How to Hide a Value or Text from the HTML Input control ?
Last Updated :
02 Apr, 2024
Hiding values or text from HTML input controls involves concealing information within the web page’s structure so that it’s not immediately visible to the user but can still be accessed and processed by the web application.
This technique is often used for storing sensitive data or for managing information that doesn’t need to be directly displayed to the user.
Using HTML <input type=”hidden”>
In this approach, we will use the HTML <input type=”hidden”> property to hide a value or text from the HTML Input control. The type=”hidden” attribute specifies that the input field should be hidden from the user interface. While hidden, the input still retains its value but is not visible to the user.
Example: Hiding a value or text from the HTML Input control using Using HTML <input type=”hidden”> Property
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Hidden Input Value</title>
<style>
.button {
background-color: #48874a;
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
margin-top: 15px;
margin-right: 15px;
}
</style>
</head>
<body>
<h6>Hiding a Value or Text from the HTML Input Control
using <input type="hidden"> Property
</h6>
<input type="hidden" id="hiddenInput"
value="Hidden Value">
<br>
<button class="button" onclick="revealHiddenInput()">
Show Hidden Input Value
</button>
<script>
function revealHiddenInput() {
let hiddenInput =
document.getElementById("hiddenInput").value;
alert("Hidden Input Value: " + hiddenInput);
}
</script>
</body>
</html>
Output:
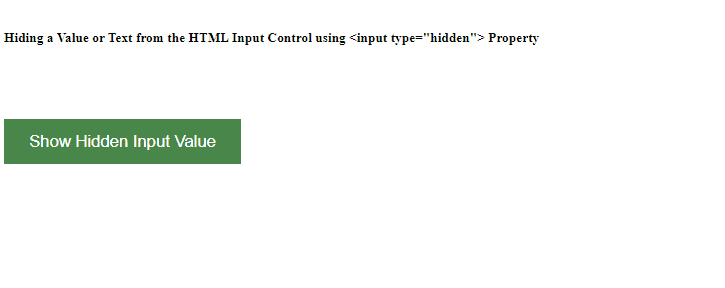
Output
Using CSS color: transparent Property
In this approach, we will use the CSS color: transparent property to hide a value or text from the HTML input control. The color property in CSS controls the color of the text content of an element. Setting color: transparent makes the text completely transparent, effectively hiding it from view while still preserving its space in the layout.
Example: Hiding a value or text from the HTML Input control using CSS color: transparent Property
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Hidden Input Value</title>
<style>
.button {
background-color: #789335;
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
margin-top: 15px;
margin-right: 15px;
}
input[type="text"] {
margin-top: 10px;
}
</style>
</head>
<body>
<h6>Hiding a Value or Text from the HTML
Input Control using transparent
</h6>
<input type="text">
<input type="text" style="color: transparent;" value="">
<br>
</body>
</html>
Output:
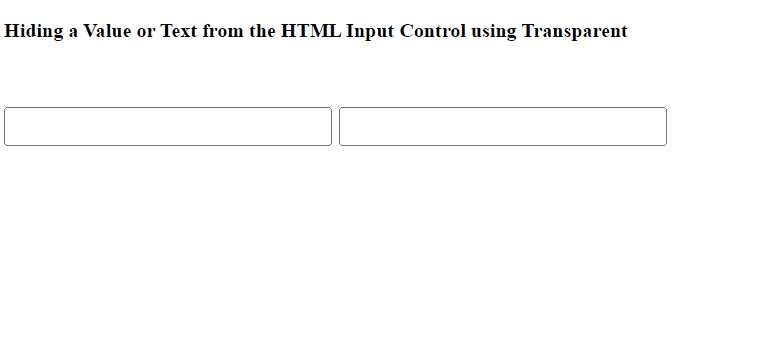
Output
Using CSS display: none Property
In this approach, we will use the CSS display: none
property to hide a value or text from the HTML input control. The display
property in CSS controls how an element is rendered in the layout.
