How can you ensure that your React app is accessible?
Last Updated :
15 Apr, 2024
Ensuring that the React app is accessible involves implementing various practices and techniques to make sure that even people with disabilities can use your application effectively.
Prerequisites:
Steps to create React application:
Step 1: Create a React application using the following command:
npx create-react-app folder-name
Step 2: After creating your project folder i.e. folder-name, move to it using the following command:
cd folder-name
Step 3: Post setting up react environment on your system, we can start by creating an App.js file and create a directory by the name of components in which we will write our desired function.
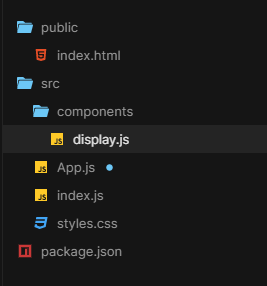
project structure
Strategies to ensure accessibility in your React app:
1. Semantic HTML:
Use semantic HTML elements (<button>, <input>, <form>, etc.) appropriately to convey the purpose and structure of your content. Avoid using non-semantic elements like <div> or <span> for interactive elements where possible.
JavaScript
//App.js
import React from 'react';
function App() {
return (
<div>
<header>
<h1>Welcome to My Website</h1>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
<li><a href="/contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<section>
<h2>About Us</h2>
<p>Learn more about our company...</p>
</section>
<section>
<h2>Contact Us</h2>
<form>
<label htmlFor="name">Name:</label>
<input type="text" id="name" name="name" />
<label htmlFor="email">Email:</label>
<input type="email" id="email" name="email" />
<label htmlFor="message">Message:</label>
<textarea id="message" name="message"></textarea>
<button type="submit">Submit</button>
</form>
</section>
</main>
<footer>
<p>© 2024 My Website. All rights reserved.</p>
</footer>
</div>
);
}
export default App;
if you observe carefully the implemented function avoids the non-semantic tags. to ensure a meaningful and accessible react application which makes the application more accessible.
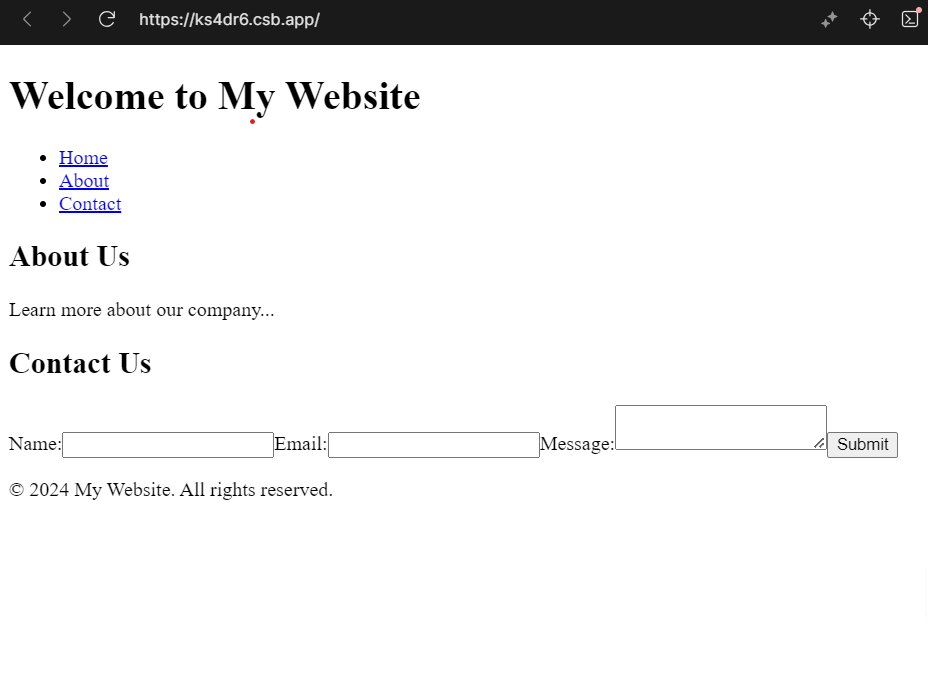
using semantic html
2. Keyboard Navigation:
Ensure that all interactive elements in the app can be accessed and operated using only the keyboard. Use the tabindex attribute to manage focus order, and ensure that keyboard focus is always visible.
JavaScript
//App.js
import React from 'react';
function App() {
return (
<div>
<h1>Tabindex Example</h1>
<p>This is a simple example demonstrating
the usage of the tabindex attribute.</p>
<button tabIndex="1">Button 1</button>
<button tabIndex="3">Button 3</button>
<button tabIndex="2">Button 2</button>
<a href="#" tabIndex="4">Link 1</a>
<a href="#" tabIndex="5">Link 2</a>
</div>
);
}
export default App;
By using the tabindex attribute, you can customize the focus order of interactive elements in your React app to improve accessibility and usability for keyboard users. However, it’s important to use tabindex judiciously and avoid disrupting the natural tab order unless necessary for accessibility reasons.
