How to load a JSON object from a file with ajax?
Last Updated :
25 Apr, 2024
Loading a JSON object from a file using AJAX involves leveraging XMLHttpRequest (XHR) or Fetch API to request data from a server-side file asynchronously. By specifying the file’s URL and handling the response appropriately, developers can seamlessly integrate JSON data into their web applications. This method enables dynamic loading of data without requiring a page refresh, facilitating responsive and interactive user experiences.
JSON (JavaScript Object Notation) is a simple and lightweight format used to exchange data between servers and different parts of an application. It is easy for both humans and machines to understand and execute. With its simplified and structured approach, JSON makes sending and retrieving data between client and server sides easy.
Approaches
AJAX Approaches:
- Using XMLHttpRequest (XHR): This is a basic AJAX approach.
- Using Fetch API: Fetch API is a modern replacement of XHR but still is an AJAX based approach.
- Using JQuery: provides a simplified AJAX based approach using ‘$’.
- Using Axios: Axios is an another important library for making AJAX requests.
Non-AJAX Approaches:
- Using Node.js: This is mainly for server side javaScript using Node.js that does not involve the communication with client side via HTTP.
example.json File
{
"website": "GeeksForGeeks",
"articles": [
{
"title": "How to access props inside a functional component",
"author": "mathivananshivam",
"published_date": "27-02-2024",
"url": "https://www.geeksforgeeks.org/how-to-access-props-inside-a-functional-component/"
},
{
"title": "Power Spectral Density",
"author": "mathivananshivam",
"published_date": "28-02-2024",
"url": "https://www.geeksforgeeks.org/power-spectral-density/"
},
{
"title": "Why we need Redux in React?",
"author": "mathivananshivam",
"published_date": "14-03-2024",
"url": "https://www.geeksforgeeks.org/why-we-need-redux-in-react/"
}
]
}
Using XMLHttpRequest (XHR)
Using XMLHttpRequest (XHR) involves creating a new instance of XMLHttpRequest, specifying the file URL and request method (typically ‘GET’), and setting up event handlers to handle the response asynchronously. This approach allows for direct control over the AJAX request and is suitable for scenarios where a lightweight solution is preferred or when working without additional libraries.
Example: Implementation to show how to load a JSON object from a file with ajax.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>JSON Fetch</title>
<style>
pre {
background-color: #f4f4f4;
border: 1px solid #ddd;
padding: 10px;
overflow-x: auto;
font-family: monospace;
border-radius: 5px;
}
</style>
</head>
<body>
<h1>JSON Data</h1>
<div id="json-container">
<!-- Parsed JSON data will be displayed here -->
</div>
<script>
let xhr = new XMLHttpRequest();
xhr.open('GET', 'example.json', true);
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
let jsonObject =
JSON.parse(xhr.responseText);
displayJSON(jsonObject);
}
};
xhr.send();
function displayJSON(jsonObject) {
let jsonContainer =
document.getElementById('json-container');
let pre =
document.createElement('pre');
pre.textContent = JSON.stringify(jsonObject, null, 2);
jsonContainer.appendChild(pre);
}
</script>
</body>
</html>
Output:
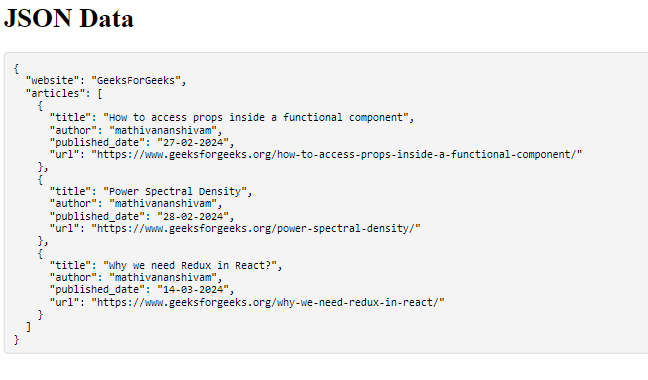
Using JQuery
Using jQuery AJAX involves calling the $.ajax()
function, specifying the URL and data type (e.g., ‘json’), and defining a success callback function to handle the response. This approach abstracts away complexities, providing a simpler syntax and compatibility across different browsers, making it convenient for developers familiar with jQuery.
Example: Implementation to show how to load a JSON object from a file with ajax.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>JSON Fetch Example</title>
<!-- Include jQuery library -->
<script src=
"https://code.jquery.com/jquery-3.6.0.min.js">
</script>
<style>
pre {
background-color: #f4f4f4;
border: 1px solid #ddd;
padding: 10px;
overflow-x: auto;
font-family: monospace;
border-radius: 5px;
}
</style>
</head>
<body>
<h1>JSON Data</h1>
<div id="json-container">
<!-- Parsed JSON data will be displayed here -->
</div>
<script>
$.getJSON('example.json', function (data) {
displayJSON(data);
});
function displayJSON(jsonObject) {
let jsonContainer =
document.getElementById('json-container');
let pre = document.createElement('pre');
pre.textContent = JSON.stringify(jsonObject, null, 2);
jsonContainer.appendChild(pre);
}
</script>
</body>
</html>
Output:
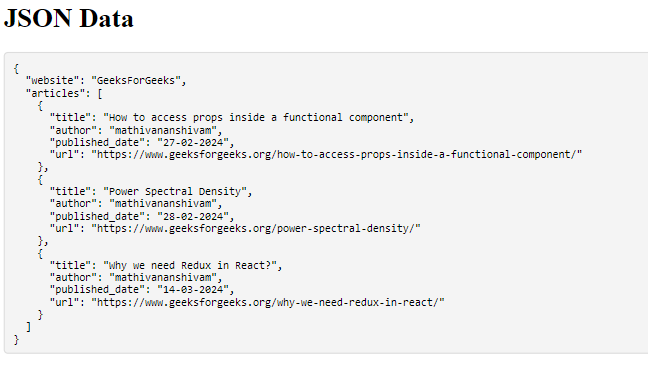
Share your thoughts in the comments
Please Login to comment...