How to make a Round Slider using JavaScript ?
Last Updated :
22 Mar, 2024
Round Slider is the UI element that allows us to select a value by dragging a handle along the circumference of the circular track.
The below approaches can be used to accomplish this task.
Using Canvas
In this example, we’re creating a round slider using the HTML5 Canvas element. The slider allows us to interact by dragging the handle, by updating its angle dynamically. The current value of the slider is displayed in the center of the canvas.
Example: The below code example uses the Canvas element to create a round slider in JavaScript.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<style>
#sContainer {
width: 200px;
height: 200px;
position: relative;
margin: auto;
}
#sValue {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
font-family: Arial, sans-serif;
font-size: 16px;
}
</style>
</head>
<body style="text-align: center;">
<h3>
Creating round slider using Canvas
</h3>
<div id="sContainer">
<canvas id="slider" width="200"
height="200"></canvas>
<div id="sValue"></div>
</div>
<script>
const canvas =
document.getElementById('slider');
const ctx = canvas.getContext('2d');
const val =
document.getElementById('sValue');
const x = canvas.width / 2;
const y = canvas.height / 2;
const r = 80;
let a = Math.PI / 4;
drawFn();
valFn();
canvas.addEventListener('mousedown', dragFn);
canvas.addEventListener('mousemove', drag);
canvas.addEventListener('mouseup', endFn);
function drawFn() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.beginPath();
ctx.arc(x, y, r, 0, Math.PI * 2);
ctx.strokeStyle = 'green';
ctx.lineWidth = 20;
ctx.stroke();
ctx.beginPath();
const handleX = x + Math.cos(a) * r;
const handleY = y + Math.sin(a) * r;
ctx.arc(handleX, handleY, 8, 0, Math.PI * 2);
ctx.fillStyle = 'red';
ctx.fill();
}
function valFn() {
const value = Math.round(a * 180 / Math.PI);
val.textContent = `Value: ${value}`;
}
let temp = false;
function dragFn(e) {
temp = true;
drag(e);
}
function drag(e) {
if (!temp) return;
const rect = canvas.getBoundingClientRect();
a = Math.atan2(e.clientY - rect.top - y,
e.clientX - rect.left - x);
drawFn();
valFn();
}
function endFn() {
temp = false;
}
</script>
</body>
</html>
Output:
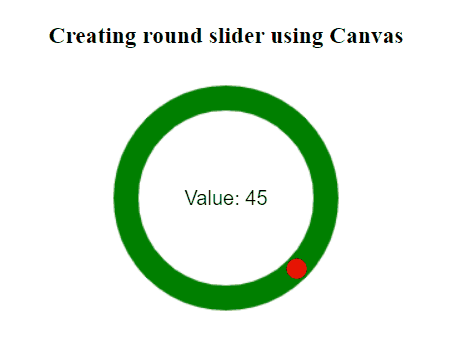
Using jQuery
In this example, we are creating a round slider using JavaScript with jQuery, allowing users to adjust a handle position along the circumference of a circular container. The range value from -100 to 100 is displayed based on the handle’s position.
Example: The below code uses jQuery to create round slider.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 2</title>
<style>
.sContainer {
margin: auto;
position: relative;
width: 200px;
height: 200px;
border-radius: 50%;
background: rgb(255, 253, 110);
overflow: hidden;
}
.sContainer::before {
content: "";
position: absolute;
width: 160px;
height: 160px;
background-color: white;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
border-radius: 50%;
}
.sHandle {
position: absolute;
top: 50%;
left: 50%;
width: 20px;
height: 20px;
background: #ff0000;
border-radius: 50%;
transform: translate(-50%, -50%);
cursor: pointer;
}
</style>
</head>
<body style="text-align: center;">
<h3>
Creating round slider using jQuery
</h3>
<div class="sContainer" id="sliderContainer">
<div class="sHandle" id="sliderHandle"></div>
</div>
<p id="rangeValue"></p>
<!-- jQuery CDN -->
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<script>
$(document).ready(function () {
const sContainer = $('#sliderContainer');
const sHandle = $('#sliderHandle');
const rVal = $('#rangeValue');
let a = 0;
function updateFn(event) {
const rect = sContainer[0].
getBoundingClientRect();
const x = rect.left + rect.width / 2;
const y = rect.top + rect.height / 2;
const dx = event.clientX - x;
const dy = event.clientY - y;
a = Math.atan2(dy, dx);
a = a < 0 ? a + 2 * Math.PI : a;
updateHFn();
updateRValFn();
}
function updateHFn() {
const r =
sContainer.width() / 2 - sHandle.width() / 2;
const x =
Math.cos(a) * r + sContainer.width() / 2;
const y =
Math.sin(a) * r + sContainer.height() / 2;
sHandle.css({ left: x, top: y });
}
function updateRValFn() {
const val = Math.
round((a / (2 * Math.PI)) * 200) - 100;
rVal.text('Range Value: ' + val);
}
sHandle.on('mousedown', function (event) {
event.preventDefault();
$(document).on('mousemove', updateFn);
});
$(document).on('mouseup', function () {
$(document).off('mousemove', updateFn);
});
updateHFn();
updateRValFn();
});
</script>
</body>
</html>
Output:
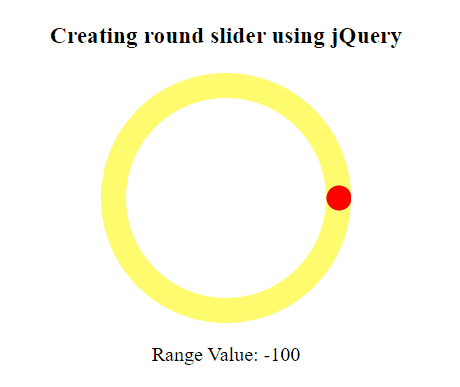
Share your thoughts in the comments
Please Login to comment...