How to fetch an array of URLs with Promise.all ?
Last Updated :
20 Nov, 2023
In this article, we will learn how to fetch an array of URLs with Promise. all ( ).
When you need information from various websites quickly, Promise.all()
is your go-to tool. It’s like having a checklist of website links and using Promise.all()
it to swiftly retrieve data from each link at the same time. Basically, it waits for all the tasks to finish before neatly handing you the combined results, saving you time and effort. It’s a smart way to efficiently gather data from multiple sources without delays.
Approach
- URLs Setup: Two specific URLs are stored in an array named urlsToFetch.
- Parallel Fetching: The fetchURLs function is designed to simultaneously fetch data from these URLs using fetch() and Promise.all().
- Data Collection: It gathers responses from all the URLs and then extracts the JSON data from these responses.
- Error Handling: The code includes error handling to catch any issues that might occur during the fetching or data extraction process.
- Results and Error Display: Upon successful data retrieval, it logs the fetched data; otherwise, it displays an error message if any issues arise during the process.
Syntax:
array of URLs to fetch data from
const urlsToFetch = ['URL1', 'URL2'];
// Map URLs to fetch promises and store in an array
const fetchPromises = urlsToFetch.map(url => fetch(url).then(response => response.json()));
// Use Promise.all() to handle all fetch promises
Promise.all(fetchPromises)
.then(responses => {
const responseData = responses.map(response => response);
})
.catch(error => console.error('Error fetching data:', error));
Example : In this example we will see how we can fetch arrays of URL like apis using Promise.all method in javascript.
Javascript
const urlsToFetch = [
];
const fetchURLs = async (urls) => {
try {
const promises =
urls.map(url => fetch(url));
const responses =
await Promise.all(promises);
const data = await
Promise.all(responses.map(response => response.json()));
return data}
catch (error) {
throw new Error(`Failed to fetch data: ${error}`)}}
fetchURLs(urlsToFetch)
.then(data => {
console.log( 'Fetched data:' , data)})
. catch (error => {
console.error( 'Error fetching data:' , error)});
|
Output:
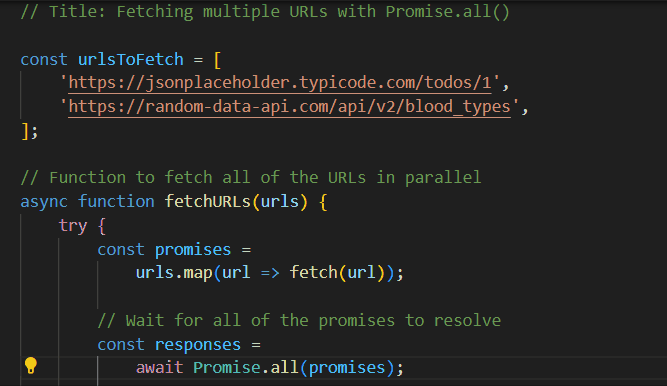
Output
Example 2 : In this example we created array of URLs and then we applied map method on that array an in each iteration we are initiating an HTTP request and storing response into fetchPromises.
Javascript
const urlsToFetch = [
];
const fetchPromises = urlsToFetch.map(url =>
fetch(url)
.then(response => response.json())
);
Promise.all(fetchPromises)
.then(responses => {
const responseData = responses.map(response => response);
console.log( 'Fetched data:' , responseData);
})
. catch (error => console.error( 'Error fetching data:' , error));
|
Output:
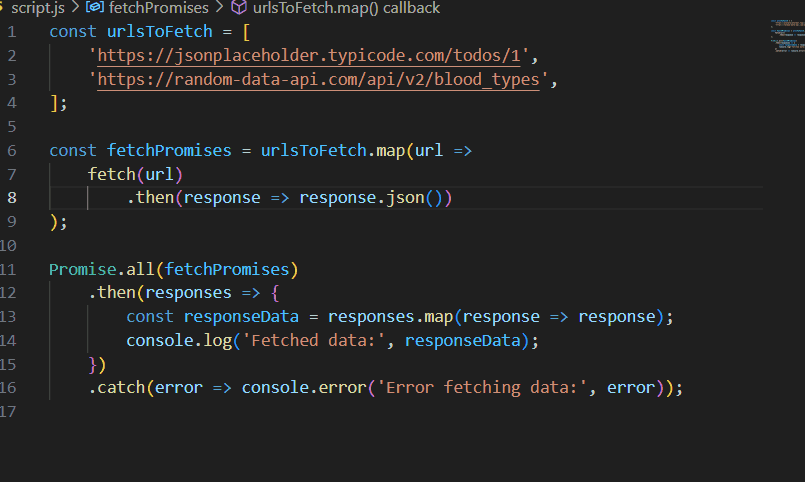
Output
Share your thoughts in the comments
Please Login to comment...