How to read or input a string?
Last Updated :
10 Feb, 2024
In this article, we are going to learn how to print or output a string using different languages. Strings are considered a data type in general and are typically represented as arrays of bytes (or words) that store a sequence of characters. Strings are defined as an array of characters.
Topics:
Language Used
|
Functions or Classes
|
How to Read or Input a String in C
We can use the scanf() function to read a string in C language. It takes the string as an argument in double quotes (” “) with the help of “%s”.
Below is the code for more reference:
C
#include <stdio.h>
int main()
{
char str[13];
printf ("Input String: ");
scanf ("%s", str);
printf ("\nEntered String: ");
printf ("\"%s\"", str);
return 0;
}
|
Output:
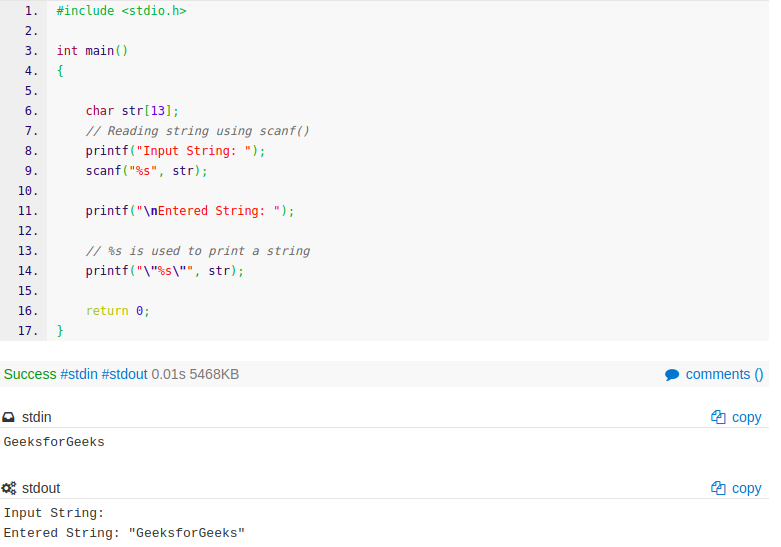
How to Read or Input a String in C++
To Read the string, just place the string (the variable that stores the string’s value) after cin>>, as shown here in the following program.
Below is the code for more reference:
C++
#include <iostream>
using namespace std;
int main()
{
string str;gfg
cout << "Input String: ";
cin >> str;
cout << "String: ";
cout << str;
return 0;
}
|
Output:
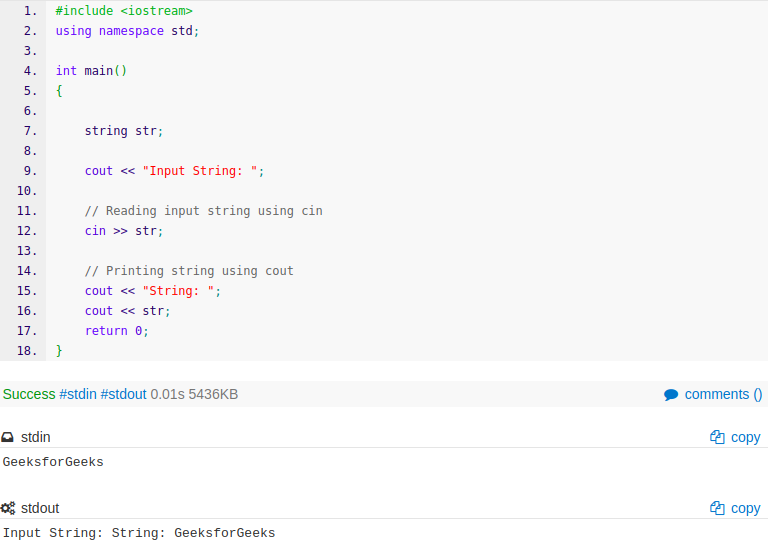
How to Read or Input a String in Java
In Java, we can use Scanner Class or InputStream to Read the string.
In below code, we will try to use the Scanner class instance to read the string.