Example: Hiding a value or text from the HTML Input control using CSS display: none Property
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Hidden Input Value</title>
<style>
.button {
background-color: #3c7e71;
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
margin-top: 15px;
margin-right: 15px;
}
input[type="text"] {
margin-top: 10px;
}
</style>
</head>
<body>
<h6>
Hiding a Value or Text from the HTML Input
Control using display: none Property
</h6>
<input type="text">
<input type="text" id="input1"
style="display: none;"
value="Hidden Value (Visibility)">
<br>
<button class="button" onclick="revealHiddenInputs()">
Show Hidden Inputs
</button>
<script>
function revealHiddenInputs() {
document.getElementById("input1")
.style.display = "block";
}
</script>
</body>
</html>
Output:
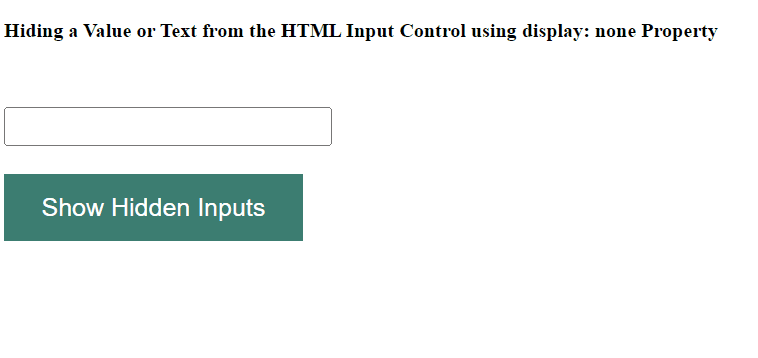
Output
Using CSS visibility: hidden Property
In this approach, we will use CSS visibility: hidden Property to hide a value or text from the HTML Input control. The visibility property in CSS controls whether an element is visible or hidden while still occupying space in the layout. Set the visibility property to hidden to hide an element while preserving its space in the document flow.
Example: Hiding a value or text from the HTML Input control using CSS visibility: hidden Property
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Hidden Input Value</title>
<style>
.button {
background-color: #48874a;
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
margin-top: 15px;
margin-right: 15px;
}
input[type="text"] {
margin-top: 10px;
}
</style>
</head>
<body>
<h6>Hiding a Value or Text from the HTML Input
Control using visibility: hidden Property
</h6>
<input type="text">
<input type="text" id="input2"
style="visibility: hidden;"
value="Hidden Value (Visibility)">
<br>
<button class="button"
onclick="revealHiddenInputs()">
Show Hidden Inputs
</button>
<script>
function revealHiddenInputs() {
document.getElementById("input2")
.style.visibility = "visible";
}
</script>
</body>
</html>
Output:
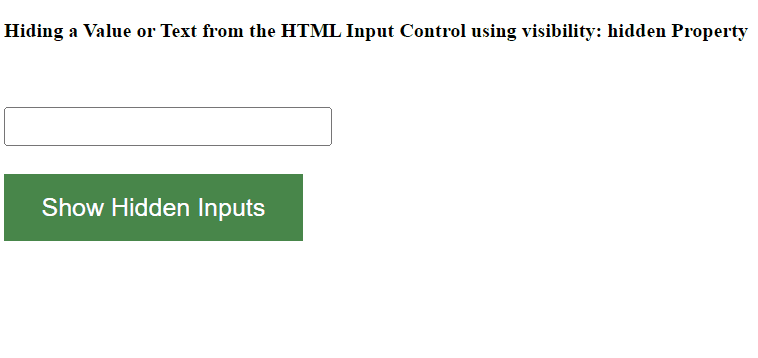
Output
Using CSS Opacity Property
In this approach, we will use CSS Opacity Property to hide a value or text from the HTML Input control. Opacity refers to the transparency level of an element. Setting an element’s opacity to 0 makes it completely transparent, effectively hiding it from view.
Example: Hiding a value or text from the HTML Input control using CSS Opacity Property
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Hidden Input Value</title>
<style>
.button {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
margin-top: 15px;
margin-right: 15px;
}
input[type="text"] {
margin-top: 10px;
}
</style>
</head>
<body>
<h6>Hiding a value or text from the HTML
Input control using CSS Opacity Property
</h6>
<!-- Opacity Method -->
<input type="text">
<input type="text" id="input3" style="opacity: 0;"
value="Hidden Value (Opacity)">
<br>
<button class="button" onclick="revealHiddenInputs()">
Show Hidden Inputs
</button>
<script>
function revealHiddenInputs() {
document.getElementById("input3")
.style.opacity = 1;
}
</script>
</body>
</html>
Output:
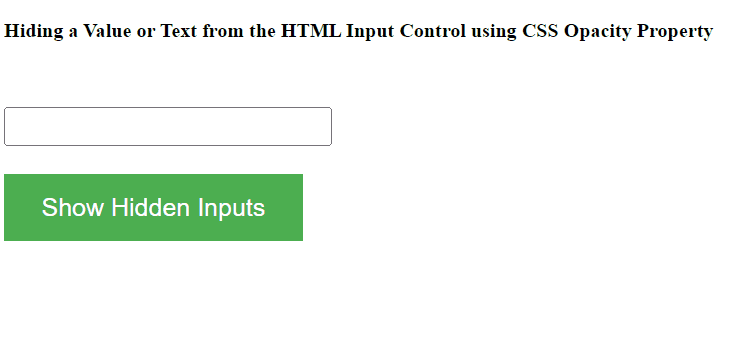
Output
Share your thoughts in the comments
Please Login to comment...