How to render an Object in a Reverse Sorted/Descending order based upon key in Angular ?
Last Updated :
07 Dec, 2023
An Object is a collection of properties, and a property is an association between a name (or key) and a value. A Property’s value can be a function, in which case the property is known as a method. Here, the sorting for an object will be done in descending order based on the key of the object.
In this article, we will see How to render an object in a reverse sorted /descending order in Angular, along with understanding their basic implementation with the help of examples.
Creating Angular application & Module Installation
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
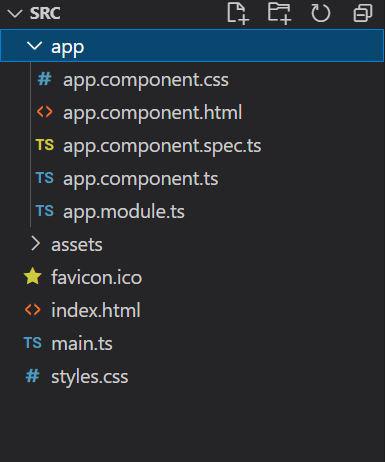
Rendering an object in a reverse sorted /descending order using keyvalue Pair
In this approach, we are using keyvalue Pair, where we have provided objects with keys in order like [1,2,3,4]. Now, we will use keyvalue and sort these keys in reverse as [4,3,2,1]. This can be done by overloading a function called reverseOrder and then implementing it in ts file and returning -1 from there.
Example: This example illustrates the displaying of an object in a reverse sorted /descending order based on key.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
How to render an object in a reverse
sorted /descending order based upon
key in Angular
</ h2 >
< div * ngFor = "let item of gfg |keyvalue :reverseOrder" >
< b style = "color:red" >
{{item.key}}
</ b >
{{set(item.value)}}
< div * ngFor = "let element of gfg2 |keyvalue " >
< ul >
< li >
< b >{{element.key}}</ b >
{{element.value}}
</ li >
</ ul >
</ div >
</ div >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
import { Pipe, PipeTransform } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg: any =
{
"1" :
{
"course" : "Java" ,
"cost" : "1000"
},
"2" :
