How to Call a JavaScript Function using an onsubmit Event ?
Last Updated :
10 Apr, 2024
HTML has a form element that is used to get the data from the user and send the data to the server. Before the HTML form sends data to the server, it generates an event called “submit”.
This event can be utilized to execute a JavaScript function that can either validate the form data or notify the user of the form submission.
Below are the approaches to call a JavaScript function using an onsubmit event:
Using Inline event handler in HTML
Here in the HTML form we pass the javascript function as string to onsubmit parameter of form. when the form submit’s, It will run the function.
Syntax:
<form onsubmit="function( )">
<input type="submit">
</form>
Example: To demonstrate running a javascript function that check name validity and create alert message.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>onsubmit Event</title>
<style>
body{
display: flex;
justify-content: center;
align-items: center;
padding-top: 2rem;
}
h1{
color: #308d46;
}
</style>
</head>
<body>
<form
action="/post"
method="post"
onsubmit="formValidation(event)">
<h1>GFG Form Example</h1>
Enter name: <input type="text" name="firstName">
<input
type="submit"
value="Submit">
</form>
<script>
function formValidation(event) {
event
.preventDefault();
let name = event
.target
.firstName.value
if( name.length == 0){
alert("Enter Valid Name");
return false;
}
alert ("Form Submitted")
}
</script>
</body>
</html>
Output:
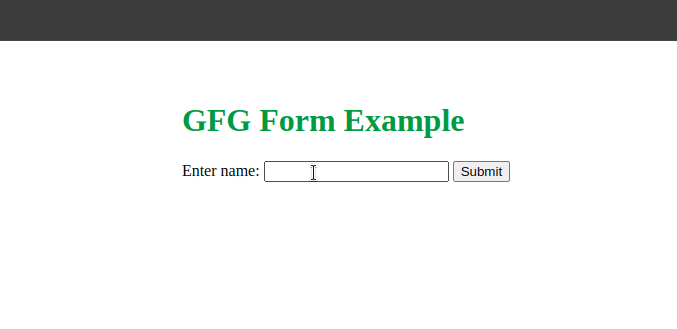
Submit form action using Inline event handler
Using on submit attribute in JavaScript
Instead of assigning the function directly inside the form parameter, we can select the form element using javascript and assign the function to onsubmit parameter from their.
Syntax:
document.getElementById("form").onsubmit = formValidation;
Note: Do not add parenthesis during function assignment.
Example: To demonstrate calling a JavaScript function from an onsubmit event using submit attribute in JavaScript
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>onsubmit Event</title>
<style>
body{
display: flex;
justify-content: center;
align-items: center;
padding-top: 2rem;
}
h1{
color: #308d46;
}
</style>
</head>
<body>
<form id="form" action="/post" method="post">
<h1>GFG Form Example</h1>
Enter name: <input type="text" name="firstName">
<input
type="submit"
value="Submit"
>
</form>
<script>
document
.getElementById("form")
.onsubmit = formValidation;
function formValidation(event) {
event
.preventDefault();
let name = event
.target
.firstName.value
if( name.length == 0){
alert("Enter Valid Name");
return false;
}
alert ("Form Submitted")
}
</script>
</body>
</html>
Output:
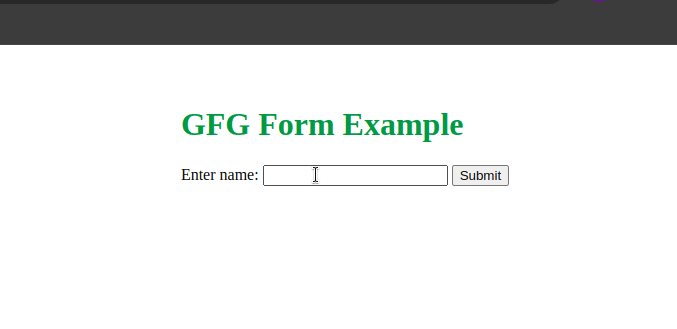
Using onsubmit attribute in JavaScript
Using addEventListener() method in JavaScript
Here instead of assigning function to onsubmit parameter of form, we attach event listener to the form element to listen for submit event.
Syntax:
document.getElementById( "form" ).addEventListener( 'submit', formValidation );
Example:To demonstrate calling a JavaScript function from an onsubmit event using event listner method in JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>onsubmit Event</title>
<style>
body {
display: flex;
justify-content: center;
align-items: center;
padding-top: 2rem;
}
h1 {
color: #308d46;
}
</style>
</head>
<body>
<form id="form" action="/post" method="post">
<h1>GFG Form Example</h1>
Enter name: <input type="text" name="firstName">
<input type="submit" value="Submit">
</form>
<script>
document
.getElementById("form")
.addEventListener('submit', formValidation);
function formValidation(event) {
event.preventDefault();
let name =
event
.target
.firstName
.value
if (name.length == 0) {
alert("Enter Valid Name");
return false;
}
alert("Form Submitted")
}
</script>
</body>
</html>
Output:
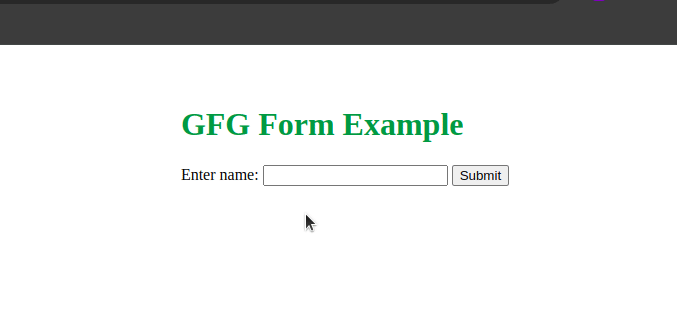
Using addEventListner method in JavaScript
Share your thoughts in the comments
Please Login to comment...