How to Add an ID to Element in JavaScript ?
Last Updated :
18 Mar, 2024
In JavaScript, the ID is the unique identifier through which the elements can be identified and manipulated if required. We can add an ID to an element in JavaScript using various approaches that are explored in this article with practical demonstration.
Below are the possible approaches:
Using setAttribute()
In this approach, we are using JavaScript’s setAttribute() method to dynamically add an ID to an HTML element. Select the element using getElementsByTagName() and then set its ID attribute with the value obtained from user input.
Syntax:
Object.setAttribute(attributename,value)
Example: The below example uses setAttribute() to add an ID to an element in JavaScript.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<style>
body {
text-align: center;
}
input {
padding: 10px;
margin: 10px;
}
</style>
</head>
<body>
<h3>
Using setAttribute()
</h3>
<input type="text" placeholder="Enter ID">
<button onclick="addFn()">
Add ID
</button>
<script>
function addFn() {
const inputEle =
document.getElementsByTagName('input');
const temp = inputEle[0];
const val = temp.value;
temp.setAttribute('id', val);
alert('ID Added successfully!');
}
</script>
</body>
</html>
Output:
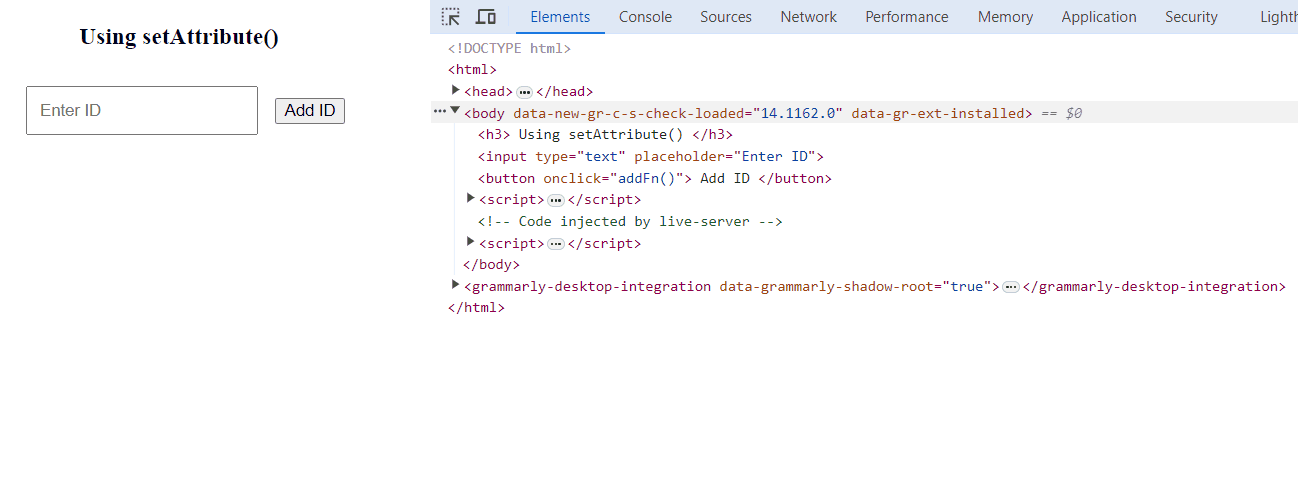
Using Direct assignment to id property
In this approach, we are using JavaScript’s direct assignment to the element’s id property to dynamically add an ID. By selecting the element with document.querySelector() and assigning a value to its id property.
Syntax:
Selected_element.id = newID;
Example: The below example uses Direct assignment to id property to add an ID to an element in JavaScript.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 2</title>
<style>
body {
text-align: center;
}
h1 {
color: green;
}
</style>
</head>
<body>
<h3>
Using Direct assignment to
id property
</h3>
<button onclick="addId()">
Add ID to h3
</button>
<script>
function addId() {
const h3Element =
document.querySelector('h3');
h3Element.id = 'h3ID';
alert('ID added to h3: ' + h1Element.id);
}
</script>
</body>
</html>
Output:
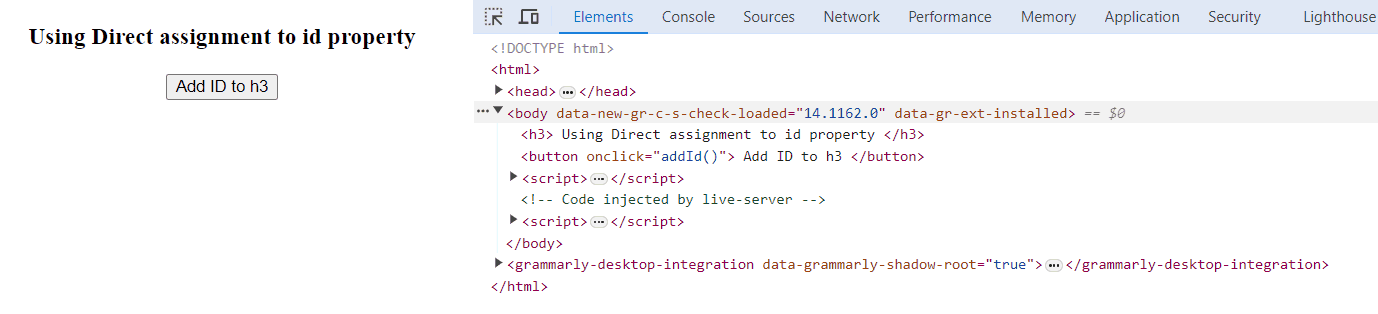
Share your thoughts in the comments
Please Login to comment...