How to convert a string to a constant in Ruby?
Last Updated :
10 Apr, 2024
In this article, we will learn how to convert a string to a constant in Ruby. Conversion of string to constant consists of dynamically defining a new class or module using the string’s value as the constant name, allowing for runtime creation and usage of the constant in Ruby code.
Convert a String to a Constant in Ruby
Below are the possible approaches to convert a string to a constant in Ruby.
Approach 1: Using Object.const_set
- In this approach, we are using Object.const_set to dynamically create a new constant named “HelloGFG” by converting the string “HelloGFG” into a class using Class.new.
- This allows us to instantiate objects of this class and access its properties and methods using the constant name “HelloGFG” in Ruby code.
In the below example, Object.const_set is used to convert a string to a constant in Ruby.
Ruby
# Define a string
str = "HelloGFG"
# Convert string to constant
Object.const_set(str, Class.new)
# Now we can use the constant
puts HelloGFG.new.inspect
Output:
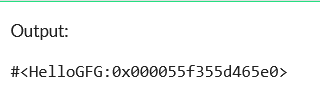
Approach 2: Using const_missing hook
- In this approach, we are utilizing the const_missing hook to dynamically create a constant named “GFG” by defining it as a class using Class.new if it is not already defined.
- This makes sure that the constant “GFG” is available for instantiation and usage in the Ruby code.
In the below example, const_missing hook is used to convert a string to a constant in Ruby.
Ruby
# Define a string
str = "GFG"
# Implement const_missing hook to handle constant creation
Object.const_set(:GFG, Class.new) unless Object.const_defined?(:MyDynamicClass)
# Now we can use the constant
puts GFG.new.inspect
Output:
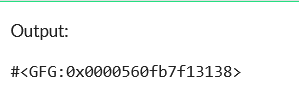
Approach 3: Using eval
- In this approach, we are using eval to dynamically convert the string “HelloGFG” into a constant by creating a new class using Class.new and assigning it to the evaluated string “#{str}“.
- This allows us to instantiate objects of the class and access its properties and methods using the constant name “HelloGFG” in Ruby code.
In the below example, eval is used to convert a string to a constant in Ruby.
Ruby
# Define a string
str = "HelloGFG"
# Convert string to constant using eval
eval("#{str} = Class.new")
# Now we can use the constant
puts HelloGFG.new.inspect
Output:
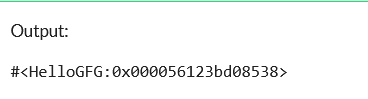
Share your thoughts in the comments
Please Login to comment...