{
"course" : "React" ,
"cost" : "1500"
},
"3" :
{
"course" : "Angular" ,
"cost" : "1700"
}
,
"4" :
{
"course" : "CSS" ,
"cost" : "800"
}
}
gfg2: any
set(obj: any) {
this .gfg2 = obj
}
console = console
reverseOrder =
(x: KeyValue<string, any>, y: KeyValue<string, any>): number => {
return -1
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
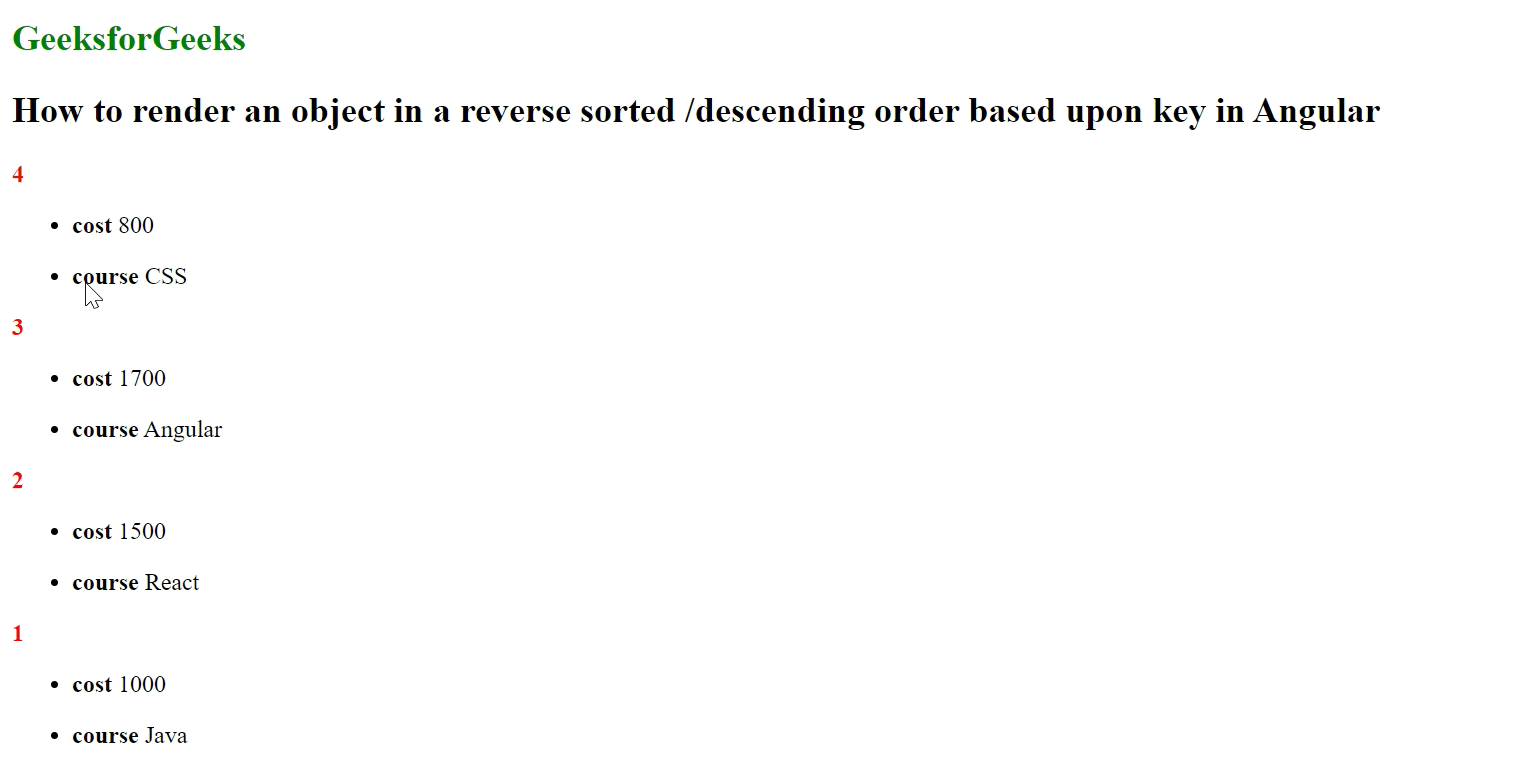
Rendering an object in a reverse sorted /descending order using the sort function
In this approach, we are creating a sort function. This function will sort the values in descending order based on keys. Also, this is done on controller initialization using ngOnInit. Here we had keys in any random order like [3,4,2,1,7,9,8,5]. Output is like [9,8,7,5,4,3,2,1].
Example: This is another example that illustrates the displaying of an object in a reverse sorted /descending order based on key.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
How to render an object in a reverse
sorted /descending order based
upon key in Angular
</ h2 >
< div * ngFor = "let item of gfg" >
< b >Key: </ b >{{item.key}}
< b >Value : </ b >{{item.value}}
</ div >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
import { Pipe, PipeTransform } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit {
gfg: any =
[
{ key: "3" , value: "Java" },
{ key: "4" , value: "React" },
{ key: "2" , value: "C++" },
{ key: "1" , value: "HTML" },
{ key: "7" , value: "Python" },
{ key: "9" , value: "CSS" },
{ key: "8" , value: "Javascript" },
{ key: "5" , value: "Angular" }
]
gfg2: any
console = console
ngOnInit() {
this .gfg.sort( function (a: any, b: any) {
return b.key - a.key;
})
console.log( this .gfg)
}
keepOrder() {
return 0;
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
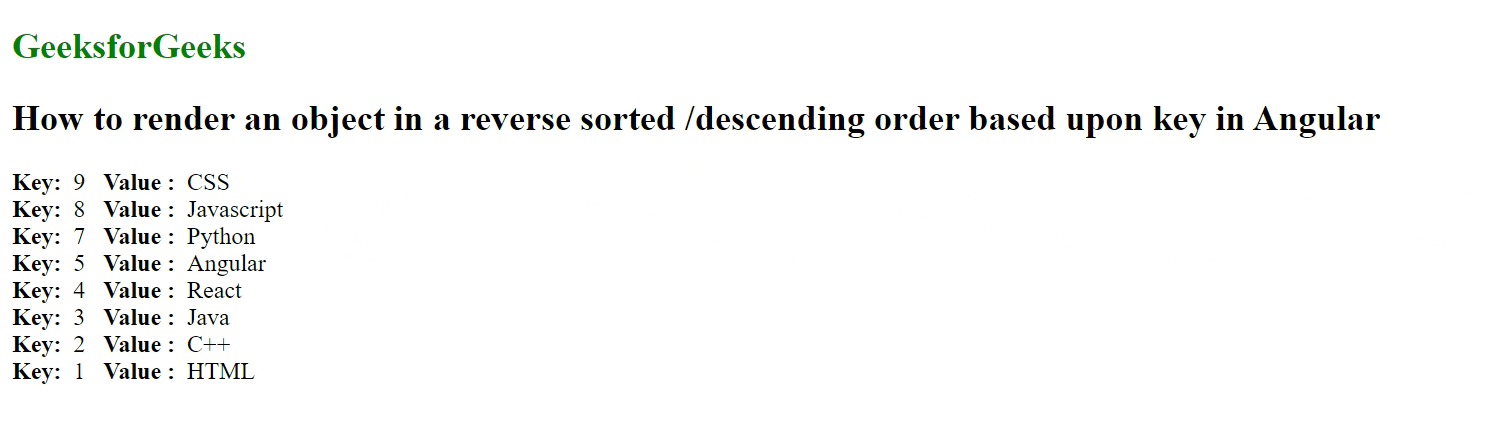
Share your thoughts in the comments
Please Login to comment...