What are the states of promises in ES6 ?
Last Updated :
13 Jun, 2022
In this article, we will discuss the basic details associated with the promises followed by various states of promises in ES6.
Let us first understand some basic details which are associated with promises.
Promises:
- A Promise is an object which is used to handle all the asynchronous operations (which are obviously executed on asynchronous data coming from certain resources).
- It is somehow inter-related with the real-life promise. It is actually a future-based scenario that is though executed in the future itself.
- There are possibly two outcomes for promises: either in the future, it may be fulfilled or it may not.
- That’s how our promises are executed, whenever we define a promise it either gets resolved with time or gets rejected.
- We may declare a promise using the following syntax.
Syntax:
The following syntax can be used for declaring a promise:
// This is how we could initialize
// or set up our promise object
let promise_object = new Promise();
States of Promises:
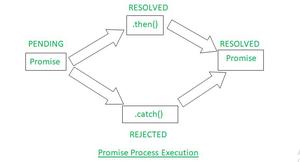
- As shown in the above pictorial representation, there are three states of promises: Resolved, Reject, Pending.
- The Pending state is the initial state where the promise doesn’t succeed or fail.
- Resolve state is another state in which promise is executed successfully.
- Reject state is the last stage in which promise is failed to be executed.
- To represent the states of promises we may follow a particular syntax.
Syntax:
The following syntax can be used for setting up the states of promises:
// At a time either a promise gets resolved
// or reject but not both.
let promise_object = new Promise(resolve, reject);
Now, we have understood the basic details associated with the promises followed by states of promises, its high time to some examples which would help us to understand all the things in a better manner.
Example 1:
- In this example, we will create a promise by following the syntax which we have seen previously.
- Along with the declaration of promises, we would be using at first the resolve() state to show how the promise can successfully a data which is passed inside the resolved method.
- Afterward, using the .then() method we would be printing out our data on the console.
Javascript
let promise = new Promise((resolve, reject) => {
resolve( "Hello World.... This is a Promise" );
})
.then((data) => console.log(data))
. catch ((error) => console.log(error));
|
Output:
Hello World.... This is a Promise
Example 2:
- In this example also, we will create a promise by following the syntax which we have seen previously.
- Along with the declaration of promises, we would be using the reject() method to show how promises can be used in case of failure of execution of any particular content.
- Afterward, we would be using the .catch() method for displaying any error message on the console.
Javascript
let promise = new Promise((resolve, reject) => {
reject( "Oops..!!! An Error Occurred" );
})
.then((data) => console.log(data))
. catch ((error) => console.log(error));
|
Output:
Oops..!!! An Error Occurred
Share your thoughts in the comments
Please Login to comment...