How to Detect if Node is Added or Removed Inside a DOM Element?
Last Updated :
22 Apr, 2024
Detecting the nodes when they are added or removed from the DOM (Document Object Model) element is a common requirement in web development. It allows developers to respond dynamically to the changes in the DOM structure enabling features such as live updates, event delegation, and more.
You can utilize the below approaches to detect the changes in the DOM:
Using Mutation Observer
The Mutation Observer API provides a way to asynchronously observe changes in the DOM. It allows developers to specify a callback function that gets invoked whenever mutations occur within the target element.
Example: The below code implements the mutation observer API to detect changes in the DOM.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
Node Mutation Observer
</title>
</head>
<body style="text-align: center;">
<div id="target">
<h3 id="heading1">
This is the target node.
</h3>
</div>
<button onclick="addParagraph()">
Add Heading
</button>
<button onclick="removeFirstChild()">
Remove Heading
</button>
<p id="result"></p>
<script>
const targetNode =
document.getElementById('target');
function callback(mutationsList, observer) {
mutationsList.forEach((mutation) => {
if (mutation.type === 'childList') {
for (let node of mutation.addedNodes) {
result.innerText =
"Added Element: " + node;
}
for (let node of mutation.removedNodes) {
result.innerText =
"Removed Element: " + node;
}
}
});
}
const observer =
new MutationObserver(callback);
const result =
document.getElementById('result');
const config =
{ childList: true, subtree: true };
observer.observe(targetNode, config);
function addParagraph() {
const newHeading =
document.createElement('h4');
newHeading.textContent =
'New heading added';
targetNode.appendChild(newHeading);
}
function removeFirstChild() {
const firstHeading =
targetNode.querySelector('h4');
if (firstHeading) {
targetNode.
removeChild(firstHeading);
} else {
console.log
("No child nodes to remove.");
}
}
</script>
</body>
</html>
Output:
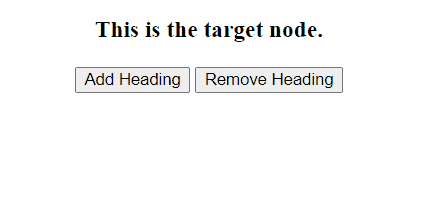
Using Event Listeners
The Event listeners can be attached to the specific events like DOMNodeInserted and DOMNodeRemoved to detect the node additions and removals within the DOM element. However, these events are deprecated and not recommended for the use in the modern web development.
Example: This example demonstrates how to detect changes to the DOM node using event listeners in JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
The Node Change Detection
</title>
</head>
<body style="text-align: center;">
<div id="target">
<h3>
Initial content
</h3>
</div>
<button id="addButton">
Add Node
</button>
<button id="removeButton">
Remove Node
</button>
<p id="result"></p>
<script>
const targetNode =
document.getElementById('target');
const result =
document.getElementById("result");
function handleNodeInserted(event) {
result.innerHTML = 'Added node is: '
+ event.target;
}
function handleNodeRemoved(event) {
result.innerHTML = 'Removed node is: '
+ event.target;
}
document.getElementById('addButton').
addEventListener('click', function () {
const newParagraph =
document.createElement('p');
newParagraph.textContent = 'New paragraph';
targetNode.appendChild(newParagraph);
});
document.getElementById('removeButton').
addEventListener('click', function () {
const firstParagraph =
targetNode.querySelector('p');
if (firstParagraph) {
targetNode.removeChild(firstParagraph);
}
});
targetNode.addEventListener
('DOMNodeInserted', handleNodeInserted);
targetNode.addEventListener
('DOMNodeRemoved', handleNodeRemoved);
</script>
</body>
</html>
Output:
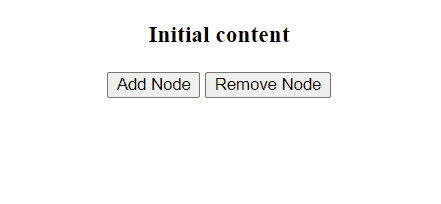
Share your thoughts in the comments
Please Login to comment...