How to create Alarm Setter Card in Tailwind CSS and JavaScript ?
Last Updated :
12 Mar, 2024
The Alarm Setter app is a web application built with HTML, Tailwind CSS, and JavaScript. It allows users to input and set an alarm time, receiving timely notifications upon match. Through its design and functionality, users enjoy a seamless experience managing alarms. The application also includes a dismiss feature allowing users to silence the alarm and reset it for future use.
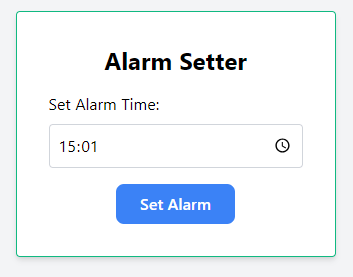
Preview
Prerequisites
Approach to create Alarm Setter Card:
- Create the basic structure of the web page using HTML elements and Integrate Tailwind CSS via CDN links for styling purposes.
- To set the background color and flexbox layout for the body use classes bg-gray-100 flex justify-center items-center h-screen
- To Create a modal for displaying the alarm notification use classes fixed top-0 left-0 w-full h-full bg-gray-900 bg-opacity-50 flex justify-center items-center hidden.
- The JavaScript code initializes a variable
alarmSet
to keep track of whether the alarm is already set. It adds an event listener to the “Set Alarm” button to handle setting the alarm. - When the button is clicked, it retrieves the entered alarm time and compares it with the current time. If the alarm time matches the current time, it plays the alarm sound and displays the modal. It also adds an event listener to the “Dismiss” button in the modal to hide the modal and stop the alarm sound when clicked.
Example: Illustration of building an Alarm Setter App in Tailwind CSS and JavaScript
HTML<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>The Alarm Setter</title>
<script src="https://cdn.tailwindcss.com"></script>
</head>
<body class="bg-gray-100 flex justify-center
items-center h-screen">
<div class="bg-white p-8 rounded shadow-md md:w-80
w-full border border-green-500">
<h1 class="text-2xl font-bold mb-4 text-center">
Alarm Setter
</h1>
<div>
<label for="alarmTime" class="block mb-2">
Set Alarm Time:
</label>
<input type="time" id="alarmTime"
class="block w-full p-2 mb-4 border
border-gray-300 rounded">
</div>
<div class="flex justify-center">
<button id="setAlarmBtn"
class="btn bg-blue-500 text-white font-semibold
py-2 px-6 rounded-lg transition
duration-300 ease-in-out">
Set Alarm
</button>
</div>
<div id="alarmMessage" class="text-center mt-4 hidden
text-green-600 font-semibold">
Alarm set successfully!
</div>
<div id="modal"
class="fixed top-0 left-0 w-full h-full bg-gray-900
bg-opacity-50 flex justify-center items-center
hidden">
<div class="bg-white p-8 rounded shadow-md md:w-80
w-full border border-green-500">
<h2 class="text-xl font-semibold mb-4 text-center">
Alarm!
</h2>
<button id="dismissModalBtn"
class="btn bg-blue-500 text-white font-semibold
py-2 px-6 rounded-lg transition
duration-300 ease-in-out">
Dismiss
</button>
</div>
</div>
</div>
<audio id="alarmSound"
src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240228225306/twirling-intime-lenovo-k8-note-alarm-tone-41440.mp3"
preload="auto">
</audio>
<script>
let alarmSet = false;
document.getElementById('setAlarmBtn')
.addEventListener('click', function () {
const alarmTime = document.getElementById('alarmTime').value;
if (!alarmSet) {
setInterval(function () {
const currentTime = new Date().toLocaleTimeString('en-US',
{ hour: '2-digit', minute: '2-digit', hour12: false });
if (alarmTime === currentTime) {
document.getElementById('alarmSound')
.play();
document.getElementById('modal')
.classList.remove('hidden');
}
}, 1000);
alarmSet = true;
document.getElementById('alarmMessage')
.classList.remove('hidden');
} else {
alert('Alarm already set!');
}
});
document.getElementById('dismissModalBtn')
.addEventListener('click', function () {
document.getElementById('modal').classList.add('hidden');
document.getElementById('alarmSound').pause();
document.getElementById('alarmSound').currentTime = 0;
});
</script>
</body>
</html>
Output:
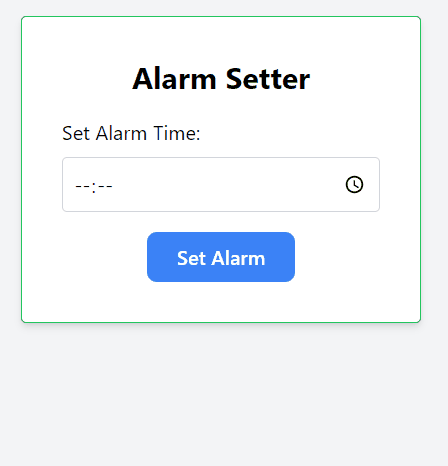
Alarm Setter Card in Tailwind CSS and JavaScript
Share your thoughts in the comments
Please Login to comment...