How to Generate Video from Images in HTML5 ?
Last Updated :
01 Apr, 2024
Generating a video from images in HTML5 involves utilizing HTML, CSS, and JavaScript to dynamically create a video by stitching together a sequence of images. Additionally, the transition effects and frame rate control ensure a seamless and optimized viewing experience for the generated video.
Approach
- The HTML file contains a basic structure with a navigation bar (navbar) and an input element for selecting images. It also has a button for generating the video and elements for displaying the video and canvas.
- JavaScript filters and loads only images onto the page.
- A “Generate Video” button initiates video creation via the MediaRecorder API.
- Smooth transitions between images are achieved with cross-fade effects.
- The resulting video, with a regulated frame rate and optimized playback quality, is displayed using an HTML <video> element.
Example: Implementation of generating video from images in HTML5.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width,
initial-scale=1.0">
<title>Image to Video with Transition</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
.navbar {
background-color: #333;
padding: 10px 0;
text-align: center;
}
.logo {
width: 80px;
height: auto;
}
#canvas {
display: none;
}
input[type="file"],
button {
margin: 10px;
padding: 8px 16px;
font-size: 16px;
cursor: pointer;
border: none;
background-color: #007bff;
color: #fff;
border-radius: 5px;
}
input[type="file"] {
display: inline-block;
}
#generateBtn {
display: inline-block;
}
#videoPlayer {
display: none;
width: 100%;
max-width: 640px;
height: auto;
}
</style>
</head>
<body>
<nav class="navbar">
<div class="logo-container">
<img alt="Logo"
class="logo"
src=
"https://media.geeksforgeeks.org/gfg-gg-logo.svg">
</div>
</nav>
<input type="file" id="imageInput"
accept="image/*" multiple>
<button id="generateBtn">Generate Video</button>
<canvas id="canvas"></canvas>
<video controls id="videoPlayer"></video>
<script>
const imageInput = document
.getElementById('imageInput');
const generateBtn = document
.getElementById('generateBtn');
const canvas = document
.getElementById('canvas');
const ctx = canvas.getContext('2d');
const videoPlayer = document
.getElementById('videoPlayer');
let images = [];
let currentImageIndex = 0;
// Load selected images
imageInput.addEventListener('change',
function () {
images = [];
const files = this.files;
for (let i = 0; i < files.length; i++) {
const file = files[i];
if (file.type.match('image.*')) {
const img = new Image();
img.src = URL.createObjectURL(file);
images.push(img);
}
}
});
// Generate video from images with transition
generateBtn.addEventListener('click', function () {
if (images.length === 0) {
alert('Please select some images first.');
return;
}
const frameRate = 10; // Frames per second
const recorder = new MediaRecorder(canvas.
captureStream(frameRate),
{ mimeType: 'video/webm' });
const chunks = [];
recorder.ondataavailable = function (e) {
if (e.data.size > 0) {
chunks.push(e.data);
}
};
recorder.onstop = function () {
const blob = new Blob(chunks,
{ type: 'video/webm' });
const url = URL.createObjectURL(blob);
videoPlayer.src = url;
videoPlayer.style.display = 'block';
};
recorder.start();
const drawFrame = () => {
if (currentImageIndex >= images.length) {
recorder.stop();
return;
}
const fadeInDuration = 1000;
const fadeOutDuration = 500;
const totalDuration = fadeInDuration +
fadeOutDuration;
const fadeIn = () => {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.globalAlpha = Math.min(1,
(Date.now() - startTime)
/ fadeInDuration);
ctx.drawImage(images[currentImageIndex], 0, 0,
canvas.width, canvas.height);
if (Date.now() - startTime < fadeInDuration) {
requestAnimationFrame(fadeIn);
} else {
setTimeout(fadeOut, totalDuration);
}
};
const fadeOut = () => {
ctx.clearRect(0, 0, canvas.width,
canvas.height);
ctx.globalAlpha = Math.max(0, 1 -
(Date.now() - startTime -
fadeInDuration) /
fadeOutDuration);
ctx.drawImage(images[currentImageIndex], 0,
0, canvas.width, canvas.height);
if (Date.now() - startTime < totalDuration) {
requestAnimationFrame(fadeOut);
} else {
currentImageIndex++;
setTimeout(drawFrame, 0);
}
};
const startTime = Date.now();
fadeIn();
};
currentImageIndex = 0;
drawFrame();
});
</script>
</body>
</html>
Output:
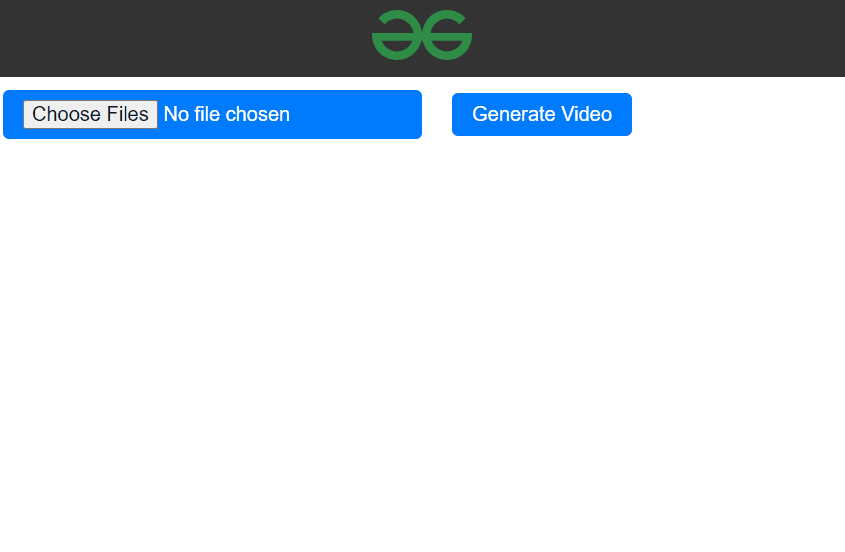
Output
Share your thoughts in the comments
Please Login to comment...