How to Remove Focus from Input Field in TypeScript ?
Last Updated :
12 Mar, 2024
Removing the focus from an input field is a common requirement in web development. This can be useful in scenarios like form validation, user interactions, or controlling focus behavior. The below approaches can be used to achieve this task in TypeScript:
Using the blur() method
This approach involves directly calling the blur() method on the input field to remove focus.
- We use the HTMLInputElement type for the input variable, indicating it’s an input element, and the HTMLButtonElement for the button variable, indicating it’s a button element.
- We add type annotations for the variables input and button to specify their types explicitly.
- We also perform a null check to ensure that the elements are found before adding event listeners.
Example: The below code will show you how to use blur() method remove focus from input fields in TypeScript.
HTML<!-- index.html file -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body {
text-align: center;
}
</style>
</head>
<body>
<h1 style="color: green">
GeeksForGeeks
</h1>
<h3>
Remove focus from input field
in TypeScript
</h3>
<input id="myInput" type="text"
placeholder="Enter some text here" />
<br /><br />
<button id="myBtn">
Click to remove focus from input
</button>
</body>
</html>
Javascript// index.ts file
const input: HTMLInputElement | null =
document.querySelector("#myInput");
const button: HTMLButtonElement | null =
document.querySelector("#myBtn");
if (input && button) {
button.addEventListener("click", () => {
input.blur();
});
}
Output:
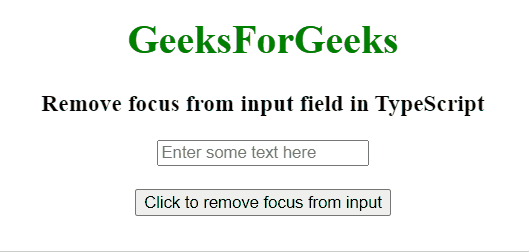
Setting focus on another element
This approach involves setting focus to another element to indirectly remove focus from the input field:
- We use type annotations for the input and button variables, specifying their respective HTML element types as HTMLInputElement and HTMLButtonElement.
- We use the as keyword for type assertion to tell TypeScript that the elements retrieved by document.getElementById() are of the expected types.
- We perform a null check to ensure that the elements are found before adding the event listener.
- We utilize the window.onload event to ensure that the script runs after the DOM is fully loaded.
Example: The below code will explain how to set focus on another element and remove focus from input field.
HTML<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body {
text-align: center;
}
</style>
</head>
<body>
<h1 style="color: green">
GeeksForGeeks
</h1>
<h3>
Remove focus from input field
in TypeScript
</h3>
<input id="myInput" type="text"
placeholder="Enter some text here" />
<br /><br />
<button id="myBtn">
Click to remove focus from input
</button>
</body>
</html>
Javascript// index.ts
window.onload = function () {
const input: HTMLInputElement | null = document.
getElementById("myInput") as HTMLInputElement;
const button: HTMLButtonElement | null = document.
getElementById("myBtn") as HTMLButtonElement;
if (input && button) {
button.addEventListener("click", () => {
button.focus();
});
}
};
Output:
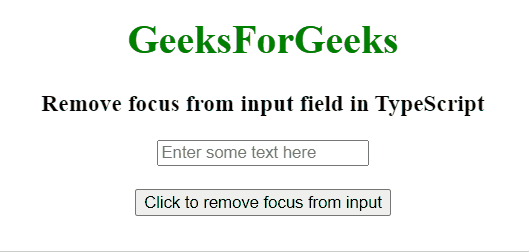
Share your thoughts in the comments
Please Login to comment...