Automatic Image Slider using JavaScript
Last Updated :
22 Dec, 2023
In this article, we will discuss how to create an automatic image slider using JavaScript. An image slider is a popular component of modern websites that allows the display of multiple images in a single location, usually in a sliding motion. With the use of JavaScript, creating an automatic image slider has become much simpler.
Preview:
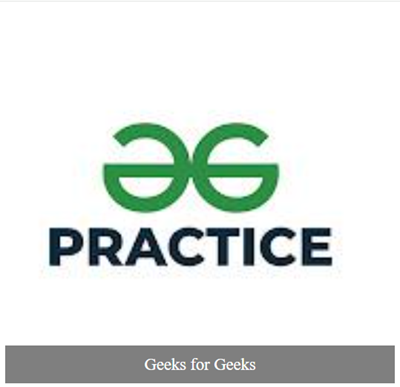
Approach:
- The first step is to create the HTML markup for the image slider. The markup will contain an unordered list (<ul>) that will hold all the images. Each list item (<li>) will have an image and a caption.
- Now, grab the HTML elements using their classes and IDs to style them using CSS styling.
- Select the slider element and all the slide elements using the querySelectorAll() method.
- Store the total number of slides in the slideCount variable and set the initial active slide to 0.
- Use the setInterval() method to execute the anonymous function every 5000 milliseconds (or 5 seconds). Inside the function, we remove the ‘active’ class from the current slide and increment the activeSlide variable by 1.
- If the activeSlide variable is equal to the slideCount, we reset it back to 0.
Example: The below example explains how you can use JavaScript to create an automatic image slider.
Javascript
const slider = document.querySelector( '.slider' );
const slides = slider.querySelectorAll( 'li' );
const slideCount = slides.length;
let activeSlide = 0;
setInterval(() => {
slides[activeSlide].classList.remove( 'active' );
activeSlide++;
if (activeSlide === slideCount) {
activeSlide = 0;
}
slides[activeSlide].classList.add( 'active' );
}, 2000);
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >Image Slider</ title >
< link rel = "stylesheet"
href = "style.css" >
</ head >
< body >
< center >
< div class = "slider" >
< ul >
< li >
< img src =
alt = "GFG Image 1" >
< div class = "caption" >
Geeks for Geeks
</ div >
</ li >
< li >
< img src =
alt = "GFG Image 2" >
< div class = "caption" >
Geeks for Geeks
</ div >
</ li >
< li >
< img src =
alt = "GFG Image 3" >
< div class = "caption" >
Geeks for Geeks
</ div >
</ li >
< li >
< img src =
alt = "GFG Image 4" >
< div class = "caption" >
Geeks for Geeks
</ div >
</ li >
</ ul >
</ div >
</ center >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
.slider {
width : 100% ;
position : relative ;
}
.slider ul {
list-style : none ;
margin : 0 ;
padding : 0 ;
}
.slider li {
position : absolute ;
top : 0 ;
left : 0 ;
opacity: 0 ;
transition: opacity 1 s ease-in-out;
}
img {
height : 400px ;
width : 400px ;
}
.slider li.active {
opacity: 1 ;
}
.slider . caption {
position : absolute ;
bottom : 0 ;
left : 0 ;
width : 100% ;
background-color : rgba( 0 , 0 , 0 , 0.5 );
color : #fff ;
font-size : 18px ;
padding : 10px ;
text-align : center ;
}
|
Output:
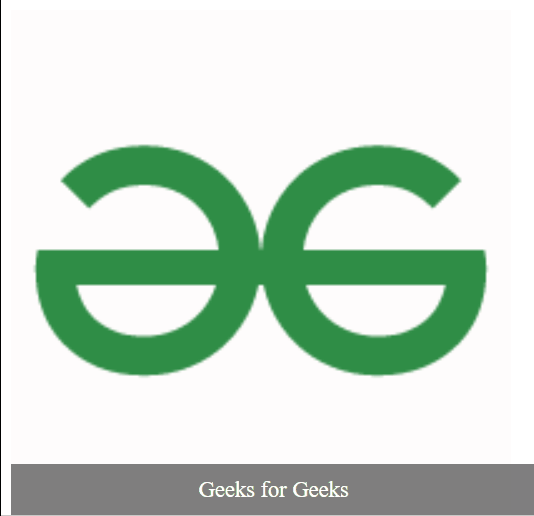
Share your thoughts in the comments
Please Login to comment...