How to render an Object in a Sorted order based upon Key in Angular ?
Last Updated :
18 Dec, 2023
An Object is a collection of properties, and a property is an association between a name (or key) and a value. A Property’s value can be a function, in which case the property is known as a method. To achieve this, we can display the object’s properties in a particular order, where the order is determined by the values of a chosen key or property. In this article, we will see the different approaches for rendering an object in a sorted order based on the key in Angular.
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
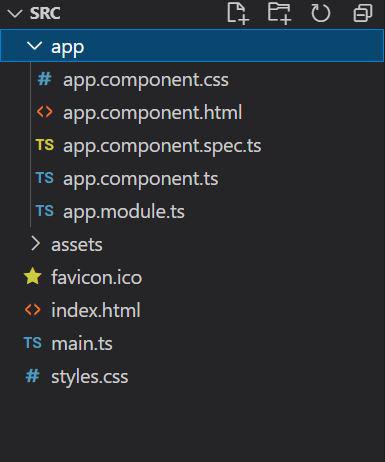
Using keyvalue Approach
In this approach, we have provided objects with keys in a random order like [3,4,2,1]. Now, we will use keyvalue and sort these keys as [1,2,3,4]. We know that keyvalue automatically sorts the object based on keys.
Example 1: This example illustrates the basic rendering of an Object in a Sorted order based upon a Key in Angular.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
How to render an object in a
sorted order based upon key
in Angular
</ h2 >
< div * ngFor = "let item of gfg |keyvalue " >
< b >{{item.key}}</ b >
{{set(item.value)}}
< div * ngFor = "let element of gfg2 |keyvalue " >
< ul >
< li >
< b >{{element.key}}</ b >
{{element.value}}
</ li >
</ ul >
</ div >
</ div >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
import { Pipe, PipeTransform } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg: any =
