How to cancel an $http request in AngularJS ?
Last Updated :
30 Oct, 2023
In AngularJS, we use different sources to fetch the data as per our needs. We call different data source APIs using $http requests. We can fetch the data along with cancelling the real-time running request. In AngularJS, we can use the $q service along with the promises to cancel the request. Also, there is a timeout() utility, which can cancel an $http request in AngularJS. In this article, we will see how we can demonstrate the live fetching of data and also simultaneously cancel the running http request.
Steps to Cancel an $http request in AngularJS
The below steps will be followed to cancel an $http request in AngularJS Applications.
Step 1: Create a new folder for the project. We are using the VSCode IDE to execute the command in the integrated terminal of VSCode.
mkdir cancel-http
cd cancel-http
Step 2: Create the index.html file in the newly created folder, we will have all our logic and styling code in this file.
We will understand the above concept with the help of suitable approaches & will understand its implementation through the illustration.
Cancelling an $http request using deferred object ($q.defer())
In this approach, we are cancelling the HTTP requests made to the “https://jsonplaceholder.typicode.com/comments/” using a deferred object created with the ‘$q.defer()‘. Here when we click on the “Fetch Data” button, the HTTP request is initiated using the “$http.get” method and this retrieves the ssampe comments from the API endpoint. There is a ‘canceler‘ object that is created using the ‘$q.defer()’ method that has the promise that can be used to cancel the request when the Cancel button is been clicked. The data which is fetched is displayed with a delay of 2 seconds.
Example: Below is an example that demonstrates the cancellation of an $http request in AngularJS using a deferred object ($q.defer()).
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< script src =
</ script >
< style >
h1 {
color: green;
}
</ style >
</ head >
< body ng-controller = "MainCtrl" >
< h1 >GeeksforGeeks</ h1 >
< h3 >
Approach 1: Using deferred object ($q.defer())
