How to call an AngularJS Function inside HTML ?
Last Updated :
30 Nov, 2023
A Function is a set of statements that takes input, does some specific computation, and produces output. In this article, we will learn How to Call an AngularJS function inside HTML. To achieve this, we can use {{…}} to call the function from HTML. We can also pass arguments and return the result back to HTML.
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2:Â After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
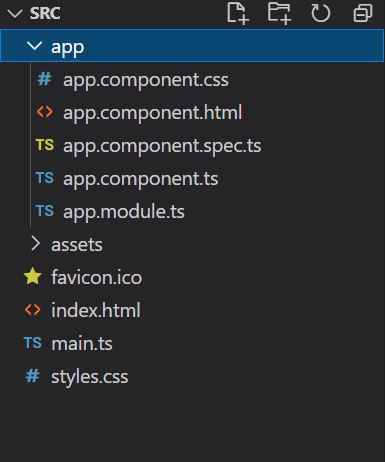
Example 1: In this example, we will call a function from HTML. This function is written in the ts file. We will call an alert() from this function.
HTML
< h2 style = "color: green" >
GeeksforGeeks
</ h2 >
< h2 >
How to Call an AngularJS function inside HTML
</ h2 >
< h3 >Open your console</ h3 >
{{displayMessage()}}
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
import { Pipe, PipeTransform } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
displayMessage() {
console.log(
"Hi GeeksforGeeks!! Happy Learning Angular!!" )
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
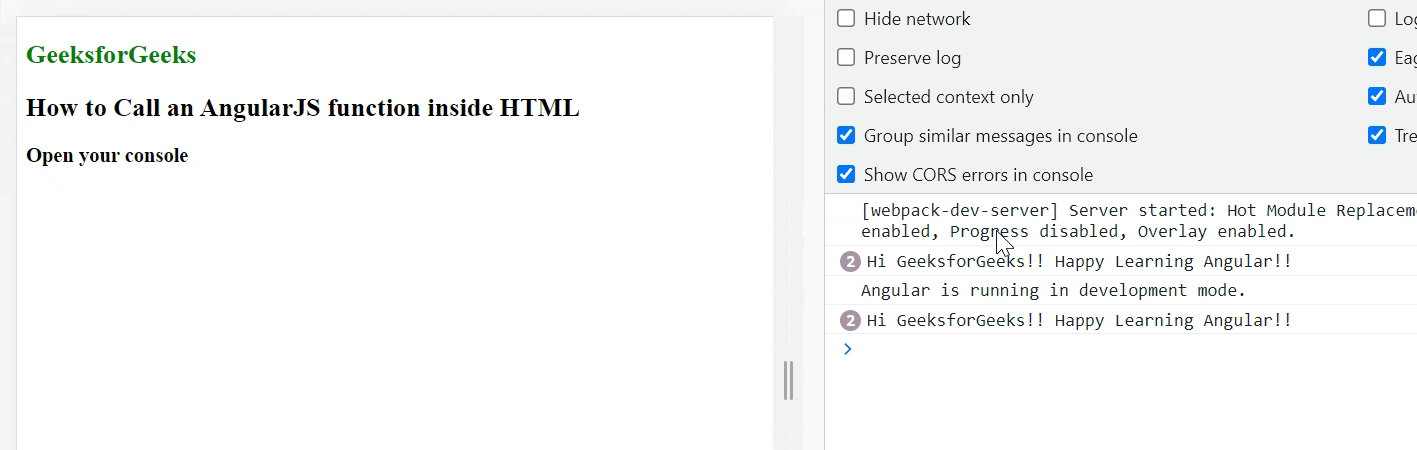
Example 2: In this example, we will see that we can pass arguments in the function, and the result will be returned by the function on the front page.
HTML
< h2 style = "color: green" >
GeeksforGeeks
</ h2 >
< h2 >
How to Call an AngularJS function inside HTML
</ h2 >
< h3 style = "color:crimson" >
{{displayMessage("Hi GeeksforGeeks!! Happy Learning Angular!!")}}
</ h3 >
< h3 style = "color: darkorange;" >
Sum of 2 and 3 is : {{getSum(2,3)}}
</ h3 >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
import { Pipe, PipeTransform } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
displayMessage(msg: string) {
return msg;
}
getSum(a: number, b: number) {
return a + b
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
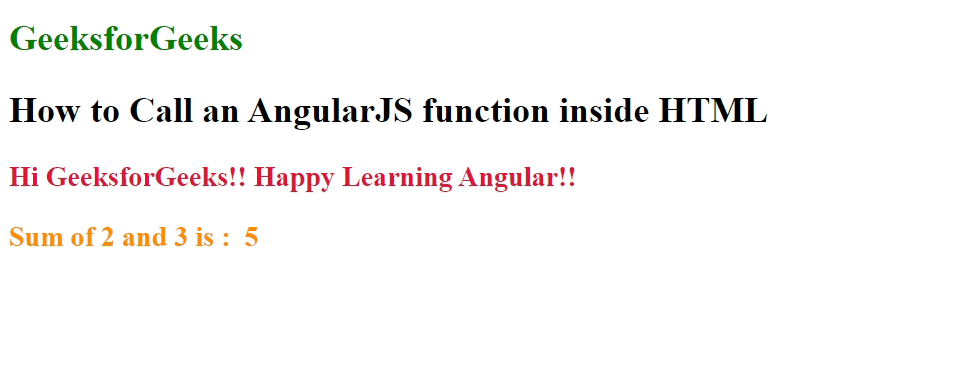
Share your thoughts in the comments
Please Login to comment...