How to Convert Map to JSON in TypeScript ?
Last Updated :
25 Apr, 2024
In TypeScript, we can convert the Map to JSON by manipulating the key-value pairs of the Map into JSON-formatted string. We can use various approaches like JSON.stringify, fast-json-stringify, and json-stringify-safe Libraries for the conversion.
Using JSON.stringify
In this approach, we are using the JSON.stringify method to convert a TypeScript Map into a JSON-formatted string. By iterating over the map entries and creating an object with key-value pairs, the output JSON string (res) prints the map data in a serialized format.
Syntax:
JSON.stringify(value: any, replacer?:
(key: string, value: any) =>
any, space?: string | number): string;
Example: The below example uses JSON.stringify to convert the map to JSON in TypeScript.
Javascript
const data: Map<string, string> = new Map([
['name', 'GeeksforGeeks'],
['type', 'Education'],
['category', 'Computer Science'],
]);
const obj: { [key: string]: string } = {};
data.forEach((value, key) => {
obj[key] = value;
});
const res: string = JSON.stringify(obj);
console.log(res);
Output:
{ name: "GeeksforGeeks", type: "Education", category: "Computer Science"}
Using fast-json-stringify Library
In this approach, we are using the fast-json-stringify library to efficiently generate a JSON stringify function with a predefined schema based on the key-value pairs of a Map. The schema is dynamically generated using Array.from(data.entries()).reduce, ensuring that each key is associated with the string type. Finally, the stringify function is applied to the converted object created from the Map, generate the JSON output.
Steps to use fast-json-stringify library with TypeScript:
Step 1: Initialize a New Project
Open your terminal or command prompt and navigate to the directory where you want to create your TypeScript project. Then run the following command.
npm init
Step 2: Install TypeScript as a dev dependency
You need to install TypeScript as a dev dependency in your project using the below command.
npm install typescript --save-dev
Step 3: Create a tsconfig.json file
The tsconfig.json file contains TypeScript compiler options for your project. Run the following command.
npx tsc --init
Step 4: Install fast-json-stringify
Install the fast-json-stringify library using the below command.
npm install fast-json-stringify
Step 5: Install node types
