cJSON – JSON File Write/Read/Modify in C
Last Updated :
15 May, 2023
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy to read and write for humans and machines alike. JSON is widely used for data exchange between applications and web services. In this article, we will discuss how to read and write JSON data in the C programming language.
JSON in C
JSON in C can be handled using the cJSON library, which is an open-source library available under the MIT License. It provides a simple and easy-to-use API for parsing, creating, and manipulating JSON data. The cJSON library is written in C and has no external dependencies, making it easy to integrate into C programs.
Writing JSON Data
To write JSON data in C, we need to create a cJSON object and convert it to a JSON string using the cJSON library. Here is an example code snippet to write JSON data to a file:
C
#include <stdio.h>
#include <cjson/cJSON.h>
int main() {
cJSON *json = cJSON_CreateObject();
cJSON_AddStringToObject(json, "name" , "John Doe" );
cJSON_AddNumberToObject(json, "age" , 30);
cJSON_AddStringToObject(json, "email" , "john.doe@example.com" );
char *json_str = cJSON_Print(json);
FILE *fp = fopen ( "data.json" , "w" );
if (fp == NULL) {
printf ( "Error: Unable to open the file.\n" );
return 1;
}
printf ( "%s\n" , json_str);
fputs (json_str, fp);
fclose
cJSON_free(json_str);
cJSON_Delete(json);
return 0;
}
|
Output:
Console Output
{
"name": "John Doe",
"age": 30,
"email": "john.doe@example.com"
}
Generated JSON file
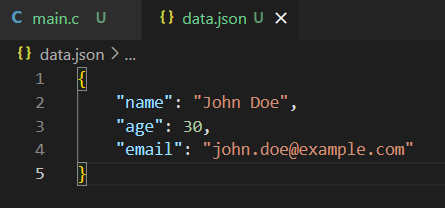
Generated JSON file
Code Explanation:
In this example, we first create a cJSON object using the `cJSON_CreateObject()` function. We then add key-value pairs to the object using the `cJSON_AddStringToObject()` and `cJSON_AddNumberToObject()` functions. We then convert the cJSON object to a JSON string using the `cJSON_Print()` function, which returns a dynamically allocated string that needs to be freed using the `cJSON_free()` function. We then write the JSON string to a file using the `fputs()` function. Finally, we delete the cJSON object using the `cJSON_Delete()` function.
Note 1: In the above program, the output is the JSON data written to a file named “data.json”. The contents of the file are the same as the JSON data printed to the console.
Note 2: The formatting of the JSON data in the output may differ depending on the operating system and text editor used. However, the contents of the JSON data will remain the same.
Reading JSON Data
To read JSON data in C, we need to parse the JSON string using the cJSON library. Here is an example code snippet to read JSON data from a file:
C
#include <stdio.h>
#include <cjson/cJSON.h>
int main() {
FILE *fp = fopen ( "data.json" , "r" );
if (fp == NULL) {
printf ( "Error: Unable to open the file.\n" );
return 1;
}
char buffer[1024];
int len = fread (buffer, 1, sizeof (buffer), fp);
fclose (fp);
cJSON *json = cJSON_Parse(buffer);
if (json == NULL) {
const char *error_ptr = cJSON_GetErrorPtr();
if (error_ptr != NULL) {
printf ( "Error: %s\n" , error_ptr);
}
cJSON_Delete(json);
return 1;
}
cJSON *name = cJSON_GetObjectItemCaseSensitive(json, "name" );
if (cJSON_IsString(name) && (name->valuestring != NULL)) {
printf ( "Name: %s\n" , name->valuestring);
}
cJSON_Delete(json);
return 0;
}
|
Input: (JSON File)
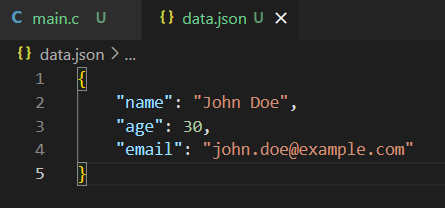
JSON File for input
Output:
Name: John Doe
In this example, we first open the JSON file using the fopen() function and check if it is successful. Then we read the file contents into a buffer using the fread() function. We close the file using the fclose() function. We then parse the JSON data using the cJSON_Parse() function, which returns a pointer to a cJSON object. If the parsing fails, we print the error message using the cJSON_GetErrorPtr() function. We then access the JSON data using the cJSON_GetObjectItemCaseSensitive() function, which returns a pointer to a cJSON object corresponding to the given key. Finally, we delete the cJSON object using the cJSON_Delete() function.
