How to include a template with parameters in EJS?
Last Updated :
27 Feb, 2024
In EJS, we can include templates with parameters for the reuse of modular components in web applications. By passing parameters during the inclusion, we can create interactive templates. In this article, we will see a practical example of including a template with parameters in EJS. Below is the syntax to include a template with parameters in EJS.
Syntax to include template with parameters:
<%- include('template.ejs', {param1: value1, param2: value2, ...}) %>
Steps to include a template with parameters in EJS:
Step 1: Firstly, we will make the folder named root by using the below command in the VScode Terminal. After creation use the cd command to navigate to the newly created folder.
mkdir root
cd root
Step 2: Once the folder is been created, we will initialize NPM using the below command, this will give us the package.json file.
npm init -y
Step 3: Once the project is been initialized, we need to install Express and EJS dependencies in our project by using the below installation command of NPM.
npm i express ejs
Step 4: Now create the below Project Structure in your project which includes app.js, views/index.ejs, and views/footer.ejs files.
Folder Structure:
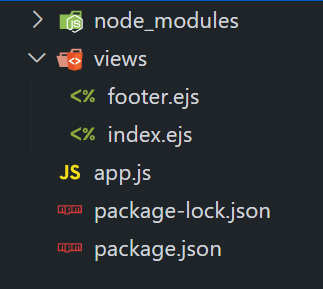
The updated dependencies in package.json will look like:
"dependencies": {
"express": "^4.18.2",
"ejs": "^3.1.9",
}
Approach to include template with parameters in EJS:
- In the above example, we are passing the information to the EJS template using res.render(‘index’, { data: temp}).
- We have included a footer template with the dynamic parameters in the main template using <%- include(‘footer.ejs’, { year: new Date().getFullYear() }) %>.
Example: Now, we need to write the code for the app.js file, code for views/index.ejs to include a template with parameters and code for views/footer.ejs which defines as a template and passes the year to the main template.
Javascript
const express = require( 'express' );
const app = express();
const port = 3000;
app.set( 'view engine' , 'ejs' );
const temp = {
title: 'GeeksforGeeks' ,
description: 'A computer science portal for geeks.' ,
topics: [ 'Algorithms' , 'Data Structures' , 'Web Development' ],
};
app.get( '/' , (req, res) => {
res.render( 'index' , { data: temp });
});
app.listen(port, () => {
console.log(
`Server is running at
http:
);
});
|
HTML
<!DOCTYPE html>
< head >
< title >
<%= data.title %>
</ title >
</ head >
< body >
< h1 style = "color: green;" >
<%= data.title %>
</ h1 >
< p >
<%= data.description %>
</ p >
< h2 >Popular Topics:</ h2 >
< ul >
<% data.topics.forEach(topic=> { %>
< li >
<%= topic %>
</ li >
<% }); %>
</ ul >
<%- include('footer.ejs', { year: new Date().getFullYear() }) %>
</ body >
</ html >
|
HTML
< footer >
© <%= year %> GeeksforGeeks
</ footer >
|
To run the application, we need to start the server by using the below command.
node app.js
Output:
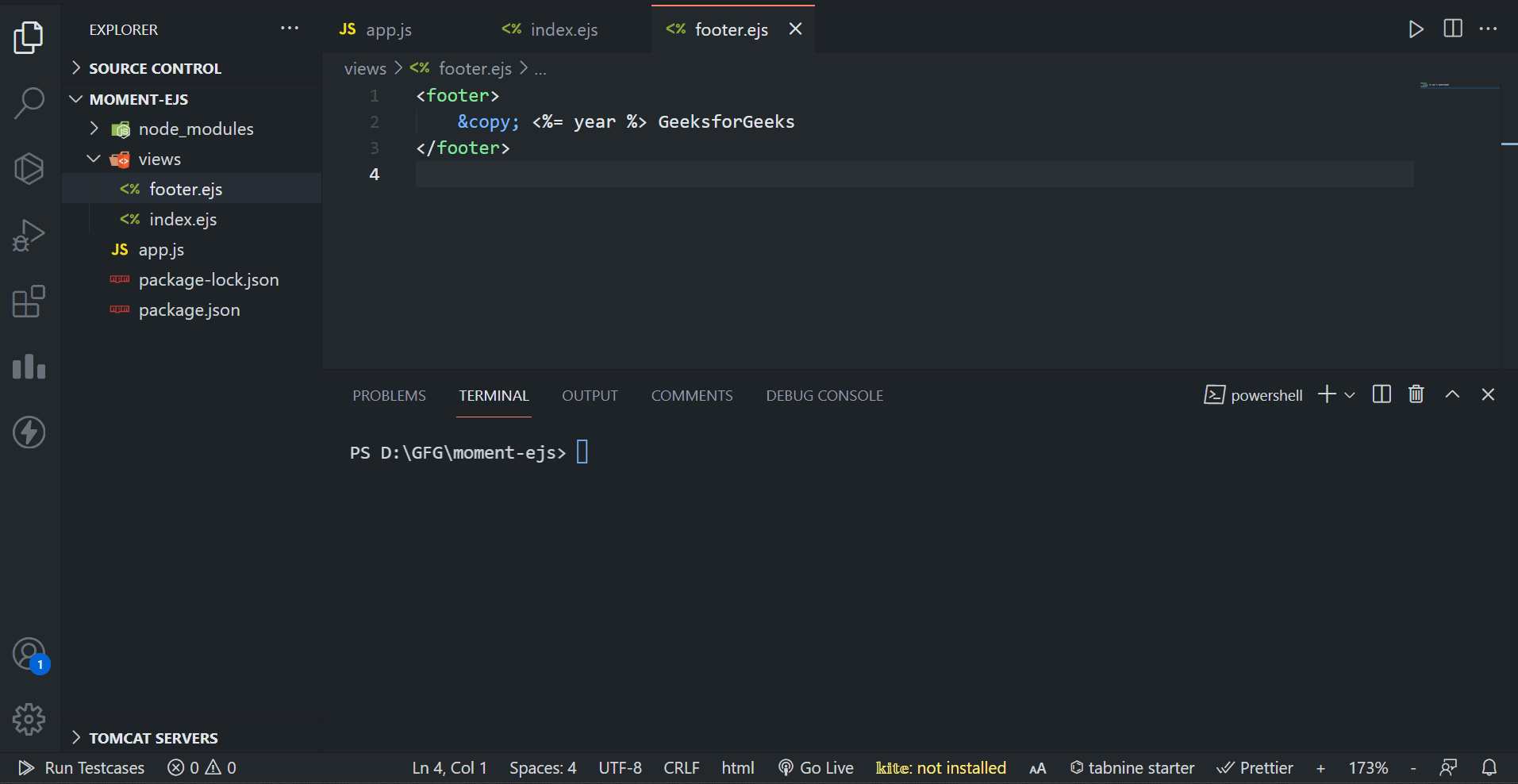
Share your thoughts in the comments
Please Login to comment...