</ h3 >
< div >
< button ng-click = "fetchData()"
ng-disabled = "fetching" >
Fetch Data
</ button >
< button ng-click = "cancelRequest()" >
Cancel
</ button >
</ div >
< div >
< p ng-repeat = "title in fetchedData" >
{{title}}
</ p >
</ div >
< div >
< h2 >Request Log:</ h2 >
< p ng-repeat = "request in requestLog track by $index" >
{{request.method}}
Request {{request.id}} URL: {{request.url}}
</ p >
</ div >
< script >
angular.module('myApp', [])
.controller('MainCtrl', function ($scope, $http, $q, $timeout) {
var canceler;
$scope.fetchedData = [];
$scope.requestLog = [];
$scope.fetching = false;
var requestId = 1;
$scope.fetchData = function () {
$scope.fetching = true;
canceler = $q.defer();
fetchComment(1);
}
$scope.cancelRequest = function () {
if (canceler) {
canceler.resolve('Request canceled');
$scope.fetching = false;
alert('Request Canceled');
}
};
function fetchComment(commentId) {
$http.get('.../example.com/' +
commentId, { timeout: canceler.promise })
.then(function (response) {
if (response.data && response.data.name) {
var title = response.data.name;
$scope.fetchedData.push(title);
}
$scope.requestLog.push({
id: commentId,
method: 'GET',
url: '../example.com/'
+ commentId,
headers: JSON.stringify(response.headers())
});
if ($scope.fetching) {
$timeout(function () {
fetchComment(commentId + 1);
}, 2000);
}
})
.catch(function (error) {
if (error && error.status === -1) {
} else {
$scope.fetching = false;
}
});
}
});
</ script >
</ body >
</ html >
|
Output:
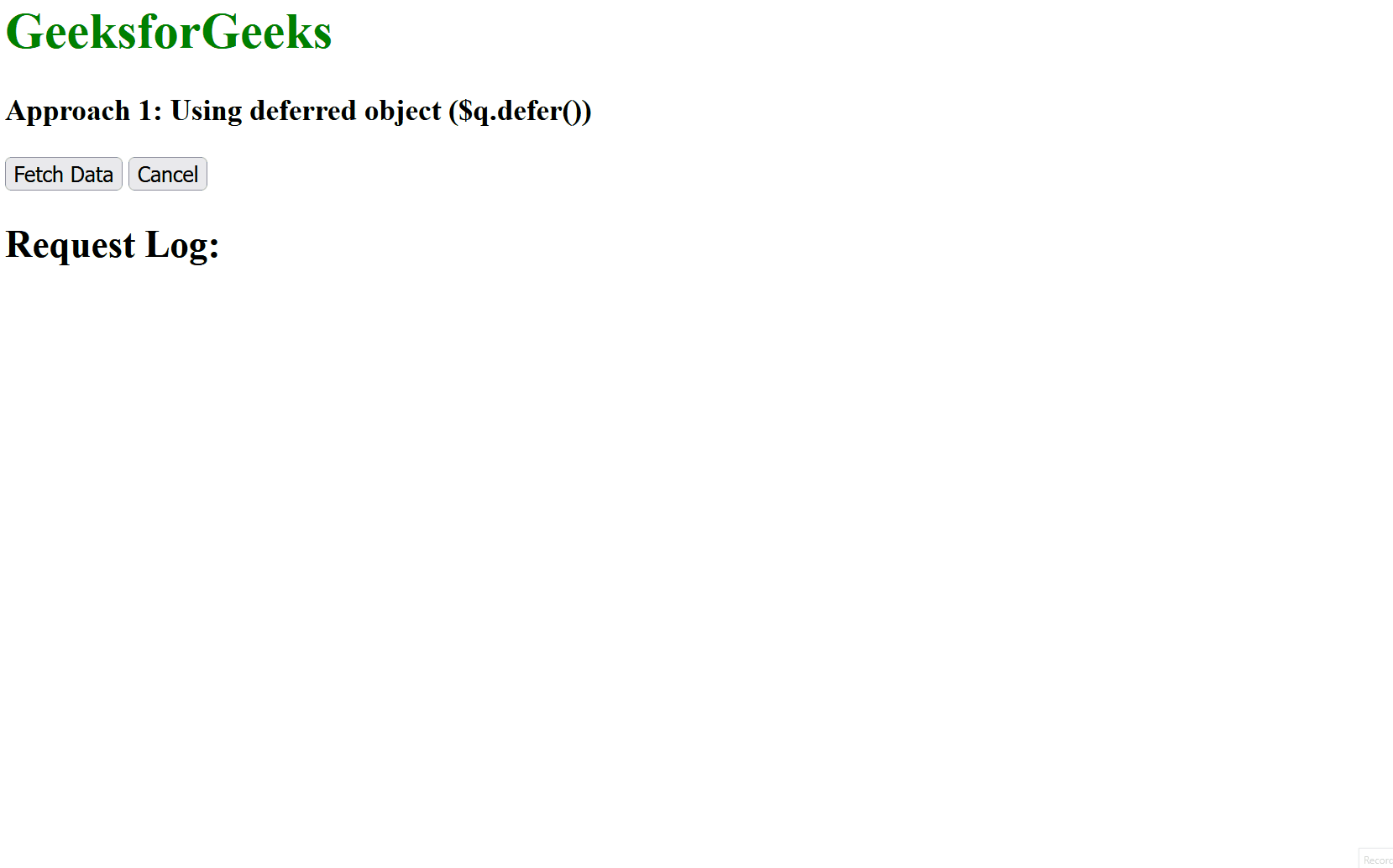
Cancelling an $http request using $timeout.cancel()
In this approach, we are using the $timeout.cancel() service to cancel the HTTP requests. When we click on the “Fetch Posts” button, the fetchPost function is been triggered which uses the $timeout service to make the delayed HTTP request. Also, the $timemout service returns the promise which represents the timer for the delayed function. When we click on the “Cancel” button, the “cancelRequest” function is been triggered in which we are using the “$timeout.cancel(requestPromise)” to cancel the delayed unction and the HTTP request.