Java
import java.io.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
String str;
Scanner sc = new Scanner(System.in);
str = sc.nextLine();
System.out.print("String: ");
System.out.println(str);
}
}
|
Output:
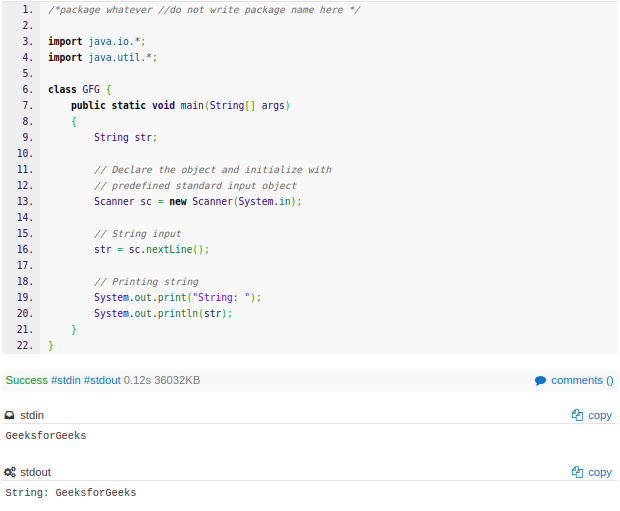
How to Read or Input a String in C#
A string is represented by class System.String. The “string” keyword is an alias for System.String class and instead of writing System.String one can use String which is a shorthand for System.String class.
Now to Read the String in C#, we need to use the Console.ReadLine() method, as shown below:
C#
using System;
public class GFG {
static public void Main()
{
String str;
str = Console.ReadLine();
Console.Write("String: {0}", str);
}
}
|
Output:
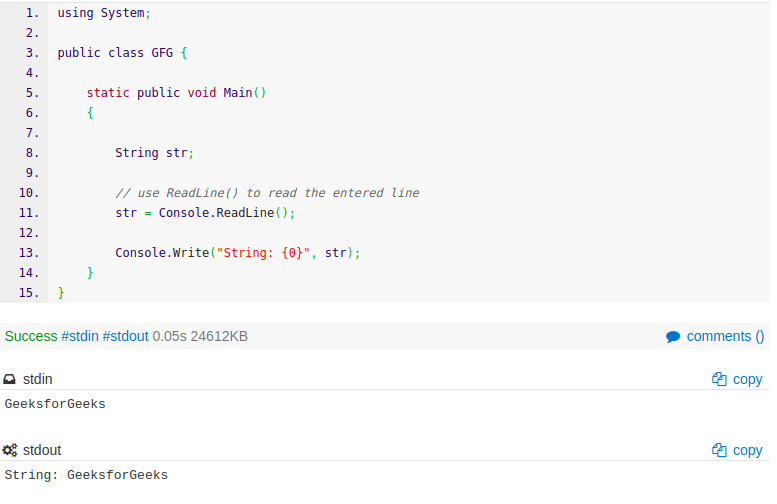
How to Read or Input a String in Python
Python input() function first takes the input from the user and converts it into a string. The type of the returned object always will be <class ‘str’>.
Python3
name = input (" Input String: ")
print ("String: ", name)
|
Output:
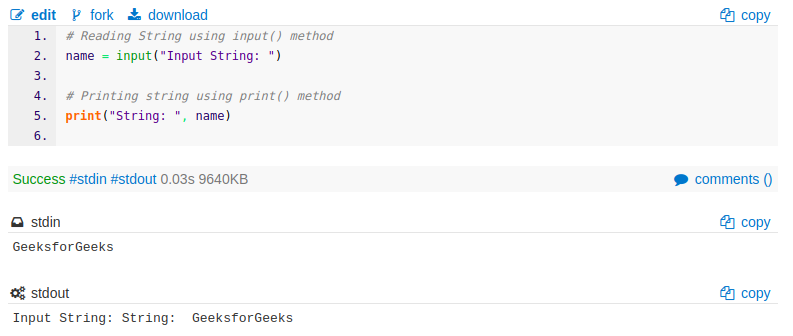
Reading Text Input in JavaScript
JavaScript provides various methods for capturing user input, especially when dealing with text. Here, we’ll explore two common approaches: using the prompt function for simplicity and creating an HTML input element for a more interactive user experience.
1. Using prompt for Basic Input:
The prompt function allows you to gather simple text input from the user in a browser environment.
Javascript
let userInput = prompt( "Enter Text:" );
console.log( "User Input:" , userInput);
|
Output:
GeeksforGeeks
Share your thoughts in the comments
Please Login to comment...