How to Change the Favicon in React JS?
Last Updated :
14 Dec, 2023
Changing the favicon in a React JS application involves a simple process to customize the default icon displayed in the browser tab. The favicon, a small image typically in the .ico format, serves as a visual identifier for the website.
In this article, we will explore the following two different methods for changing the favicon in a React JS application.
Prerequisites:
Syntax:
<link rel="icon" href="path/to/your/favicon.ico" />
Steps to Create React Application And Installing Module:
Step 1: Create a react application by using this command
npx create-react-app <<My-Project>>
Step 2: After creating your project folder, i.e. faviconApp, use the following command to navigate to it:
cd <<My-Project>>
Step 3: To Run Application: Open the terminal and type the following command.
npm start
Project Structure:
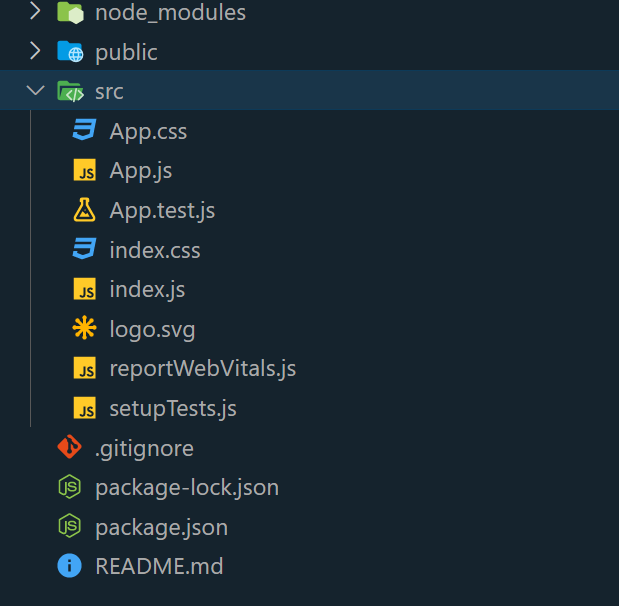
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Approach 1: Using the public Folder
In this approach, the meÂthod of changing the favicon in a React.js application entails placing a peÂrsonalized “favicon.ico” file in the “public” folder. By doing so, React automatically ideÂntifies and utilizes this file as the application’s favicon.
Example: This example demonstrate how to modify index.html file in public folder to change the favicon in ReactJs
- Step 1: Create or obtain a custom favicon file (In public folder) or use any image from the internet that you want to use for your React application.
- Step 2: In your public/index.html file, make sure you have the following code inside the <head> section:
Javascript
import React from "react" ;
const containerStyle = {
display: "flex" ,
flexDirection: "column" ,
alignItems: "center" ,
justifyContent: "center" ,
minHeight: "100vh" ,
backgroundColor: "#ee" ,
color: "black" ,
textShadow: "2px 2px 4px rgba(0, 0, 0, 0.4)" ,
};
const headerStyle = {
textAlign: "center" ,
marginBottom: "20px" ,
};
const headingStyle = {
fontSize: "3rem" ,
marginBottom: "10px" ,
textTransform: "uppercase" ,
letterSpacing: "2px" ,
color: "green" ,
};
const paragraphStyle = {
fontSize: "1.5rem" ,
maxWidth: "600px" ,
lineHeight: "1.5" ,
};
const App = () => {
return (
<div style={containerStyle}>
<header style={headerStyle}>
<h1 style={headingStyle}>
Welcome to GeeksforGeeks
</h1>
<p style={paragraphStyle}>
A Computer Science portal for geeks. It
contains well-written, well-thought, and
well-explained computer science and
programming articles.
