How to call a JavaScript Function from an onmouseover Event ?
Last Updated :
15 Apr, 2024
The onmouseover event in JavaScript is triggered when the mouse pointer moves onto an element, such as a button, image, or text. We will see how to call JavaScript function from an onmouseover event in various ways, like using the onmouseover Attribute and Using Event Listener.
Using the onmouseover Attribute
In this approach, we are directly adding the onmouseover attribute to an HTML element. The HTML button element utilizes the onmouseover attribute to trigger the JavaScript function greet() when the mouse hovers over it. Upon hovering, an alert message “Welcome to GeeksForGeeks!” displays the onmouseover event’s usage to call a function.
Example: The below example uses the onmouseover attribute to call a JavaScript function from an onmouseover Event
HTML
<!DOCTYPE html>
<html>
<head>
<title>Call Function on Mouseover</title>
<style>
body {
text-align: center;
}
h1 {
color: green;
}
</style>
</head>
<body>
<h1>GeeksForGeeks</h1>
<h3>
Call Function on mouseover using
onmouseover attribute
</h3>
<button id="myButton" onmouseover="greet()">
Hover over me
</button>
<script>
function greet() {
alert('Welcome to GeeksForGeeks!');
}
</script>
</body>
</html>
Output:
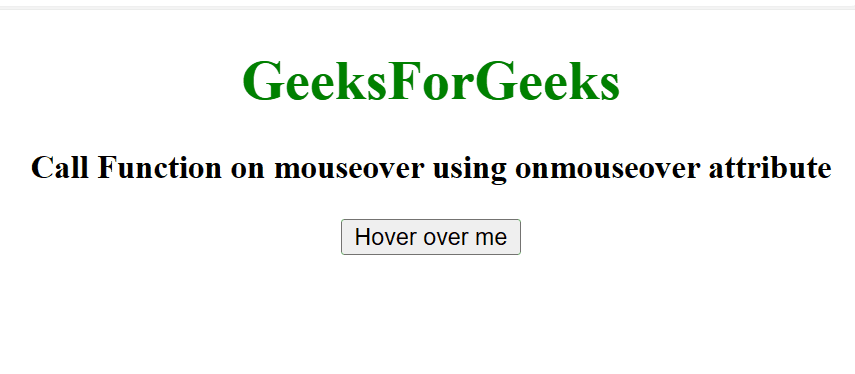
Using Event Listener
In this approach, we utilize the addEventListener method to bind an event listener to an HTML element. The JavaScript function changeText() is linked to the HTML button element using the addEventListener method, activating when the mouse hovers over it. Upon hover, the text within the paragraph element with the ID “myParagraph” is dynamically altered to “This is a New text!”.
Example: The below example uses the addEventListener method to call a JavaScript function from an onmouseover Event.
HTML
<!DOCTYPE html>
<html>
<head>
<style>
body {
text-align: center;
}
h1 {
color: green;
}
</style>
</head>
<body>
<h1>GeeksForGeeks</h1>
<h3>
Call Function on mouseover
using Event Listener
</h3>
<button id="myButton">Hover over me</button>
<p id="myParagraph">Original text</p>
<script>
// Function to change text on mouseover
function changeText() {
var paragraph =
document.getElementById('myParagraph');
paragraph.textContent = 'This is a New text!';
}
document.addEventListener('DOMContentLoaded',
function () {
// Select the button element
var button = document.getElementById('myButton');
// Attach event listener for the 'mouseover' event
button.addEventListener('mouseover', changeText);
});
</script>
</body>
</html>
Output:
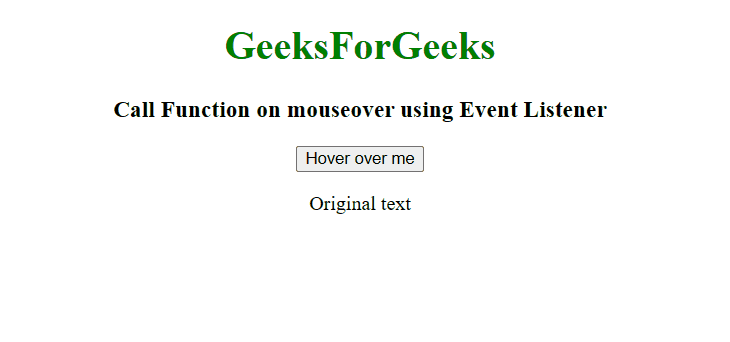
Share your thoughts in the comments
Please Login to comment...