How to Link JavaScript File to a Separate HTML File ?
Last Updated :
21 Mar, 2024
To link the JS file to an HTML document for applying behavior to the UI elements. We can use the <script> tag along with the src attribute. But, we can also link the JS file using the ECMAScript Modules.
Below are the approaches to link a JavaScript file to a separate HTML file:
Using src attribute in script Tag
In this approach, we use the src attribute within the <script> tag in the HTML file to specify the source file (JavaScript) location. The external JavaScript file is then linked to the HTML document, enabling the execution of its code.
Syntax:
<script src="js-file-name"></script>
Example: The below example uses the src attribute in <script> Tag to link the javascript file to a separate HTML file.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Link JavaScript file
to a separate HTML file
</title>
<script src="script.js" defer></script>
</head>
<body>
<h1 style="color: green;">GeeksforGeeks</h1>
<h3>Using src attribute in
<script> Tag
</h3>
<button onclick="h1ChgFn()">
Change Heading
</button>
</body>
</html>
Javascript
// script.js
function h1ChgFn() {
let heading1 = document.querySelector('h1');
heading1.textContent = 'GFG';
}
Output:
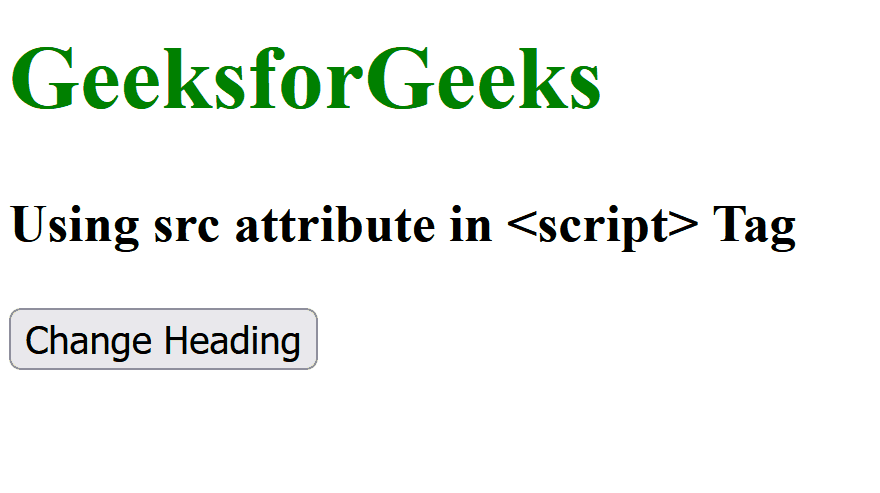
Using ECMAScript Modules
In this approach, we are using ECMAScript Modules to link a JavaScript file to a separate HTML file. The import statement in the HTML file specifies the module’s path. It imports the “initialize” function from the “script.js” file and calls it to initialize the webpage. The JavaScript file defines the “initialize” function, which creates an h1 element with the text “GeeksforGeeks” and an h3 element with the text “Using ECMAScript Modules”. It also creates a button that changes the color of the h1 element when clicked.
Syntax:
import { functionName, variableName } from './file.js';
Example: The below example uses the ECMAScript Modules to link the javascript file to a separate HTML file.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Link JavaScript using ECMAScript Modules</title>
</head>
<body>
<script type="module">
import { initialize } from './script.js';
initialize();
</script>
</body>
</html>
Javascript
// script.js
let headingColor = 'green';
export function initialize() {
document.addEventListener('DOMContentLoaded', function () {
let heading1 = document.createElement('h1');
heading1.textContent = 'GeeksforGeeks';
heading1.style.color = headingColor;
document.body.appendChild(heading1);
let heading3 = document.createElement('h3');
heading3.textContent = 'Using ECMAScript Modules';
document.body.appendChild(heading3);
let button = document.createElement('button');
button.textContent = 'Change Color';
button.addEventListener('click', function () {
changeColor(heading1);
});
document.body.appendChild(button);
});
}
function changeColor(element) {
headingColor = headingColor === 'green' ? 'red' : 'green';
element.style.color = headingColor;
}
Output:
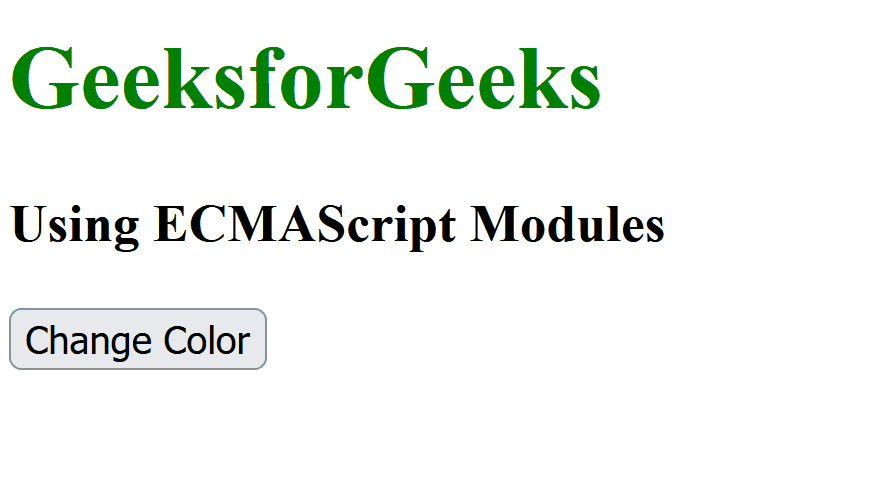
Share your thoughts in the comments
Please Login to comment...