How to conditionally apply CSS styles in AngularJS ?
Last Updated :
09 Oct, 2023
In AngularJS we can build the dynamic single-page application with interactive CSS embedded in it. But as usual, while developing the application, the CSS is once statically defined and used, we are not changing the CSS when the application is running. But, in AngularJS we can dynamically change the UI of the application based on the conditions and also as per the user inputs. There are various techniques through which we can conditionally apply the CSS styles in AngularJS applications. In this article, we will cover the two approaches through which we can apply the CSS to the components as per the condition or the user input.
Steps for Configuring AngularJS Application
The below steps will be followed to configure the AngularJS Application:
- Create a new folder for the project. We are using the VSCode IDE to execute the command in the integrated terminal of VSCode.
mkdir css-condition
cd css-condition
- Create the index.html file in the newly created folder, we will have all our logic and styling code in this file. We can also create separate files for HTML, CSS, and JS.
We will explore the above approaches & will understand its implementation through the illustration.
Conditionally implementing CSS styles using ng-class Directive
In this approach, we are using the ng-class directive that allows us to conditionally apply the CSS classes to HTML elements like h1, h3, etc. based on the AngularJS expressions. Using this directive we are binding the CSS classes to particular variables and expressions and then changing them as per the choice. In the below example, we are changing the color, and underlining the text which is defined in the HTML. We are doing this through the button click when a certain condition is met.
Example: Below is an example that showcases conditionally applying CSS in AngularJS in AngularJS using ng-class.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< script src =
</ script >
< title >
How to conditionally apply CSS
styles in AngularJS?
</ title >
< style >
.styled-button {
background-color: #ff4949;
color: white;
border: none;
padding: 4px 12px;
margin-right: 10px;
cursor: pointer;
}
.button-container {
display: flex;
flex-direction: row;
