How to Convert String to JSON in Ruby?
Last Updated :
02 Apr, 2024
In this article, we will learn how to convert a string to JSON in Ruby. String to JSON conversion consists of parsing a JSON-formatted string into a corresponding JSON object, enabling structured data manipulation and interoperability within Ruby applications.
Converting String to JSON in Ruby
Below are the possible approaches to convert string to JSON in Ruby.
Approach 1: Using json.parse
- In this approach, we are using the JSON.parse method in Ruby, which is part of the json library.
- This method takes a JSON-formatted string (json_string) as input and converts it into a Ruby hash (json_object), effectively parsing the JSON data structure and making it accessible as a native Ruby object.
In the below example, json.parse is used to convert string to JSON in Ruby.
Ruby
require 'json'
json_string = '{"name": "GFG", "age": 30, "city": "Noida"}'
json_object = JSON.parse(json_string)
puts json_object
Output:
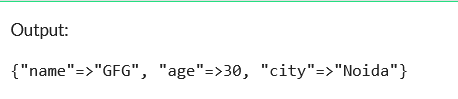
Approach 2: Using eval Method
- In this approach, we are using the eval method in Ruby to directly evaluate the JSON-formatted string (json_string) as Ruby code.
- This method treats the string as executable code, effectively converting it into a Ruby hash (json_object).
In the below example, eval method is used to convert string to JSON in Ruby.
Ruby
json_string = '{"name": "GFG", "age": 30, "city": "Noida"}'
json_object = eval(json_string)
puts json_object
Output:
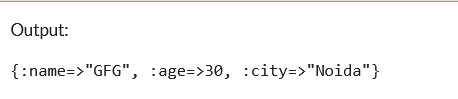
Approach 3: Using yaml.load
- In this approach, we are using the YAML.load method in Ruby after requiring the ‘yaml’ library.
- Although YAML is primarily used for its own format, it can also parse JSON-like strings with basic structures.
In the below example,yaml.load is used to convert string to JSON in Ruby.
Ruby
require 'yaml'
json_string = '{"name": "GFG", "age": 30, "city": "Noida"}'
json_object = YAML.load(json_string)
puts json_object
Output:
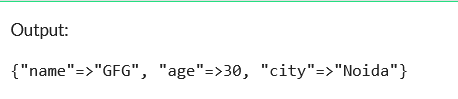
Share your thoughts in the comments
Please Login to comment...