Install the @types/node into your project directory using the below command.
npm install --save @types/node
Step 6: Compile TypeScript Code
Use below command to compile TypeScript code.
npx tsc
Step 7: Run Your Code
Use the below command to run the code.
node index.js
Project Structure:
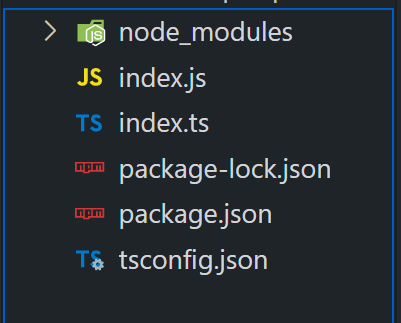
Example: The below example uses fast-json-stringify library to convert the map to JSON in TypeScript.
Javascript
const fastJsonStringify =
require('fast-json-stringify');
const data: Map<string, string> =
new Map([
['name', 'GeeksforGeeks'],
['type', 'Education'],
['category', 'Computer Science'],
]);
const temp = fastJsonStringify({
type: 'object',
properties: Array.from(data.entries()).reduce(
(acc: Record<string, { type: string }>, [key, value]) =>
{
acc[key] = { type: 'string' };
return acc;
}, {}),
});
const res = temp(Object.fromEntries(data));
console.log(res);
Output:
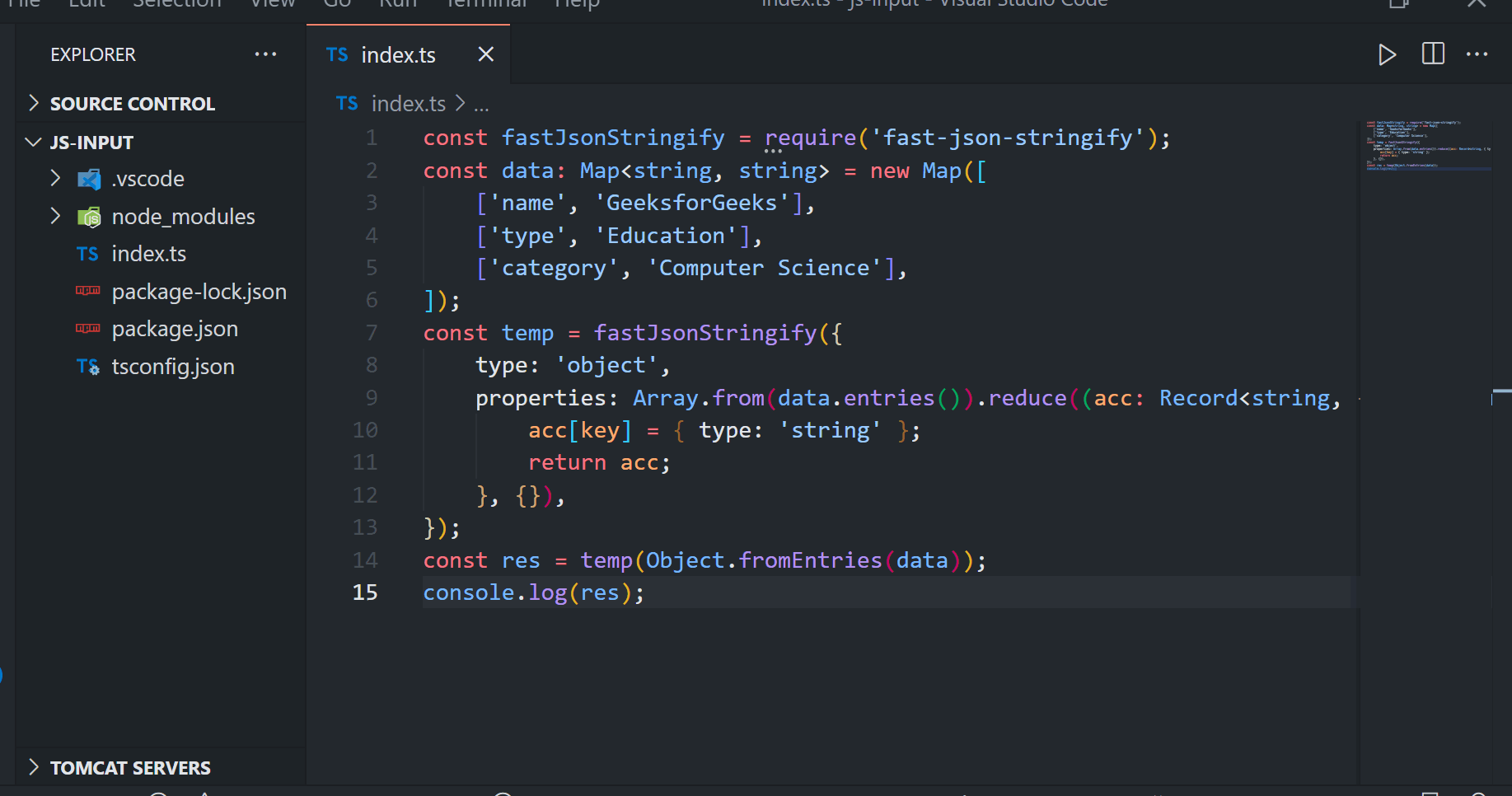
Using json-stringify-safe Library
In this approach, we are using the json-stringify-safe library to convert a TypeScript Map to JSON. We first convert the Map into a plain object using the forEach method, and then apply json-stringify-safe to get the JSON string representation.
Steps to use json-stringify-safe library with TypeScript:
Step 1: Initialize a New Project
Open your terminal or command prompt and navigate to the directory where you want to create your TypeScript project. Then run the following command.
npm init
Step 2: Install TypeScript as a dev dependency
You need to install TypeScript as a dev dependency in your project using the below command.
npm install typescript --save-dev
Step 3: Create a tsconfig.json file
The tsconfig.json file contains TypeScript compiler options for your project. Run the following command.
npx tsc --init
Step 4: Install json-stringify-safe
Install the json-stringify-safe library using the below command.
npm install json-stringify-safe
Step 5: Install node types
Install the @types/node into your project directory using the below command.
npm install --save @types/node
Step 6: Compile TypeScript Code
Use below command to compile TypeScript code.
npx tsc
Step 7: Run Your Code
Use the below command to run the code.
node index.js
Project Structure:
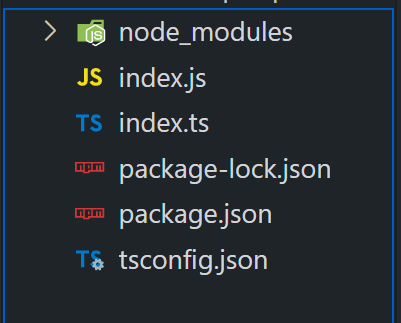
Example: The below example uses json-stringify-safe library to convert the map to JSON in TypeScript.
