How to get the domain originating the request in Express?
Last Updated :
25 Dec, 2023
In Express, there is a feature to get or retrieve the domain originating the request. The request client’s original domain can be received by checking the HTTP headers or properties that are lined with the incoming request. Along with this, there are some built-in approaches and methods through which we can get the domain originating the request in Express.
We are going to get the domain originating the request in Express by using the two scenarios/approaches below.
Prerequisites
Steps to create Express application
Step 1: In the first step, we will create the new folder by using the below command in the VScode terminal.
mkdir folder-name
cd folder-name
Step 2: After creating the folder, initialize the NPM using the below command. Using this the package.json file will be created.
npm init -y
Step 3: Now, we will install the express dependency for our project using the below command.
npm i express
Step 4: Now create the below Project Structure of our project which includes the file as app.js.
Project Structure:
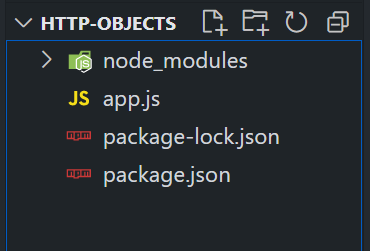
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2"
}
Approach 1: Using host Property
- We are going to use the host property which is the request object that can be used to access the hostname which is specified in the HTTP request.
- Using this method, we can directly receive the host information without relying on any other specific header.
Syntax:
const originDomain = req.get('host');
Example: Below is the implementation of the above-discussed approach.
Javascript
const express = require( 'express' );
const app = express();
app.use((req, res, next) => {
const originDomain = req.get( 'host' );
console.log( 'Origin Domain:' , originDomain);
next();
});
app.get( '/' , (req, res) => {
res.send( 'Hello, GeeksforGeeks. Domain is in Terminal' );
});
app.listen(3000, () => {
console.log( 'Server is running on port 3000' );
});
|
Output:
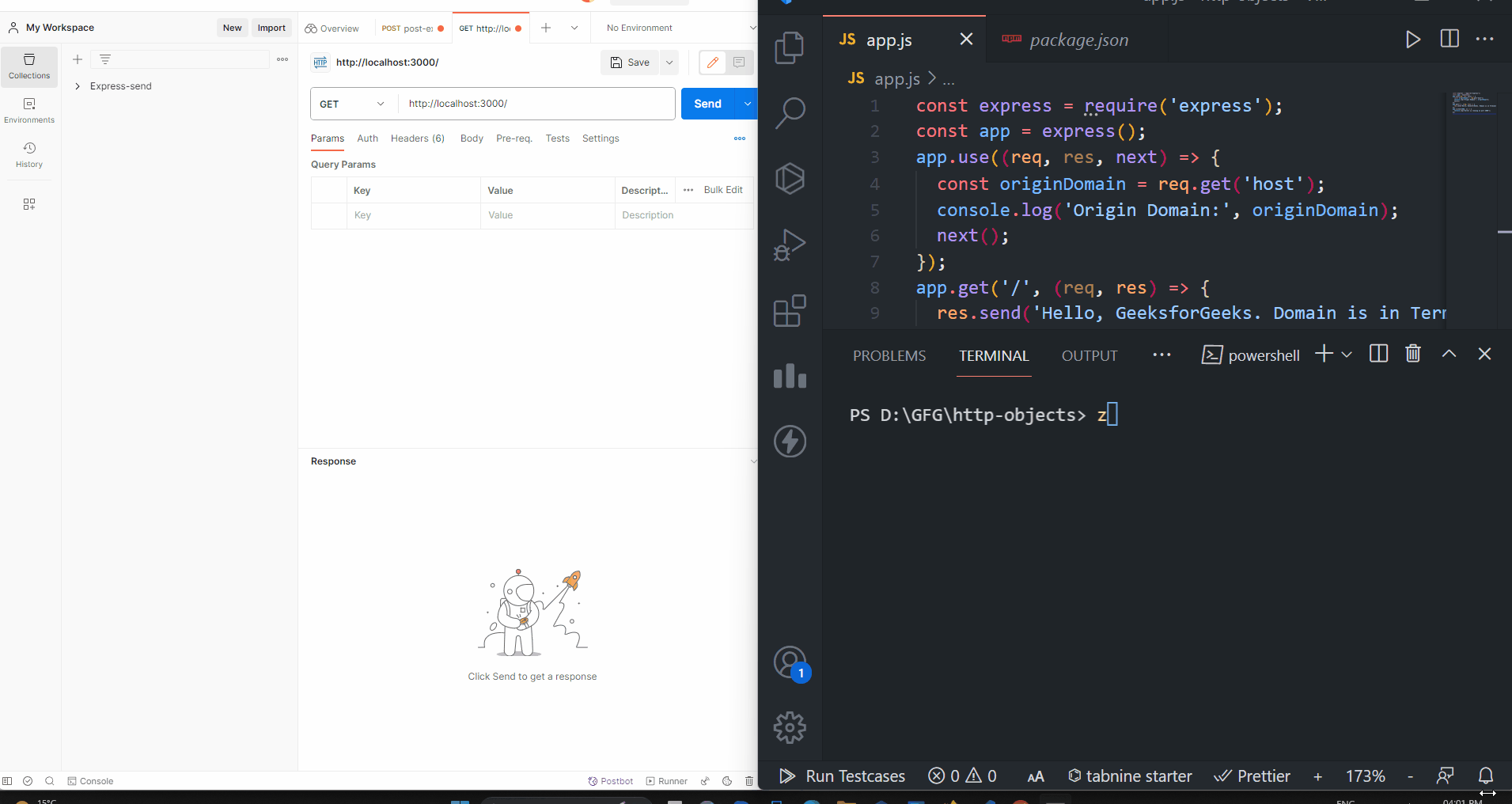
Approach 2: Using hostname
- We are using the hostname property that provides the hostname of the request’s URL. This represents the host(domain) portion of the URL which excludes the port and other subdomains.
