How to migrate from create-react-app to Vite?
Last Updated :
02 Nov, 2023
In this article, we will learn how we can migrate from create-react-app to Vite. Vite is a build tool for frontend development that aims for fast and efficient development. It provides a development server with hot module replacement and supports various JavaScript flavors, including JSX, out of the box. In this guide, we’ll explore the steps to transition from a Create React App project to a Vite-based setup.
Steps to create react app using vite:
Step 1: Start with creating a react app with the following command
npx create-react-app vite
Step 2: Move inside main project
cd vite
Step 3: Uninstall React Script by using following command
npm uninstall react-scripts
Step 4: Now Install Vite Dependencies through given command
npm install vite @vitejs/plugin-react-swc vite-plugin-svgr
Note: Depending on your needs, you can explore different plugins from the official Vite plugins documentation.
Step 5: Add the script tag in your index.html as shown in the given command. Also bring index.html to root directory.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" />
< meta name = "viewport"
content = "width=device-width, initial-scale=1" />
< meta name = "theme-color"
content = "#000000" />
< meta name = "description"
content = "Web site created using create-react-app" />
< title >React App</ title >
</ head >
< body >
< noscript >
You need to enable JavaScript to run this app.
</ noscript >
< div id = "root" ></ div >
< script type = "module" src = "./src/index.jsx" ></ script >
</ body >
</ html >
|
Step 6: Change the name of following files
Index.js -> Index.jsx
App.js -> App.jsx
Step 7: Create a file vite.config.js in the root directory of your project and paste the given code in that file.
Javascript
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react-swc'
export default defineConfig({
plugins: [react()],
})
|
The Project Structure will look like:
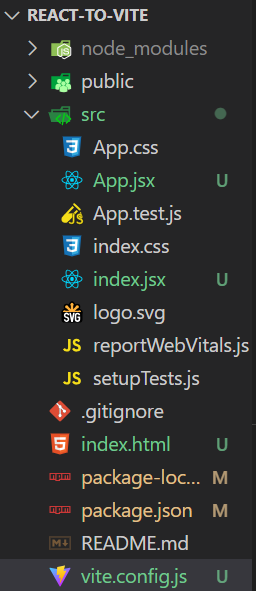
Project Structure
Step 8: Update the script in Package.json with the given vite code.
"scripts": {
"start": "vite",
"build": "vite build",
"serve": "vite preview"
}
Step 9: Copy the given code to App.jsx
Javascript
import { useState } from "react" ;
import logo from "./logo.svg" ;
import "./App.css" ;
function App() {
const [count, setCount] =
useState(0);
return (
<>
<div>
<a
target= "_blank" >
<img
src={logo}
className= "logo react"
alt= "React logo"
style={{
width: "200px" ,
}}/>
</a>
</div>
<h1>Vite + React</h1>
<div className= "card" >
<button
onClick={() =>
setCount(
(count) =>
count +
1
)}>
count is {count}
</button>
<p>
Edit{ " " }
<code>
src/App.jsx
</code>{ " " }
and save to test HMR
</p>
</div>
<p className= "read-the-docs" >
Click on the Vite and
React logos to learn
more
</p>
</>
);
}
export default App;
|
Step to run the application:
Step 1: Write the following command in your terminal
npm run start
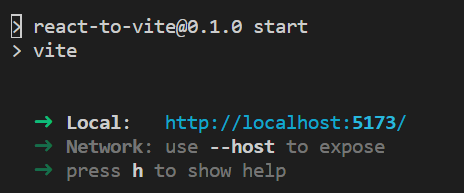
example
Step 2: Browse the following URL
http://localhost:5173/
Output:
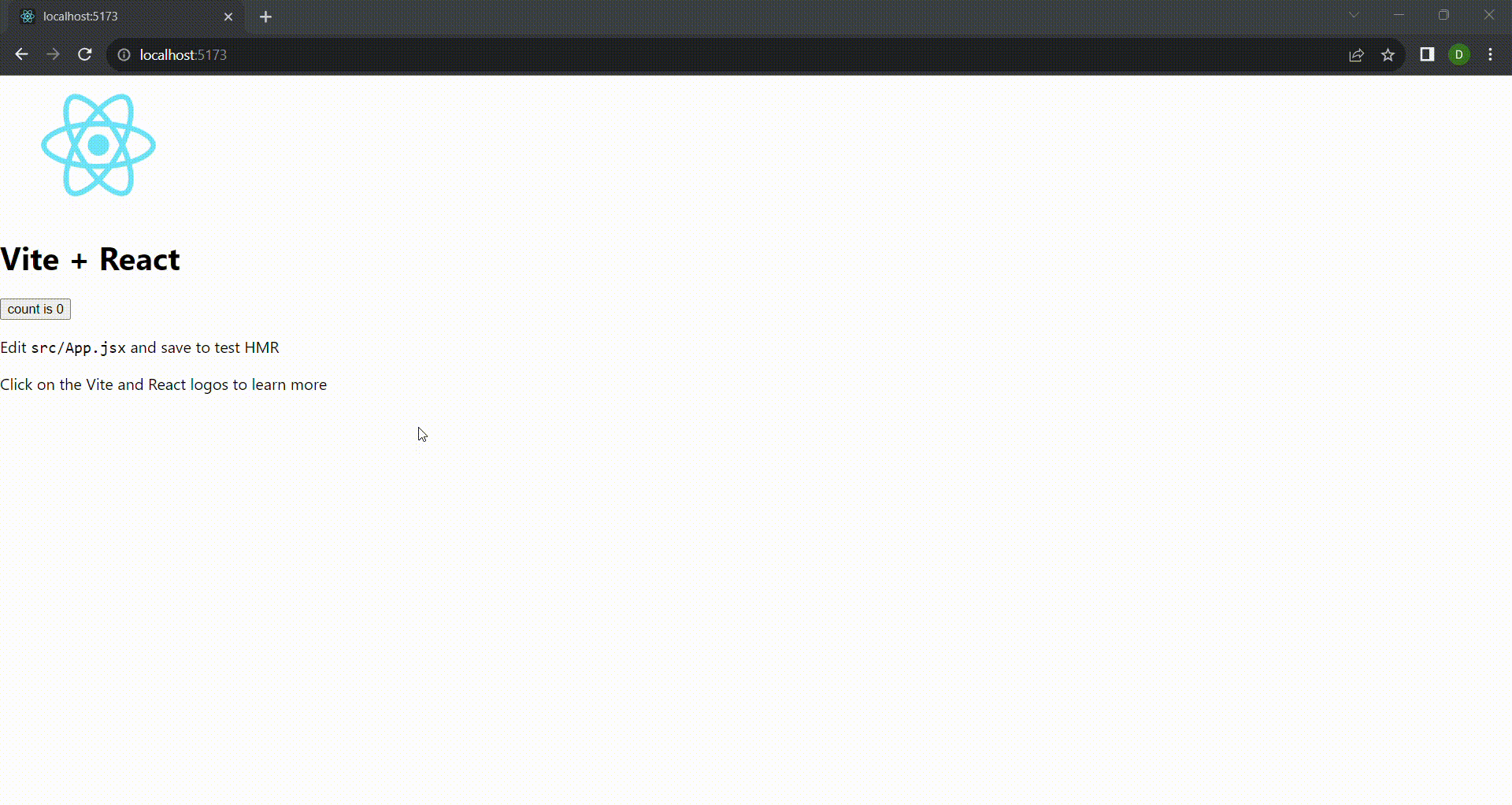
Share your thoughts in the comments
Please Login to comment...