Javascript
const stringify = require('json-stringify-safe');
const data: Map<string, string> = new Map([
['name', 'GeeksforGeeks'],
['type', 'Education'],
['category', 'Computer Science'],
]);
const obj: { [key: string]: string } = {};
data.forEach((value, key) => {
obj[key] = value;
});
const jsonString: string = stringify(obj);
console.log(jsonString);
Output:
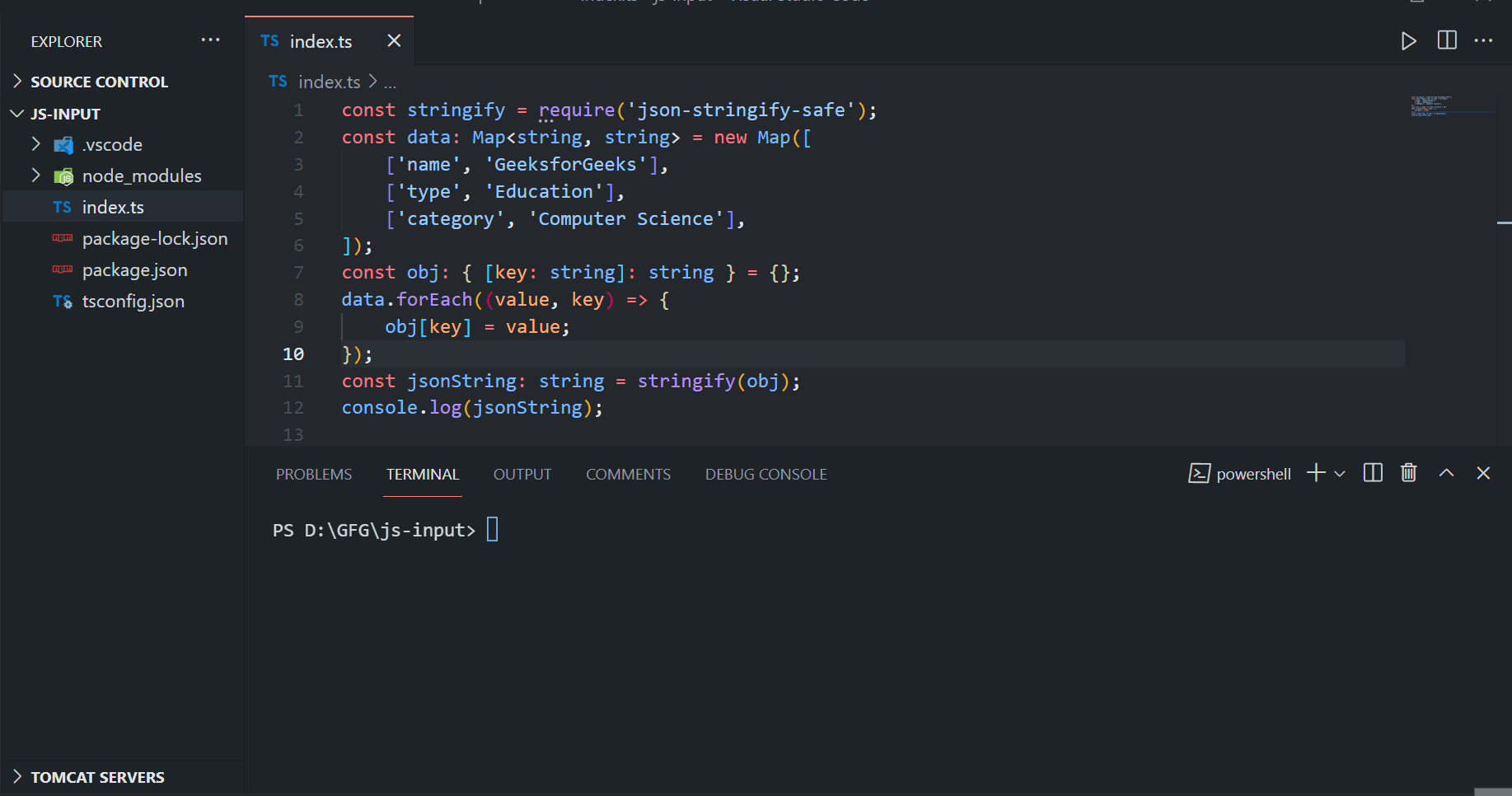
Using mapToObj Method
This approach defines a mapToObj function that iterates over the Map’s entries, assigning each key-value pair to an object. Then, it returns the resulting object. Finally, you can stringify the resulting object using JSON.stringify() if you need a JSON string representation.
Example: In this example we defines a function mapToObj() to convert a Map to an object, then demonstrates its usage by converting a Map to a JSON string. It converts a Map to an object and stringifies it.
JavaScript
// Define a function to convert Map to object
function mapToObj(map: Map<any, any>): { [key: string]: any } {
const obj: { [key: string]: any } = {};
map.forEach((value, key) => {
obj[key] = value;
});
return obj;
}
// Example usage
const data: Map<string, string> = new Map([
['name', 'GeeksforGeeks'],
['type', 'Education'],
['category', 'Computer Science'],
]);
const jsonObject = mapToObj(data);
const jsonString = JSON.stringify(jsonObject);
console.log(jsonString);
Output:
{"name":"GeeksforGeeks","type":"Education","category":"Computer Science"}
Share your thoughts in the comments
Please Login to comment...