- As compared to the above approach, the host property gives the port and subdomains also.
Syntax:
const domain = req.hostname;
Example: Below is the implementation of the above-discussed approach.
Javascript
const express = require( 'express' );
const app = express();
app.get( '/' , (req, res) => {
const domain = req.hostname;
res.send(`Domain: ${domain}`);
});
const port = 3000;
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
|
Output:
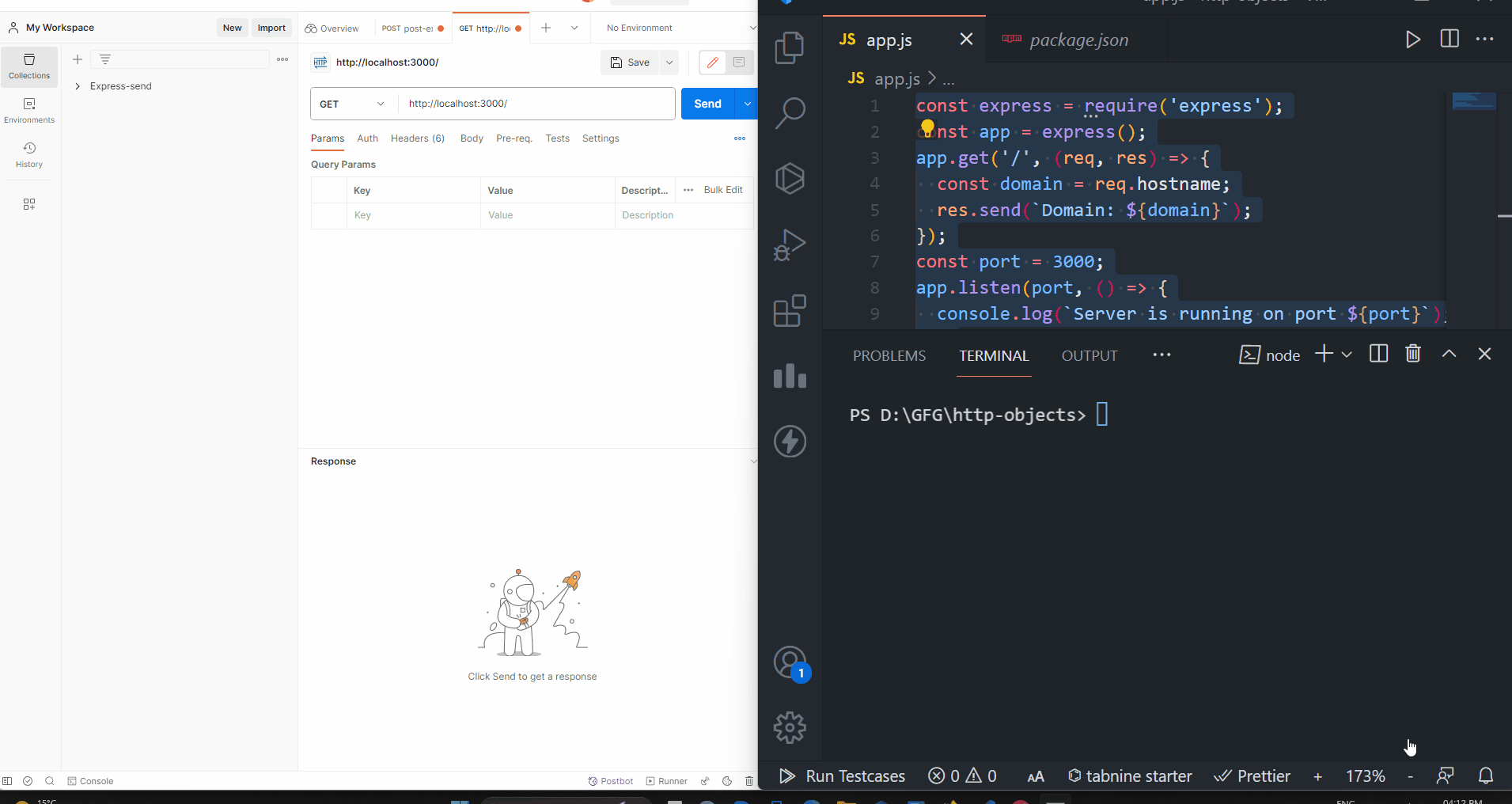
Share your thoughts in the comments
Please Login to comment...