How to generate document with Node.js or Express.js REST API?
Last Updated :
03 Jan, 2024
Generating documents with Node and Express REST API is an important feature in the application development which is helpful in many use cases.
In this article, we will discuss two approaches to generating documents with Node.js or Express.js REST API.
Prerequisites:
Setting up the Project and install dependencies.
Step 1: Create a directory for the project and move to that folder.
mkdir document-generator-app
cd document-generator-app
Step 2: Create a node.js application
npm init -y
Step 3: Install the required dependencies.
npm install express
Project Structure:
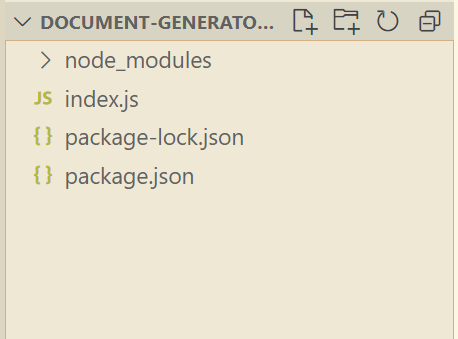
Project Structure
Approach 1: Document Generation using PdfKit library
To start building documents using PdfKit library, we first need to install PdfKit package.
npm install pdfkit
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"pdfkit": "^0.14.0"
}
Example: Now, write the following code into the index.js file.
Javascript
const express = require( 'express' );
const PDFDocument = require( 'pdfkit' );
const app = express();
const port = 3000;
app.use(express.json());
app.post( '/generate-pdf' , (req, res) => {
const { content } = req.body;
const stream = res.writeHead(200, {
'content-type' : 'application/pdf' ,
'content-disposition' : 'attachment;filename=doc.pdf'
});
buildPdf(
(chunk) => stream.write(chunk),
() => stream.end()
);
function buildPdf(dataCallback, endCallback) {
const doc = new PDFDocument();
doc.on( 'data' , dataCallback);
doc.on( 'end' , endCallback);
doc.fontSize(25).text(content);
doc.end();
}
});
app.listen(port, () => {
console.log(`Server is running on http:
});
|
Step to run the application:
Step 1: Run the following command in the terminal to start the server.
node index.js
Step 2: Open the Postman app and make a post request with the following route and add the content in the body which you want to print on the pdf.
http://localhost:3000/generate-pdf
{
"content": "This is my first PDF generated with PdfKit"
}
Output:
.jpg)
Approach 2: Document Generation using Puppeteer library:
To have a build-in function of generating pdf from HTML, we can make the use of puppeteer library over the express server. To have the things setup, we first make sure that we have the puppeteer library installed.
you can install puppeteer by opening the terminal and commanding:
npm install puppeteer
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"puppeteer": "^21.6.1"
}
With puppeteer being installed in the system. Paste the following code in index.js file.
Javascript
const express = require( 'express' );
const puppeteer = require( 'puppeteer' )
const app = express();
const port = 3000;
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
app.post( '/generate-document' , async (req, res) => {
try {
const browser = await puppeteer.launch();
const page = await browser.newPage();
const htmlContent =
`<html>
<body>
<h1>${req.body.title}</h1>
<p>${req.body.content}</p>
</body>
</html>`;
await page.setContent(htmlContent, { waitUntil: 'domcontentloaded' });
const pdfBuffer = await page.pdf();
await browser.close();
res.contentType( 'application/pdf' );
res.send(pdfBuffer);
} catch (error) {
console.error( 'Error generating document:' , error);
res.status(500).send( 'Error generating document' );
}
});
app.listen(port, () => {
console.log(`Server listening at http:
});
|
Step to run the application
Step 1: Run the following command in the terminal to start the server.
node index.js
Step 2: Open the Postman app and make a post request with the following route and add the content in the body which you want to print on the pdf.
http://localhost:3000/generate-document
{
"title": "PDF generated with Express + Pippeteer",
"content": "This is my first PDF generated with Pippeteer"
}
Output:
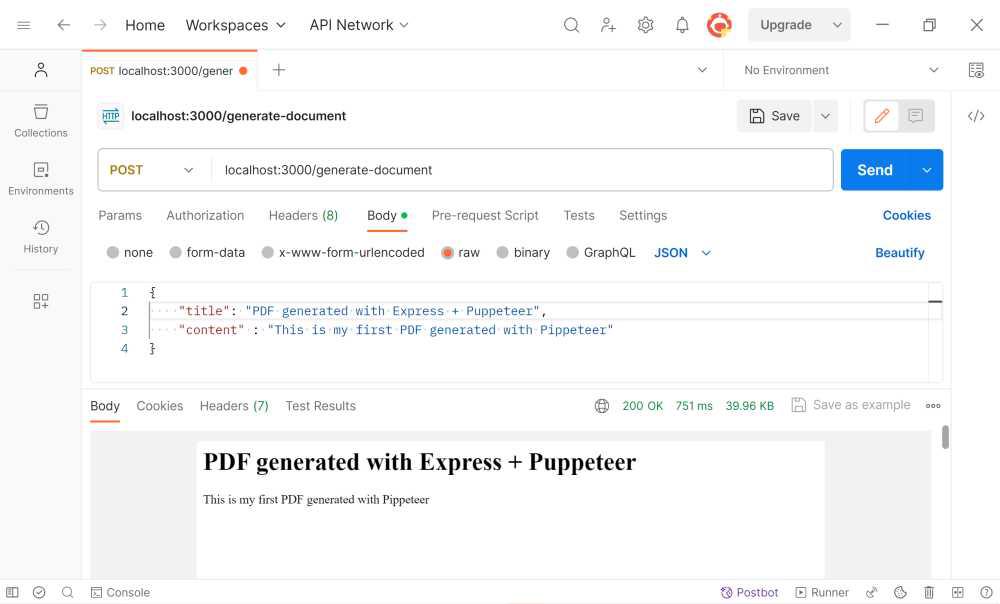
Share your thoughts in the comments
Please Login to comment...