How to get selected text from a drop-down list using jQuery?
Last Updated :
14 Dec, 2023
In this article, we will learn how we can get the text of the selected option in a select tag using jQuery. There are two ways in jQuery that we can use to accomplish this task.
Using the val() method
The val() method is an inbuilt method in jQuery that is used to return or set the value of the value attributes of the selected elements.
Syntax:
$(selector).val(parameter)
Parameter: It takes the optional parameter as the value you want to set to the value attribute of the selected element.
Example: The below example will explain the use of the val() method to get the value of the selected option.
html
< html >
< head >
< title >jQuery Selector</ title >
< script src =
</ script >
< script >
$(document).ready(function() {
$("#submit").click(function() {
const selectedVal = $("#myselection").val();
$('#result')
.text(`The selected value is: ${selectedVal}`);
});
});
</ script >
< style >
div {
width: 50%;
height: 200px;
padding: 10px;
border: 2px solid green;
}
</ style >
</ head >
< body >
< center >
< div >
< p >The selected value:</ p >
< select id = "myselection" >
< option value = "1" >One</ option >
< option value = "2" >Two</ option >
< option value = "3" >Three</ option >
< option value = "4" >Four</ option >
< option value = "5" >Five</ option >
</ select >
< br >
< br >
< button id = "submit" >Submit</ button >
< p id = "result" ></ p >
</ div >
</ center >
</ body >
</ html >
|
Output:
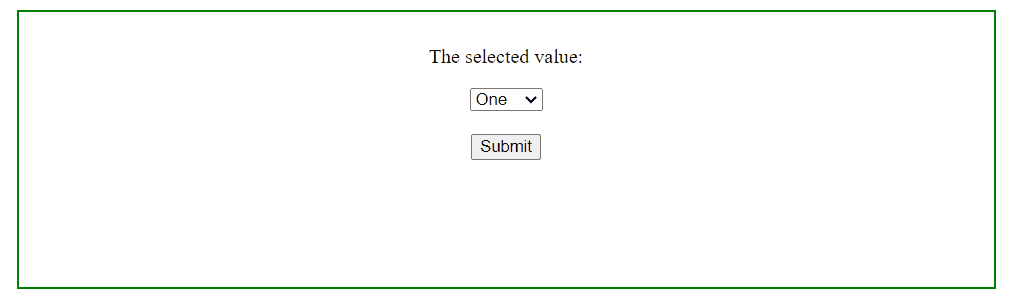
By using option:selected selector and text() method togehter
The option:selected selector is a way in jQuery which is used to return the selected element from a list of the element. Once we get the selected list item, we can use the text() method to get the text of that selected element.
Syntax:
$("#selector option:selected").text();
Example: The below code example illustrate the use of the option:selected selector and text() method to get the value of the selected item.
html
< html >
< head >
< title >jQuery Selector</ title >
< script src =
</ script >
< script >
$(document).ready(function() {
$("#submit").click(function() {
const selectedVal =
$("#myselection option:selected").text();
$('#result')
.text(`The selected value is: ${selectedVal}`);
});
});
</ script >
< style >
div {
width: 50%;
height: 200px;
padding: 10px;
border: 2px solid green;
}
</ style >
</ head >
< body >
< center >
< div >
< p >The selected value:</ p >
< select id = "myselection" >
< option value = "1" >One</ option >
< option value = "2" >Two</ option >
< option value = "3" >Three</ option >
< option value = "4" >Four</ option >
< option value = "5" >Five</ option >
</ select >
< br >
< br >
< button id = "submit" >Submit</ button >
< p id = "result" ></ p >
</ div >
</ center >
</ body >
</ html >
|
Output:
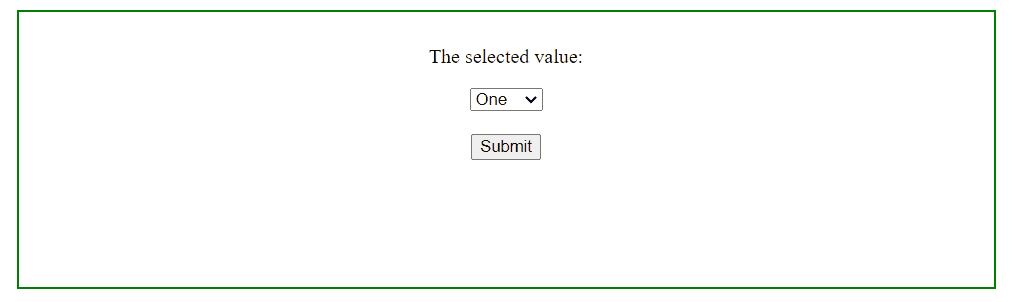
jQuery is an open source JavaScript library that simplifies the interactions between an HTML/CSS document, It is widely famous with it’s philosophy of “Write less, do more”. You can learn jQuery from the ground up by following this jQuery Tutorial and jQuery Examples.
Share your thoughts in the comments
Please Login to comment...