How to fix ‘django.core.exceptions.FieldDoesNotExist’
Last Updated :
06 Dec, 2023
In this article we will explore how we can fix / solve the django.core.exceptions.FieldDoesNotExist’ error This exception raised by Django indicates that the field we are trying to access or modify is not exist. There may be some typo mistakes, field may not be there. So in this article we are looking for the solutions to fix this error.
What is ‘django.core.exceptions.FieldDoesNotExist’ ?
When working with Django, you might encounter an exception known as ‘django.core.exceptions.FieldDoesNotExist.’ This exception indicates that the field you are attempting to access or modify does not exist. This error often occurs due to typographical errors or missing fields in your models. In your Django, project, Somewhere in the project you are accessing the object with the wrong field name or with an attribute that is not mentioned in your model schema. It may happen by mistake which leads to this error. Go through some common reasons and try to identify what mistake you have done.
Example:
'django.core.exceptions.FieldDoesNotExist'
Common Reasons:
- Typing Mistakes While Accessing the Field: One of the most common reasons for this error is typing mistakes. Ensure that you access the field using the same name you defined in the model schema.
- Missing Field: It’s possible that you forgot to add a field to the schema. Double-check your model’s schema to ensure all fields are correctly defined.
- Incorrect Model: Sometimes, you might not be using the correct model in which you defined the specific field. Verify that you are working with the appropriate model.
- Migration Issues: If you made modifications to the model schema but the changes were not properly migrated, this could result in the ‘django.core.exceptions.FieldDoesNotExist‘ error. Ensure migrations are up-to-date.
- Inheritance Issues: If you are using model inheritance, make sure that the fields are accessible in the appropriate base class.
Lets try out solving this error with following possible solutions.
How to Solve ‘django.core.exceptions.FieldDoesNotExist’ ?
Method 1: Fix misspelled / incorrect field names
For instance, consider a scenario where you have defined a ‘Product’ model, but you are trying to access an object using a non-existing field name. This will trigger the ‘FieldDoesNotExist‘ error. By using the available field names as defined in your model, you can resolve this error.
Python3
from django.db import models
class Product(models.Model):
id = models.AutoField(primary_key = True )
name = models.CharField(max_length = 100 )
price = models.IntegerField()
obj = Product.objects. filter (price = 1000 )
|
Method 2: Missing/ Removal Field
If you are trying to access a field that no longer exists in the model’s schema, you have a couple of options. In this case, you can either refer to another field that does exist in the model’s schema, or you can add the missing field and then migrate the model.
To add the field you want to the model’s schema, follow these steps:
- Open your Django model file.
- Add the desired field to the model definition.
- Save the changes to the model file.
Python3
from django.db import models
class Car(models.Model):
name = models.CharField()
price = models.IntegerField()
category = models.CharField()
|
Next, you’ll need to run the following commands to migrate the changes:
python manage.py makemigrations
python manage.py migrate
Pending Migrations
If you have done any changes to model schema and you are trying to access the new field But getting this error. it may happen that the migrations you applied is not reflected in your database.
for this reasons you can try this 2 steps first for applying the migrations properly. Second for clearing the caches because cached data may not reflect the recent changes to the model.
- Apply pending migrations
- Step 1 – Delete initial migration file from the migrations folder present in your directory.
- Step 2 – Migrate it again using the command “Python manage.py makemigrations app_name” replace app_name with your installed app.
- Step 3 – Verify another migration file has been created in Migrations folder.
- Clear Cache
- Step 1 – Install django-clearcache package in your project environment.
- Step 2 – After successfull installation add it into installed apps in settings.py file.
- Step 3 – Add a url in your project base directory.
- Step 4 – Now you can use “Python manage.py clearcache” command to clear the cache.
- Step 5 – Restart the server.
Sync Database Schema
If you are experiencing this error in the development environment then this command you can try to reset the database. This will reset the database.
Python manage.py flush
click "YES"
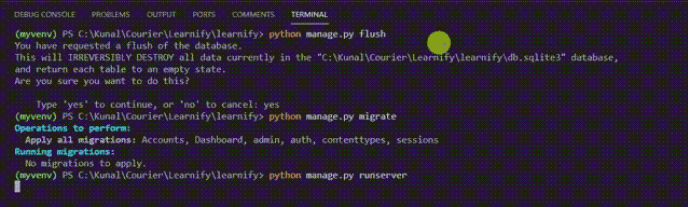
Share your thoughts in the comments
Please Login to comment...