How to Convert an Object to a JSON String in Typescript ?
Last Updated :
17 Apr, 2024
In TypeScript, an object is a collection of related data and functionality. Objects are made up of properties and methods. Properties describe the object, methods describe what it can do.
Using JSON.stringify()
- Define an interface Course that describes the shape of the object with the required properties and their types.
- Declare the course variable with the Course type. This ensures the object matches the interface shape.
- Assign the object literal to the course – TypeScript will check it matches the interface.
- Call JSON.stringify() on the typed object.
Example: The below code will explain the use of the JSON.stringify() method to convert an object to JSON string.
Javascript
interface Course {
courseName: string;
courseFees: number;
}
const course: Course = {
courseName: "Javascript",
courseFees: 30000,
};
const jsonString = JSON.stringify(course);
console.log(jsonString);
Output:
{
"courseName": "Javascript",
"courseFees": 30000
}
Using json-stringify-safe library
Steps to use JSON-stringify-safe library with TypeScript:
Step 1: Initialize a New Project
Open your terminal or command prompt and navigate to the directory where you want to create your TypeScript project. Then run the following command.
npm init
Step 2: Install TypeScript as a dev dependency
You need to install TypeScript as a dev dependency in your project using the below command.
npm install typescript --save-dev
Step 3: Create a tsconfig.json file
The tsconfig.json file contains TypeScript compiler options for your project. Run the following command.
npx tsc --init
Step 4: Install json-stringify-safe
Install the JSON-stringify-safe library using the below command.
npm install json-stringify-safe
Step 5: Install node types
Install the @types/node into your project directory using the below command.
npm install --save @types/node
Step 6: Compile TypeScript Code
Use below command to compile TypeScript code.
npx tsc index.ts
Step 7: Run Your Code
Use the below command to run the code.
node index
Project Structure:
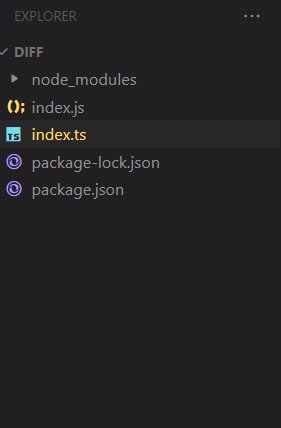
project structure
Example: The below code uses JSON-stringify-safe library to convert an object into JSON string.
Javascript
// index.ts
const stringifySafe =
require('json-stringify-safe');
interface Course {
courseName: string;
courseFees: number;
}
const course: Course = {
courseName: 'Javascript',
courseFees: 30000
}
const jsonString: string =
stringifySafe(course);
console.log(jsonString);
Output:
{
"courseName": "Javascript",
"courseFees": 30000
}
Using a Custom Serialization Function
In some cases, you may have complex objects with nested structures or non-serializable properties that need special handling when converting to JSON. In such scenarios, you can define a custom serialization function to handle the conversion process.
Example:
JavaScript
interface Course {
courseName: string;
courseFees: number;
startDate: Date;
}
const course: Course = {
courseName: "JavaScript",
courseFees: 30000,
startDate: new Date("2024-03-01"),
};
function customStringify(obj: any): string {
return JSON.stringify(obj, (key, value) => {
if (value instanceof Date) {
// Serialize Date objects to ISO string
return value.toISOString();
}
return value;
});
}
const jsonString = customStringify(course);
console.log(jsonString);
Output:
{"courseName":"JavaScript","courseFees":30000,"startDate":"2024-03-01T00:00:00.000Z"}
Share your thoughts in the comments
Please Login to comment...