How to Create Global Variables Accessible in all Views using Express/NodeJS ?
Last Updated :
09 Apr, 2024
In Express.js, global variables can be immensely useful for sharing data across multiple views or middleware functions. Whether it’s storing user authentication status, site-wide configurations, or any other frequently accessed data, global variables streamline development and enhance code organization.
Steps to Create React Application And Installing Module
Step 1:Â Create a nodejs application using the following command:
npm init -y
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: Install Express.js using npm
npm install express
The updated dependencies in package.json file will look like.
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.3"
}
Project Structure:
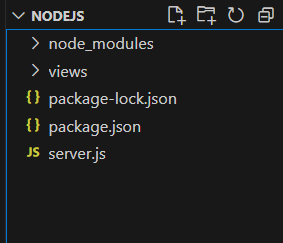
Using app.locals
app.locals in Express.js allows developers to define variables that are accessible throughout the application’s lifecycle. Once defined, these variables can be accessed in all views across your application without needing to pass them explicitly in each route handler or view render function.
Syntax:
app.locals.variableName = value;
Explanation
- app.locals is an object that represents application-level variables.
- By assigning a value to app.locals.variableName, you create a global variable that can be accessed anywhere in your Express application.
- This approach is particularly useful for storing static data or configurations that are common across all views or routes.
Example: Illustration to create global variable using app.locals
HTML
<!-- index.ejs -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>MERN</title>
</head>
<body>
<h1>
Welcome to <%= pageTitle %>
</h1>
</body>
</html>
Javascript
<!-- server.js -->
const express = require('express');
const path = require('path');
const app = express();
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Define global variable pageTitle
app.locals.pageTitle = 'My Express App';
// Example route
app.get('/', (req, res) => {
res.render('index', { pageTitle: app.locals.pageTitle });
});
// Other routes and middleware...
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Output: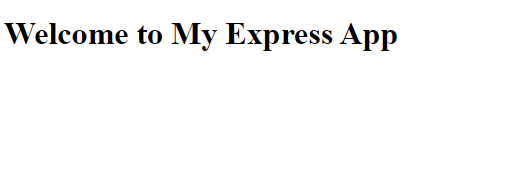
Utilizing res.locals
Unlike app.locals
, which stores application-level variables accessible across all requests, res.locals
is specific to the current request and response cycle. Once defined, variables set in res.locals
can be accessed within the current request’s route handler and any subsequent middleware functions that are part of that request chain.
Syntax:
res.locals.variableName = value;
Explanation:
- res.locals is an object that represents request-specific variables.
- By assigning a value to res.locals.variableName, you create a local variable that is available only within the current request-response cycle.
- This approach is useful for passing dynamic data to views based on each request, such as user authentication status or request-specific information.
Example: Illustration to create global variable using res.locals
HTML
<!-- index.ejs -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>MERN</title>
</head>
<body>
<h1>
Welcome to <%= pageTitle %>
</h1>
</body>
</html>
Javascript
<!-- server.js -->
const express = require('express');
const app = express();
// Set EJS as the view engine
app.set('view engine', 'ejs');
app.get('/', (req, res) => {
// Define local variable pageTitle for this request
res.locals.pageTitle = 'Home Page';
res.render('index', { pageTitle: res.locals.pageTitle });
});
app.get('/gfg', (req, res) => {
res.render('index', { pageTitle: res.locals.pageTitle });
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Output: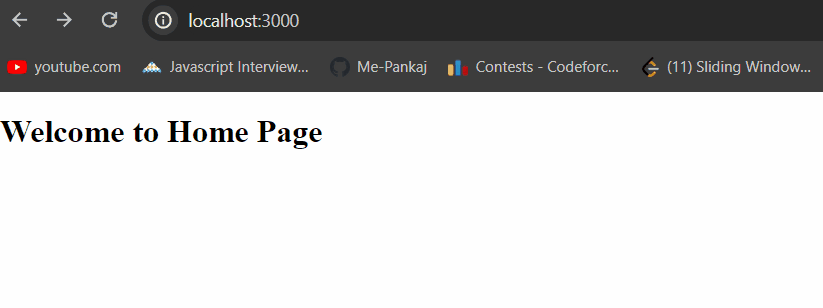
Implementing Custom Middleware
Custom middleware functions in Express.js allow developers to manipulate the request or response objects before passing them to subsequent middleware functions or routes. By setting variables in res.locals within a custom middleware, developers can ensure the availability of those variables in all views rendered during that request.
Syntax:
app.use(function(req, res, next) {
res.locals.variableName = value;
next();
});
Explanation:
- Custom middleware functions are functions that have access to the request, response, and the next middleware function in the application’s request-response cycle.
- By calling app.use() with a custom middleware function, you can define global variables that will be available in all routes and views for each request.
- This approach offers flexibility and control over when and how global variables are set, allowing for dynamic data manipulation based on the request.
Example: Illustration to create global variable via middleware.
HTML
<!-- index.ejs -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>MERN</title>
</head>
<body>
<h1>
Welcome to <%= pageTitle %>
</h1>
</body>
</html>
Javascript
<!-- server.js -->
const express = require('express');
const app = express();
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Custom middleware function to set global variable pageTitle
app.use(function (req, res, next) {
res.locals.pageTitle = 'My Express App';
next();
});
// Example route
app.get('/', (req, res) => {
res.render('index', { pageTitle: res.locals.pageTitle });
});
// Other routes and middleware...
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Output:
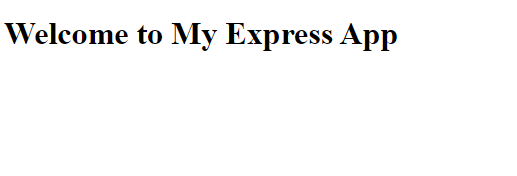
Share your thoughts in the comments
Please Login to comment...