align-items: center;
margin-bottom: 10px;
}
.styled-button+.styled-button {
margin-left: 10px;
}
.label {
margin-right: 10px;
}
</ style >
</ head >
< body ng-controller = "myCtrl" >
< h1 ng-class =
"{'green-text': isGreen}" >
GeeksforGeeks
</ h1 >
< h3 ng-class=
"{'blue-text': isBlue,
'underline': isUnderline}">
Approach 1: Using ng-class
</ h3 >
< div class = "button-container" >
< div class = "label" >
Toggle Color:
</ div >
< button ng-click = "toggleColor()"
class = "styled-button" >
Toggle Green
</ button >
</ div >
< div class = "button-container" >
< div class = "label" >
Toggle Other Styles:
</ div >
< button ng-click = "toggleBlue()"
class = "styled-button" >
Toggle Blue
</ button >
< button ng-click = "toggleUnderline()"
class = "styled-button" >
Toggle Underline
</ button >
</ div >
< script >
var app = angular.module('myApp', []);
app.controller('myCtrl', function ($scope) {
$scope.isGreen = false;
$scope.isBlue = false;
$scope.isUnderline = false;
$scope.toggleColor = function () {
$scope.isGreen = !$scope.isGreen;
};
$scope.toggleBlue = function () {
$scope.isBlue = !$scope.isBlue;
};
$scope.toggleUnderline = function () {
$scope.isUnderline = !$scope.isUnderline;
};
});
</ script >
< style >
.green-text {
color: green;
}
.blue-text {
color: blue;
}
.underline {
text-decoration: underline;
}
</ style >
</ body >
</ html >
|
Output:
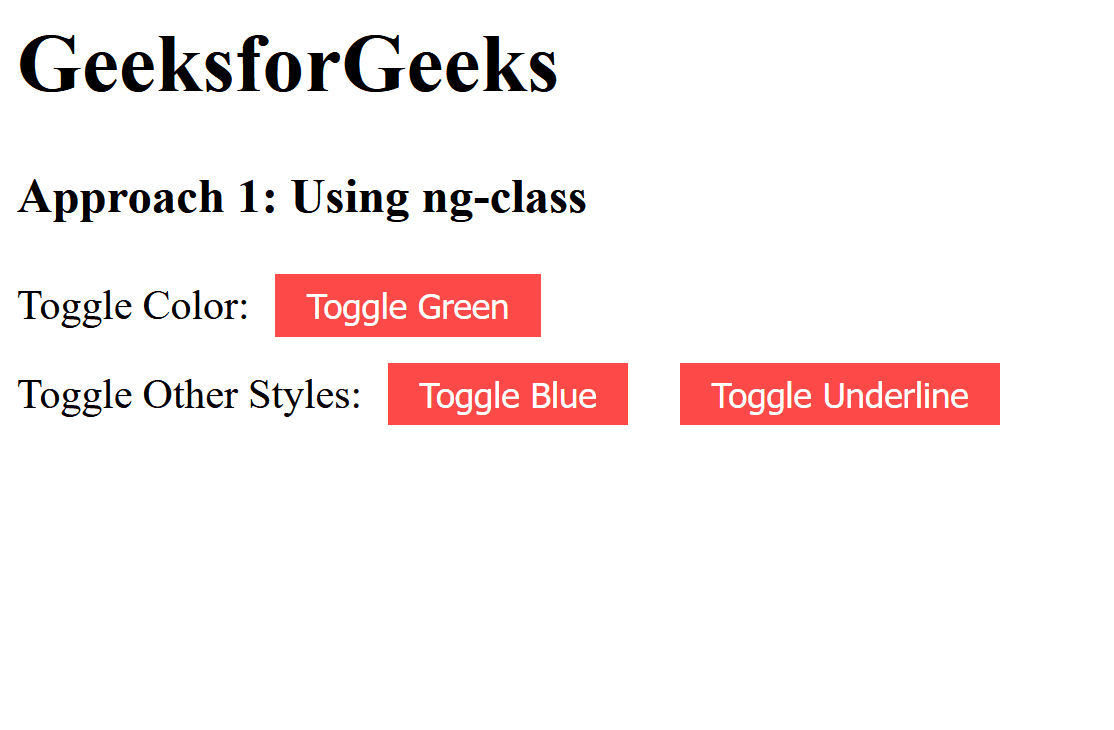
Conditionally implementing CSS styles using ng-style Directive
In this approach, we are using another directive that is ng-style, which allows us to dynamically apply the inline CSS styles that are based on the AngularJS expressions. This approach is more efficient than Approach 1, as it gives more control over the individual style properties like color, font, and background. etc. In the below example, we are changing the color of h1 (GeeksforGeeks) as per the user input, also the color of h3 and its italic flavor changes when the condition is met. The condition is like, when the user clicks on the button, the color and italic flavor get added.
Example: Below is an example that illustrates conditionally implementing CSS using the ng-style directive in AngularJS.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< title >
How to conditionally apply
CSS styles in AngularJS?