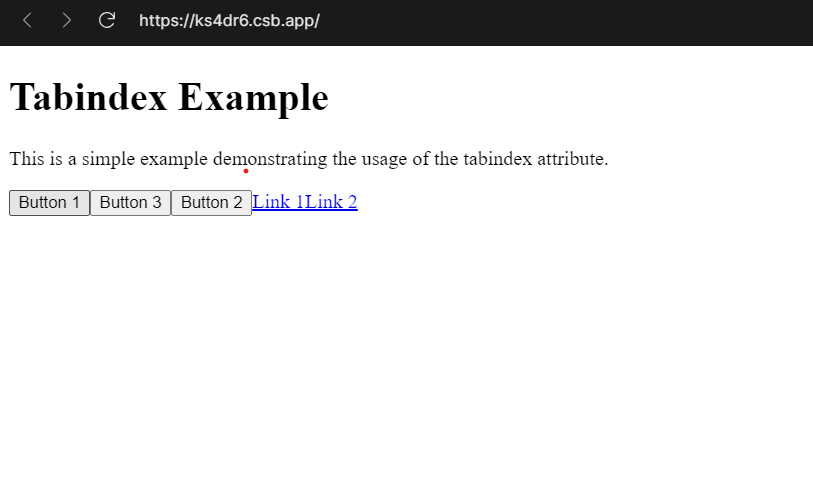
tabindex
3. Focus Management:
focus should be managed properly, especially in dynamic UI components such as modals or dropdown menus. Use the focus() and blur() methods to manage focus programmatically.
JavaScript
//App.js
import React, { useRef } from 'react';
function App() {
const inputRef = useRef(null);
const focusInput = () => {
inputRef.current.focus();
};
return (
<div>
<h1>Focus Management Example</h1>
<p>Click the button below to focus on the input field.</p>
<input type="text" ref={inputRef} />
<button onClick={focusInput}>Focus Input</button>
</div>
);
}
export default App;
By managing focus programmatically in this way, you can control the focus behavior in response to user interactions or other events in your React app, improving accessibility and user experience.
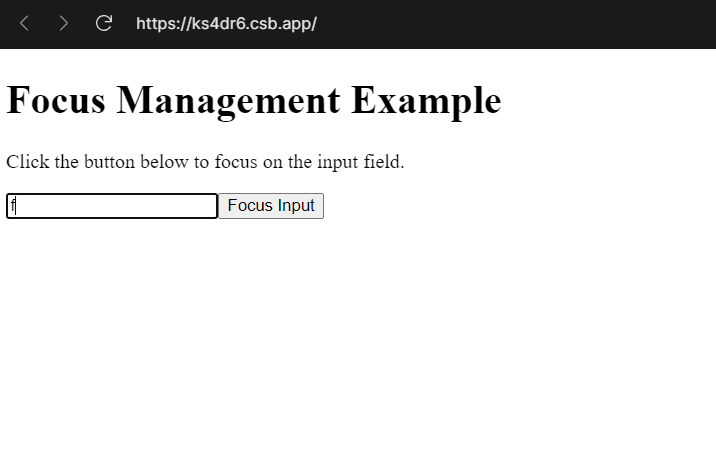
focus management
4. Accessible Images:
Include descriptive alt attributes for all images to provide context for screen readers.
JavaScript
//App.js
import React from "react";
function App() {
return (
<div>
<img
src={"give source link here"}
alt={"This is a slideshow image."}
/>
</div>
);
}
export default App;
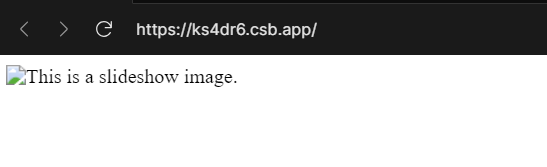
img decription usage
5. Color Contrast:
Ensure that text has sufficient color contrast against its background to be readable for users with visual impairments. Tools like the WebAIM Color Contrast Checker can help you verify color contrast ratios.
6. Screen Reader Compatibility:
Test your app with screen readers like JAWS, NVDA, and VoiceOver. Ensure that all content and interactive elements are showing correctly. This helps visually blind persons to go through your content on app.
7. Testing:
Regularly test your app for accessibility using automated testing tools like Lighthouse, Axe, or Pa11y. Manual testing with assistive technologies is also crucial to catch accessibility issues that automated tools might miss.
8. Documentation :
Provide documentation on how to use your app with accessibility features, including keyboard shortcuts and screen reader instructions.
Share your thoughts in the comments
Please Login to comment...