How to Hide Value or Text From Input Control in HTML ?
Last Updated :
14 Feb, 2024
Hiding a value or text from an input control is a common requirement in web development. Whether for security purposes, such as password fields, or to maintain a clean user interface, HTML and CSS offer several methods to achieve this. This article explores various approaches to hide text or values within input controls, providing detailed descriptions and complete HTML code examples.
Approach 1: Using the type=”password” Attribute
The basic method to hide text in an input control is by using the type=”password” attribute. This approach is particularly suited for forms requiring users to enter sensitive information like passwords.
Example:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Hide Text in Input Control</ title >
</ head >
< body >
< form >
< label for = "password" >Password:</ label >
< input type = "password" id = "password" name = "password" >
</ form >
</ body >
</ html >
|
Output
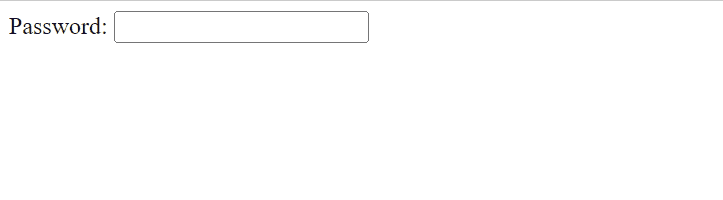
Approach 2: Using CSS to Temporarily Hide Text
For scenarios where you might want to hide input text temporarily (for example, hiding API keys or temporarily sensitive information that might become visible under certain conditions), you can use CSS. This method involves changing the text color to match the input’s background.
Example:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Temporarily Hide Input Text</ title >
< style >
.hidden-text {
color: transparent;
}
</ style >
</ head >
< body >
< form >
< label for = "api-key" >API Key:</ label >
< input type = "text" id = "api-key"
name = "api-key" class = "hidden-text" >
</ form >
</ body >
</ html >
|
Output
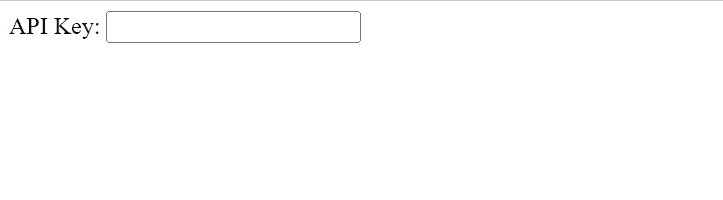
This CSS trick makes the text color transparent, effectively hiding it from view but still present in the DOM. This method is not secure for sensitive information, as the value can easily be seen by inspecting the element or disabling CSS.
Approach 3: Dynamically Hiding Text with JavaScript
JavaScript can dynamically toggle the visibility of input values, offering more flexibility, such as hiding or showing text based on user interactions.
Example:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Dynamically Hide Input Text</ title >
</ head >
< body >
< form >
< label for = "user-input" >User Input:</ label >
< input type = "text" id = "user-input" name = "user-input" >
< button type = "button" onclick = "toggleVisibility()" >
Toggle Visibility
</ button >
</ form >
< script >
function toggleVisibility() {
const input = document.getElementById('user-input');
if (input.type === 'text') {
input.type = 'password';
} else {
input.type = 'text';
}
}
</ script >
</ body >
</ html >
|
Output
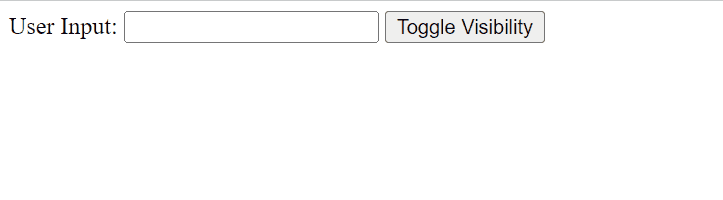
In this example, a button toggles the input type between text and password, thereby controlling the visibility of the input’s value. This approach is versatile, allowing text to be hidden or shown based on user actions.
Share your thoughts in the comments
Please Login to comment...