</ title >
< script src =
</ script >
< style >
.styled-button {
background-color: #007bff;
color: white;
border: none;
padding: 10px 20px;
margin-right: 10px;
cursor: pointer;
}
.button-container {
display: flex;
flex-direction: row;
align-items: center;
margin-bottom: 10px;
}
.styled-button+.styled-button {
margin-left: 10px;
}
.label {
margin-right: 10px;
}
.styled-input {
padding: 8px;
margin-right: 10px;
}
</ style >
</ head >
< body ng-controller = "myCtrl" >
< h1 ng-style =
"{'color': userColor || (isGreen ? 'green' : 'black')}" >
GeeksforGeeks
</ h1 >
< h3 ng-style = "style" >
Approach 2: Using 'ng-style'
</ h3 >
< div class = "button-container" >
< label >Select Style:</ label >
< button ng-click = "applyStyle('default')"
class = "styled-button" >
Default
</ button >
< button ng-click = "applyStyle('blue')"
class = "styled-button" >
Blue
</ button >
< button ng-click = "applyStyle('italic')"
class = "styled-button" >
Italic
</ button >
</ div >
< div class = "button-container" >
< label >Enter Custom Color:</ label >
< input type = "text"
ng-model = "userColor"
placeholder = "Enter a color name"
class = "styled-input" >
</ div >
< script >
var app = angular.module('myApp', []);
app.controller('myCtrl', function ($scope) {
$scope.isGreen = false;
$scope.style = {};
$scope.userColor = '';
$scope.applyStyle = function (selectedStyle) {
switch (selectedStyle) {
case 'default':
$scope.style = {};
break;
case 'blue':
$scope.style = {
'color': 'blue',
'font-weight': 'bold',
'font-size': '18px'
};
break;
case 'italic':
$scope.style = {
'font-style': 'italic',
'font-size': '20px'
};
break;
default:
$scope.style = {};
break;
}
};
$scope.custColor = function () {
if (/^#([0-9A-F]{3}){1,2}$/i.test($scope.userColor) ||
/(^#[0-9A-F]{6}$)|(^#[0-9A-F]{3}$)/i.test($scope.userColor)) {
$scope.style = {
'color': $scope.userColor
};
} else {
alert('Invalid color format. Please use a'+
' valid color name or hexadecimal code.');
}
};
});
</ script >
</ body >
</ html >
|
Output:
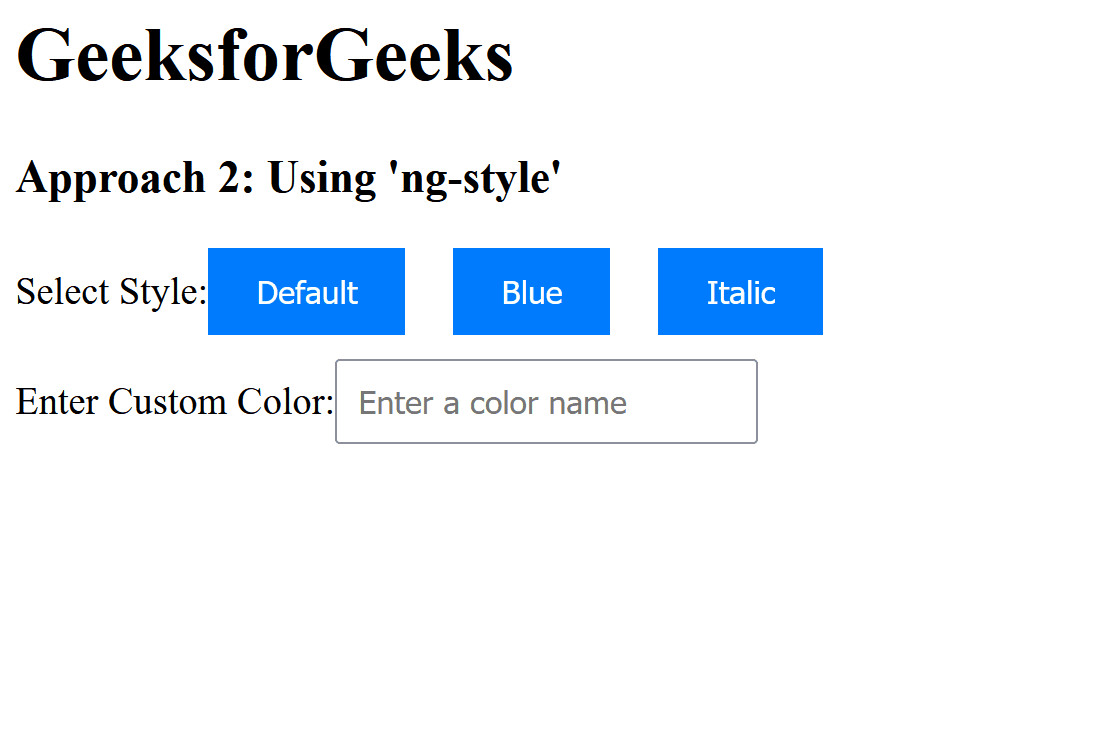
Share your thoughts in the comments
Please Login to comment...