How to Color Cells with write.xlsx
Last Updated :
12 Jun, 2023
The xlsx package in R provides functions that can be used to read, write and format excel files. In this article, we will look at one such use of the xlsx package to format and basically color the cells of an XLSX file using the XLSX package and R Programming Language.
Coloring Cells using XLSX Package
Coloring cell is a part of formatting and we can accomplish it with the help of the required methods listed below.
Syntax:
This method creates a Fill object which can be used for styling cells, and can be used along with CellStyle to color cells.
Fill(foregroundColor, backgroundColor, pattern)
where,
- foregroundColor: Takes hex colour value beginning with ‘#’, eg. “#00FF00” or a valid color belonging to colours(), eg. “green”.
- backgroundColor: Takes hex colour value beginning with ‘#’, eg. “#00FF00” or a valid color belonging to colours(), eg. “green”.
- pattern: Takes string value specifying the fill pattern style. Valid values come from constant “FILL_STYLES_”, eg. “SOLID_FOREGROUND”.
Syntax:
This method creates a cell style and returns it as a CellStyle object.
CellStyle(wb, fill, font, border, alignment, . . . )
where,
- wb: A workbook object.
- Fill: A Fill object returned by Fill().
Syntax:
setCellStyle(cell, cellStyle)
where,
- cell: A cell object. Single-cell to be formatted.
- cellStyle: A CellStyle object returned by CellStyle().
We will be working with the below sample.xlsx file for the demonstration purpose which was initially as shown below:
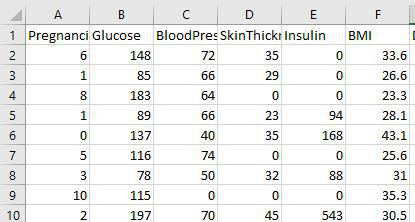
Sample.xlsx file for the demonstration purpose
You can check the full argument list and its value for Fill() and CellStyle().
R
library (xlsx)
setwd ( "D:/New folder/R" )
wb <- loadWorkbook (file= "sample.xlsx" )
sheets <- getSheets (wb)
sheet <- sheets[[1]]
rows <- getRows (sheet, rowIndex = 2:5)
cells <- getCells (rows, colIndex = 2:3)
fll <- Fill (backgroundColor= "green" ,
foregroundColor= "green" ,
pattern= "SOLID_FOREGROUND" )
br <- Border (color= "grey" ,
position= c ( "BOTTOM" , "TOP" ,
"LEFT" , "RIGHT" ),
pen= "BORDER_THIN" )
cs <- CellStyle (wb, dataFormat= NULL ,
alignment= NULL ,
border=br, fill=fll,
font= NULL , cellProtection= NULL )
for (c in cells) {
setCellStyle (c, cs)
}
saveWorkbook (wb,file= 'sample_colored.xlsx' )
|
Output:
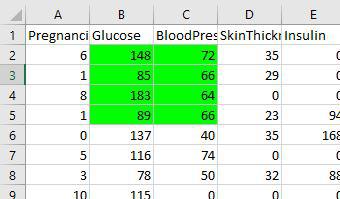
Changes made in the sample.xlsx file
Now let’s look at an example in which we will try to use conditional formatting to color cells in an xlsx file using xlsx package.
R
library (xlsx)
setwd ( "D:/Sem VIII/New folder/R" )
wb <- loadWorkbook (file= "sample.xlsx" )
sheets <- getSheets (wb)
sheet <- sheets[[1]]
rows <- getRows (sheet, rowIndex = 2:5)
cells <- getCells (rows, colIndex = 2:3)
fllGreen <- Fill (backgroundColor= "green" ,
foregroundColor= "green" ,
pattern= "SOLID_FOREGROUND" )
fllPink <- Fill (backgroundColor= "pink" ,
foregroundColor= "pink" ,
pattern= "SOLID_FOREGROUND" )
br <- Border (color= "grey" ,
position= c ( "BOTTOM" , "TOP" ,
"LEFT" , "RIGHT" ),
pen= "BORDER_THIN" )
csPink <- CellStyle (wb, border=br, fill=fllPink)
csGreen <- CellStyle (wb, border=br, fill=fllGreen)
for (c in cells) {
if ( getCellValue (c) > 15){
setCellStyle (c, csPink)
}
else {
setCellStyle (c, csGreen)
}
}
saveWorkbook (wb, file= 'sample_colored_conditional.xlsx' )
|
Output:
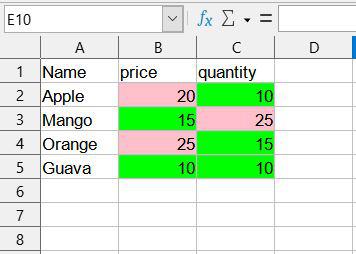
Conditional formatting using xlsx
Coloring Cells using openxlsx Package
Alternatively, we can also use the openxlsx package to color cells when writing to an Excel file. The required methods are: You can check the full argument list and its value for createStyle() and addStyle().
Here is an example of how to use the openxlsx package to write to an Excel file and color a specific cell.
R
library (openxlsx)
wb <- createWorkbook ()
addWorksheet (wb, "People" )
age <- c (12, 13, 14)
name <- c ( "John" , "Martinez" , "Gomez" )
mydata <- data.frame (name, age)
writeData (wb, "People" , mydata, startCol = 1,
startRow = 1, rowNames = TRUE )
cellStyle <- createStyle (fontColour = "#00bb0f" ,
fgFill = "yellow" )
addStyle (wb, "People" , cellStyle,
rows = 1:4, cols = 1)
setwd ( "D:/New folder/R" )
saveWorkbook (wb, "color_cell.xlsx" , overwrite = TRUE )
|
Output:
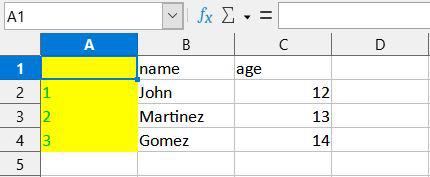
Cell colored using openxlsx
Now let’s look at an example in which we will try to use conditional formatting to color cells in an xlsx file using openxlsx package.
R
library (openxlsx)
wb <- createWorkbook ()
setwd ( "D:/New folder/R" )
addWorksheet (wb, "Car_data" )
style1 <- createStyle (fontColour = "darkgreen" ,
bgFill = "green" )
mydata <- mtcars[5:8, 1:3]
writeData (wb, "Car_data" , mydata,
startCol = 1, startRow = 1,
rowNames = TRUE )
conditionalFormatting (wb, "Car_data" , cols = 3,
rows = 1:11, style = style1,
type = "between" , rule = c (4, 7))
saveWorkbook (wb, "conditional_formatting_color.xlsx" ,
overwrite = TRUE )
|
Output:
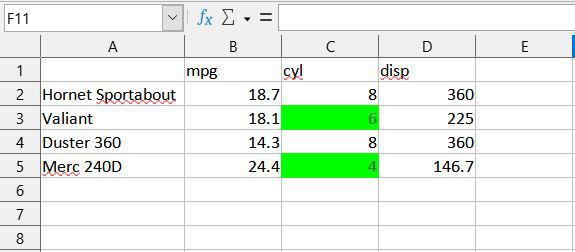
Cell colored using between conditional formatting in openxlsx
Share your thoughts in the comments
Please Login to comment...