How to import a CSS file in React ?
Last Updated :
15 Mar, 2024
In React applications, styling is a crucial aspect of creating visually appealing and responsive user interfaces. CSS (Cascading Style Sheets) files are commonly used to define styles for React components. Importing CSS files into React components allows developers to apply styles to specific elements and create a cohesive design for their applications. This guide provides a detailed description of how to import CSS files in React components, including best practices and optional configurations such as CSS Modules.
Steps to Import a CSS file in a React:
Step 1: Create a new ReactJS project and install the required dependencies:-
npx create-react-app css-app
Step 2: Navigate to the root directory of your project using the following command.
cd css-app
Project Structure:
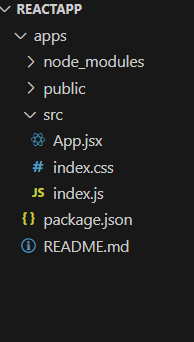
Importing an External CSS File:
In this code i have set the background color of page to aqua, and all the data in the h1 tag will have a border radius of 20px , background color to pastel pink and text should be aligned to center with margin from top 100px.
Code: Write the following code in index.css file
Javascript
/* index.css */
* {
margin: 0;
}
body {
background-color: aqua;
}
h1 {
border-radius: 20px;
background-color: rgb(251, 141, 196);
text-align: center;
margin-top: 100px;
}
In index.js file write the below code:
Note : Import the index.css file using import ‘./index.css’;
Importing CSS in ReactJs:
Javascript
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
ReactDOM.render(
<>
<h1> hello Geek!!</h1>
</>
, document.getElementById("root")
);
Using Inline CSS in React:
Note : In index.js file write this code this will apply the cs using the inline css:
In this we don’t need to create a seprate index.css file. We have applied css to the h1 tag withing the body part using <style>.
Javascript
import React from 'react';
import ReactDOM from 'react-dom';
ReactDOM.render(
<>
<head>
<style>// importing css
{`
body {
margin: 0;
background-color: aqua;
}
`}
</style>
</head>
<body>
<h1 style={{
borderRadius: '20px',
backgroundColor: 'rgb(251, 141, 196)',
textAlign: 'center',
marginTop: '100px'
}}>hello Geek!!</h1>
</body>
</>,
document.getElementById("root")
);
Using Internal CSS in React :
Note : In index.js file write this code this will apply the cs using the internal css:
In this code we have used the internal css where all the css is defined in the <head> tag using the <style>, below is the code snippet:
Javascript
import React from 'react';
import ReactDOM from 'react-dom';
ReactDOM.render(
<>
<head>
<style>
{`
* {
margin: 0;
}
body {
background-color: aqua;
}
h1 {
border-radius: 20px;
background-color: rgb(251, 141, 196);
text-align: center;
margin-top: 100px;
}
`}
</style>
</head>
<body>
<h1>hello Geek!!</h1>
</body>
</>,
document.getElementById("root")
);
Start your application using the following command.
npm start
Output:
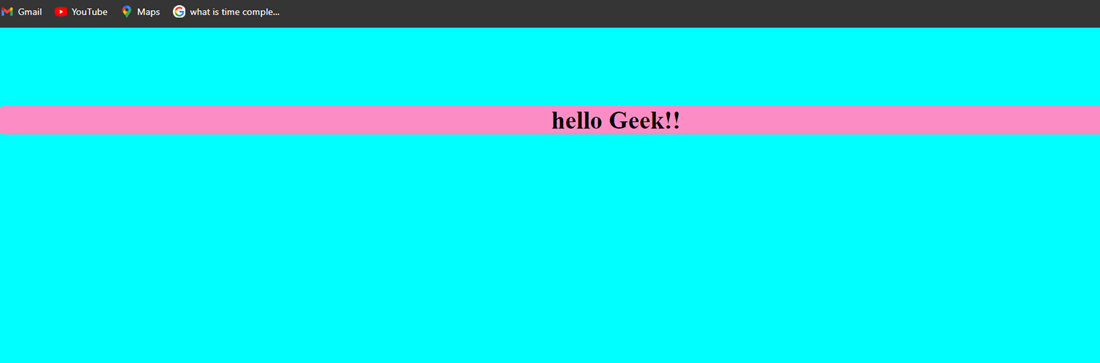
Share your thoughts in the comments
Please Login to comment...