{
"3" :
{
"course" : "Java" ,
"cost" : "1000"
},
"4" :
{
"course" : "React" ,
"cost" : "1500"
},
"2" :
{
"course" : "Angular" ,
"cost" : "1700"
}
,
"1" :
{
"course" : "CSS" ,
"cost" : "800"
}
}
gfg2: any
set(obj: any) {
this .gfg2 = obj
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output
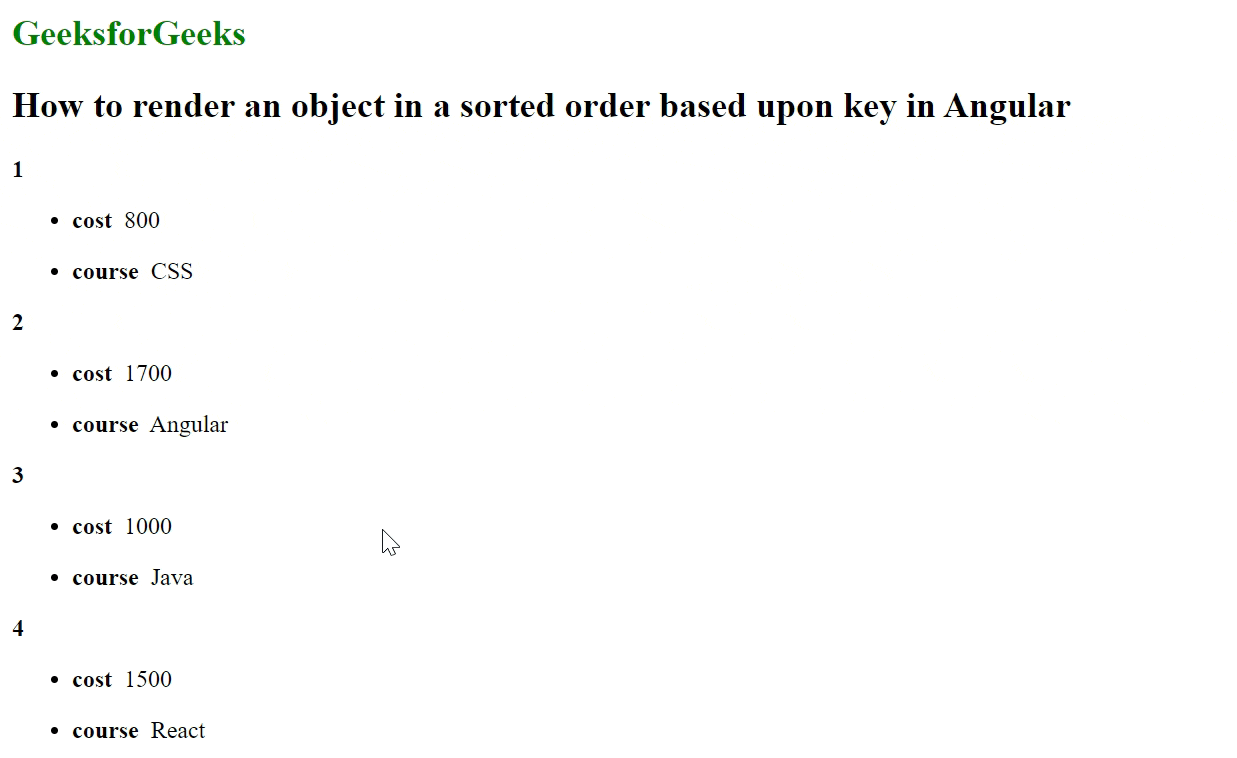
Using sort Function
In this approach, we will sort the function of Arrays.sort and sort the values on ngOnInit. Here, we need to transform the object’s properties into an array and sort that array based on the desired key.
Example: This is another example that illustrates the basic rendering of an Object in a Sorted order based on a Key in Angular.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
How to render an object in a sorted
order based upon key in Angular
</ h2 >
< div * ngFor = "let item of gfg" >
< div * ngFor = "let element of item|keyvalue" >
< b >Key: </ b >{{element.key}}
< b >Value : </ b >{{element.value}}
</ div >
</ div >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
import { Pipe, PipeTransform } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit {
gfg: any =
[
{
"3" : "Java" ,
"4" : "React" ,
"2" : "C++" ,
"1" : "HTML" ,
"7" : "Python" ,
"9" : "CSS" ,
"8" : "Javascript" ,
"5" : "Angular"
}
]
ngOnInit() {
this .gfg.sort();
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
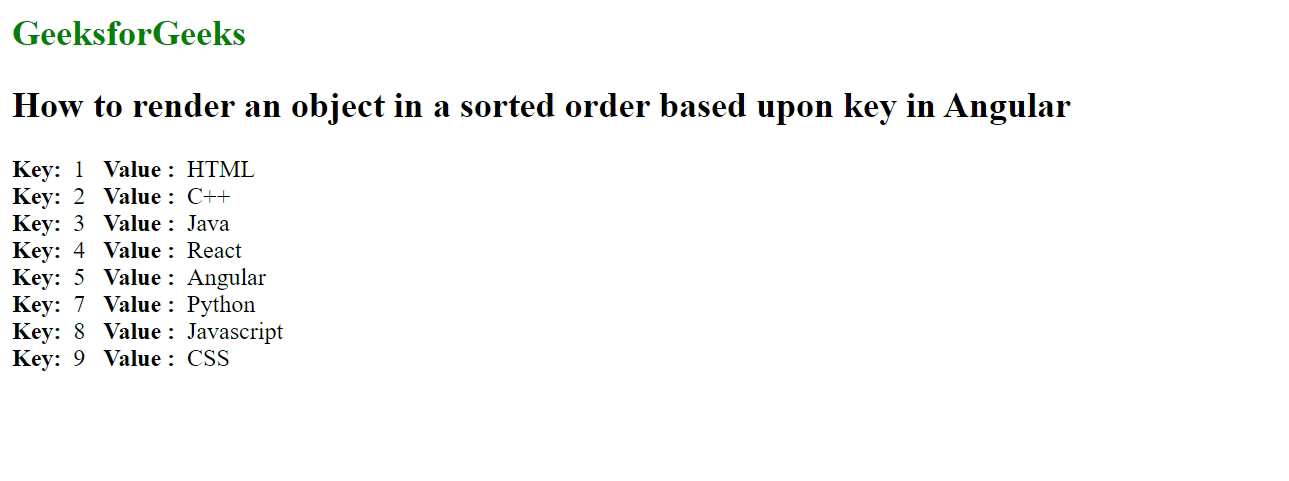
Share your thoughts in the comments
Please Login to comment...