Modifying Existing JSON file Data
Certainly! To modify an existing JSON file, we can use the cJSON library’s functions to read the file into a cJSON object, modify the object, and then write the modified object back to the file. Here’s an example program that demonstrates how to modify a JSON file using the cJSON library in C:
C
#include <stdio.h>
#include <cjson/cJSON.h>
int main() {
FILE *fp = fopen ( "data.json" , "r" );
if (fp == NULL) {
printf ( "Error: Unable to open the file.\n" );
return 1;
}
char buffer[1024];
int len = fread (buffer, 1, sizeof (buffer), fp);
fclose (fp);
cJSON *json = cJSON_Parse(buffer);
if (json == NULL) {
const char *error_ptr = cJSON_GetErrorPtr();
if (error_ptr != NULL) {
printf ( "Error: %s\n" , error_ptr);
}
cJSON_Delete(json);
return 1;
}
cJSON_ReplaceItemInObjectCaseSensitive(json, "name" , cJSON_CreateString( "Jane Doe" ));
cJSON_AddNumberToObject(json, "age" , 32);
cJSON_AddStringToObject(json, "phone" , "555-555-5555" );
char *json_str = cJSON_Print(json);
fp = fopen ( "data.json" , "w" );
if (fp == NULL) {
printf ( "Error: Unable to open the file.\n" );
return 1;
}
printf ( "%s\n" , json_str);
fputs (json_str, fp);
fclose (fp);
cJSON_free(json_str);
cJSON_Delete(json);
return 0;
}
|
In this example program, we first open the JSON file, read its contents into a string, and parse the JSON data using the cJSON_Parse() function. We then modify the JSON data by replacing the value of the “name” key with a new string, adding a new key-value pair for “phone”, and changing the value of the “age” key to 32. We then convert the cJSON object back to a JSON string using the cJSON_Print() function, write the JSON string to the file using the fputs() function, and free the cJSON object and JSON string. After running this program, the “data.json” file should now contain the modified JSON data.
Input: (JSON File)
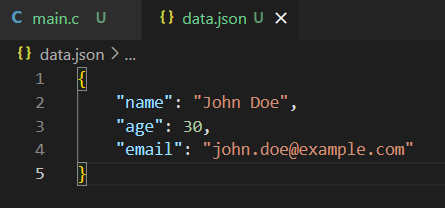
JSON file for input
Output:
Console
{
"name": "Jane Doe",
"age": 32,
"email": "john.doe@example.com",
"phone": "555-555-5555"
}
Modified JSON File
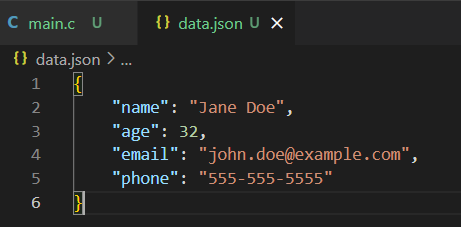
Modified JSON file
Note: In this example, we used the cJSON_ReplaceItemInObjectCaseSensitive() function to replace the value of the “name” key, which is case-sensitive. If the key is not case-sensitive, we can use the cJSON_ReplaceItemInObject() function instead. Also, we can modify the JSON data in any way we want using the cJSON library’s various functions.
Conclusion
In this article, we discussed how to read and write JSON data in C using the cJSON library. The cJSON library provides a simple and easy-to-use API for handling JSON data in C. By using this library, we can easily integrate JSON data into our C programs and exchange data with other applications and web services.
Share your thoughts in the comments
Please Login to comment...