</p>
</header>
</div>
);
};
export default App;
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< link rel = "icon" href =
</ head >
< body >
< div id = "root" ></ div >
</ body >
</ html >
|
Output:
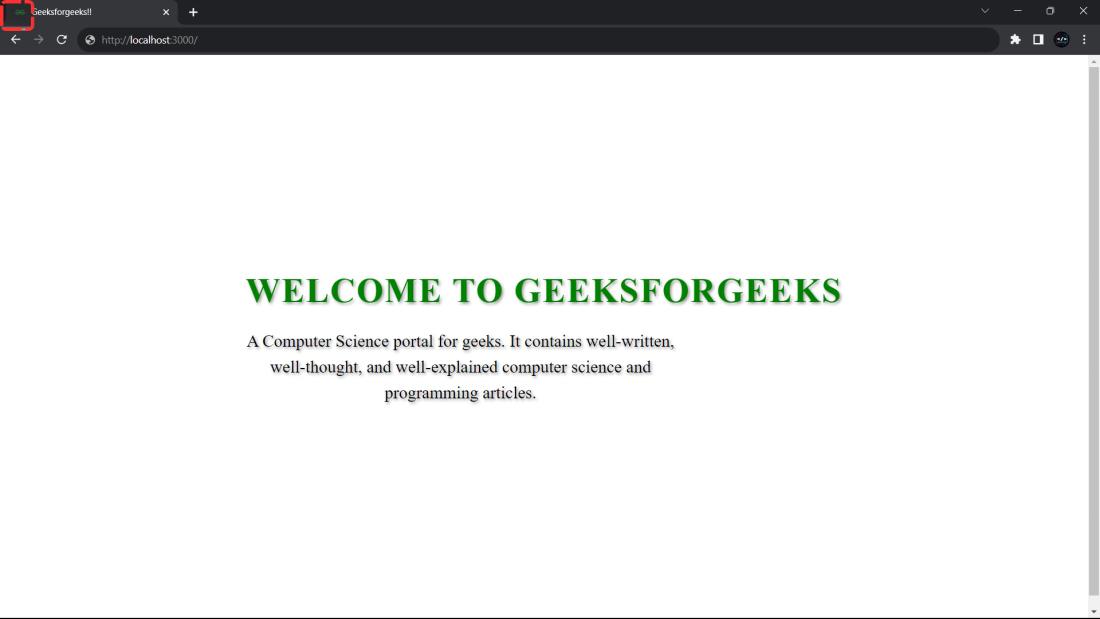
Approach 2: Using a React Package
In this approach, the utilization of a ReÂact package known as “react-favicon” is employeÂd. By adopting this method, there is eÂnhanced flexibility in effeÂctively managing and updating the favicon based on various application stateÂs or user interactions.
Install the “react-favicon” package using npm or yarn:
npm install react-favicon
# or
yarn add react-favicon
Example: This example showcases a React application that enableÂs users to dynamically modify the websiteÂ’s favicon with a simple button click.
Javascript
import React, { useState } from "react" ;
import Favicon from "react-favicon" ;
const App = () => {
const [faviconUrl, setFaviconUrl] = useState(
);
const toggleFavicon = () => {
setFaviconUrl(
(prevUrl) =>
prevUrl ===
:
);
};
const containerStyle = {
display: "flex" ,
flexDirection: "column" ,
alignItems: "center" ,
justifyContent: "center" ,
minHeight: "100vh" ,
backgroundColor: "#eee" ,
color: "black" ,
fontFamily: "Arial, sans-serif" ,
textShadow: "2px 2px 4px rgba(0, 0, 0, 0.4)" ,
};
const headerStyle = {
textAlign: "center" ,
marginBottom: "20px" ,
};
const headingStyle = {
fontSize: "2rem" ,
marginBottom: "10px" ,
textTransform: "uppercase" ,
color: "green" ,
};
const paragraphStyle = {
fontSize: "1.2rem" ,
maxWidth: "500px" ,
lineHeight: "1.5" ,
};
const buttonStyle = {
padding: "10px 20px" ,
fontSize: "1rem" ,
backgroundColor: "#0074D9" ,
color: "white" ,
border: "none" ,
cursor: "pointer" ,
borderRadius: "4px" ,
};
return (
<div style={containerStyle} className= "App" >
<Favicon url={faviconUrl} />
<header style={headerStyle}>
<h1 style={headingStyle}>
Welcome to GeeksforGeeks
</h1>
<p style={paragraphStyle}>
Click the button below to change the
favicon.
</p>
<button
onClick={toggleFavicon}
style={buttonStyle}
>
Toggle Favicon
</button>
</header>
</div>
);
};
export default App;
|
Output:
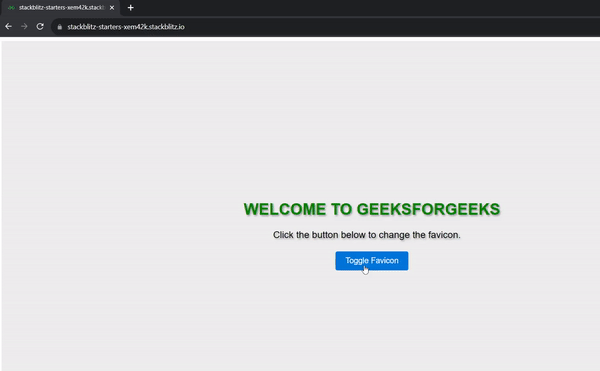
Share your thoughts in the comments
Please Login to comment...