steps to Create a React App and Installing Axios
Step 1: Create a new react app and enter into it by running the following commands provided below.
npx create-react-app axios-demo
cd axios-demo
Step 2: Install axios dependency by running the command provided below.
npm install axios
The updated Dependencies in package.json file of frontend will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"axios": "^1.6.7",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Project Structure(Frontend):
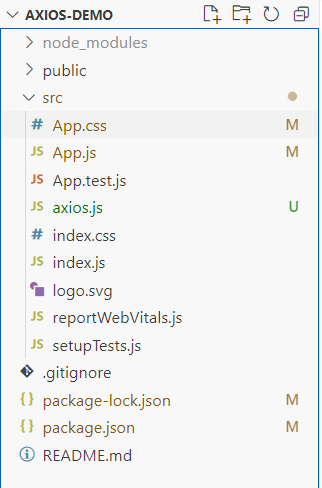
Example 1: Basic Approach with Inline Headers:
In this example, we will send basic auth without creating axios instance.
Javascript
import React, { useState } from 'react';
import axios from 'axios';
function App() {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const [message, setMessage] = useState('');
const handleSubmit = async () => {
try {
const response =
await axios.post('http://localhost:5000/login',
{ username, password });
setMessage(response.data.message);
} catch (error) {
console.error('Error logging in:', error);
setMessage('An error occurred');
}
};
return (
<div style={{ maxWidth: '500px', margin: '0 auto' }}>
<header style={{ padding: '20px 0px', color: "#224F63" }}>
<h1>Testing Username and Password</h1>
</header>
<div style={
{
display: 'flex',
flexDirection: 'column',
marginTop: '20px'
}}>
<input
type='text'
placeholder='Enter Username'
value={username}
onChange={(e) => setUsername(e.target.value)}
style={
{
padding: '20px',
marginBottom: '10px',
border: '1px solid #ccc',
borderRadius: '5px',
fontSize: '16px'
}}
/>
<input
type='password'
placeholder='Enter Password'
value={password}
onChange={(e) => setPassword(e.target.value)}
style={
{
padding: '20px',
marginBottom: '10px',
border: '1px solid #ccc',
borderRadius: '5px',
fontSize: '16px'
}}
/>
<button
onClick={handleSubmit}
style={
{
padding: '20px 20px',
backgroundColor: '#0C73A1',
color: 'white',
border: 'none',
borderRadius: '5px',
cursor: 'pointer',
fontSize: '16px'
}}>
Submit
</button>
{message && <p>{message}</p>}
</div>
</div>
);
}
export default App;
Output: Run the following command to start the server.
npm start
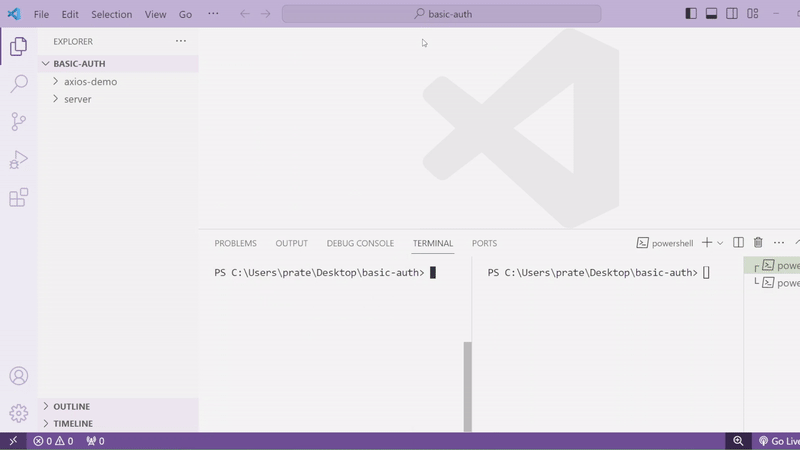
Example 2: Creating an instance of Axios:
In this approach, we will create an instance of Axios and export it. We will import it whenever want to send any requests.
Javascript
// src/App.js
import React, { useState } from 'react';
import axios from './axios';
function App() {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const [message, setMessage] = useState('');
const handleSubmit = async () => {
try {
const response =
await axios.post('/login',
{ username, password });
setMessage(response.data.message);
} catch (error) {
console.error('Error logging in:', error);
setMessage('An error occurred');
}
};
return (
<div style=
{{ maxWidth: '500px', margin: '0 auto' }}>
<header style=
{{ padding: '20px 0px', color: "#224F63" }}>
<h1>Sending Basic Auth With Axios</h1>
</header>
<div style={
{
display: 'flex',
flexDirection: 'column',
marginTop: '20px'
}}>
<input
type='text'
placeholder='Enter Username'
value={username}
onChange={(e) => setUsername(e.target.value)}
style={
{
padding: '20px',
marginBottom: '10px',
border: '1px solid #ccc',
borderRadius: '5px',
fontSize: '16px'
}}
/>
<input
type='password'
placeholder='Enter Password'
value={password}
onChange={(e) => setPassword(e.target.value)}
style={
{
padding: '20px', marginBottom: '10px',
border: '1px solid #ccc', borderRadius: '5px',
fontSize: '16px'
}}
/>
<button
onClick={handleSubmit}
style={
{
padding: '20px 20px', backgroundColor: '#0C73A1',
color: 'white', border: 'none', borderRadius: '5px',
cursor: 'pointer', fontSize: '16px'
}}>
Submit
</button>
{message && <p>{message}</p>}
</div>
</div>
);
}
export default App;
Javascript
// src/axios.js
import axios from 'axios';
const instance = axios.create({
baseURL: 'http://localhost:5000',
headers: {
'Content-Type': 'application/json',
},
});
export default instance;
Run the following command to start the server.
npm start
Output:
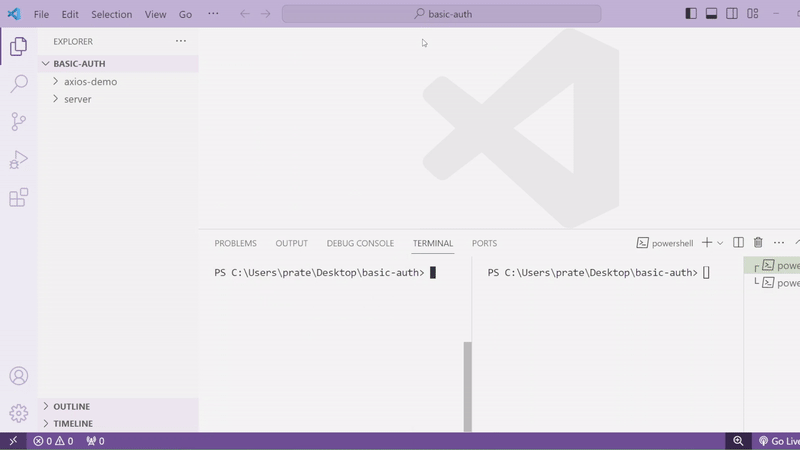
output
Share your thoughts in the comments
Please Login to comment...