Example: Below is an example that demonstrates the cancellation of an $http request in AngularJS using $timeout.cancel() in AngularJS.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< script src =
</ script >
< script src =
</ script >
< style >
h1 {
color: green;
}
</ style >
</ head >
< body ng-controller = "MainCtrl" >
< h1 >GeeksforGeeks</ h1 >
< h3 >
Approach 2: Using $timeout.cancel() in AngularJS
</ h3 >
< div >
< button ng-click = "fetchData()"
ng-disabled = "fetching" >
Fetch Posts
</ button >
< button ng-click = "cancelRequest()" >
Cancel
</ button >
</ div >
< div >
< p ng-repeat = "post in fetchedData" >
{{post.title}}
</ p >
</ div >
< div >
< h2 >Request Log:</ h2 >
< p ng-repeat = "request in requestLog track by $index" >
{{request.method}}
Request {{request.id}} URL: {{request.url}}
</ p >
</ div >
< script >
angular.module('myApp', ['ngResource'])
.controller('MainCtrl',
function ($scope, $resource, $timeout, $http) {
var requestPromise;
$scope.fetchedData = [];
$scope.requestLog = [];
$scope.fetching = false;
var requestId = 1;
$scope.fetchData = function () {
$scope.fetching = true;
fetchPost(1);
}
$scope.cancelRequest = function () {
if (requestPromise) {
$timeout.cancel(requestPromise);
$scope.fetching = false;
alert('Request Canceled');
}
};
function fetchPost(postId) {
requestPromise = $timeout(function () {
$http.get('.../example.com/'
+ postId)
.then(function (response) {
if (response.data && response.data.title) {
var post = response.data;
$scope.fetchedData.push(post);
}
$scope.requestLog.push({
id: postId,
method: 'GET',
url: '.../example.com/'
+ postId,
headers: JSON.stringify(response.headers())
});
if ($scope.fetching) {
fetchPost(postId + 1);
}
})
.catch(function (error) {
if (error && error.status === -1) {
} else {
$scope.fetching = false;
}
});
}, 2000);
}
});
</ script >
</ body >
</ html >
|
Output:
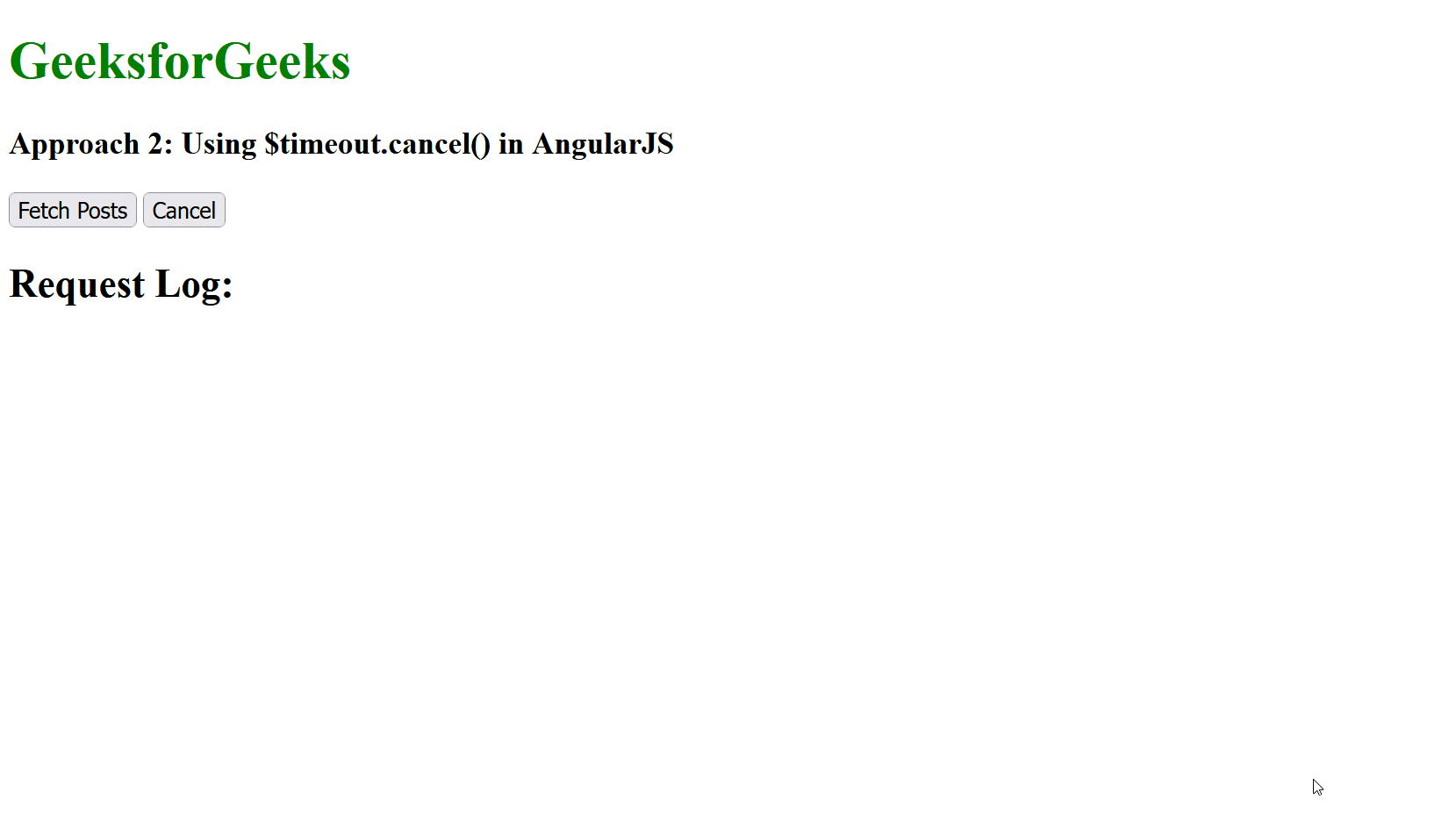
Share your thoughts in the comments
Please Login to comment...