How to Call a Function with Mouse Hover in VueJS ?
Last Updated :
15 Apr, 2024
In Vue.js, the function can be triggered when the element is hovered using directives like @mouseover or @mousemove, allowing for dynamic interactions based on mouse movements.
Using @mouseover Directive
In this approach, we are using the @mouseover directive in Vue.js to trigger the mouseOverFn method when the mouse hovers over the specified element. The method sets a message and shows it for 1 second before hiding it when the mouse leaves the element.
Syntax:
<div @mouseover="methodName">...</div>
Example: The below example uses @mouseover Directive to call a function with Mouse Hover in VueJS.
HTML
<!DOCTYPE html>
<head>
<title>
Example 1
</title>
<script src=
"https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js">
</script>
<style>
.styled-link {
color: green;
text-decoration: underline;
cursor: pointer;
}
</style>
</head>
<body>
<div id="app">
<h1>
Using @mouseover Directive
</h1>
<div @mouseleave="mouseLeaveFn"
@mouseover="mouseOverFn"
class="styled-link">
Hover over me!
</div>
<p v-show="showMessage">
{{ message }}
</p>
</div>
<script>
new Vue({
el: '#app',
data: {
message: '',
showMessage: false
},
methods: {
mouseOverFn() {
if (!this.showMessage) {
this.message =
'Hello GFG! Message from Function';
this.showMessage = true;
setTimeout(() => {
this.showMessage = false;
}, 1000);
}
},
mouseLeaveFn() {
this.showMessage = false;
}
}
});
</script>
</body>
Output:
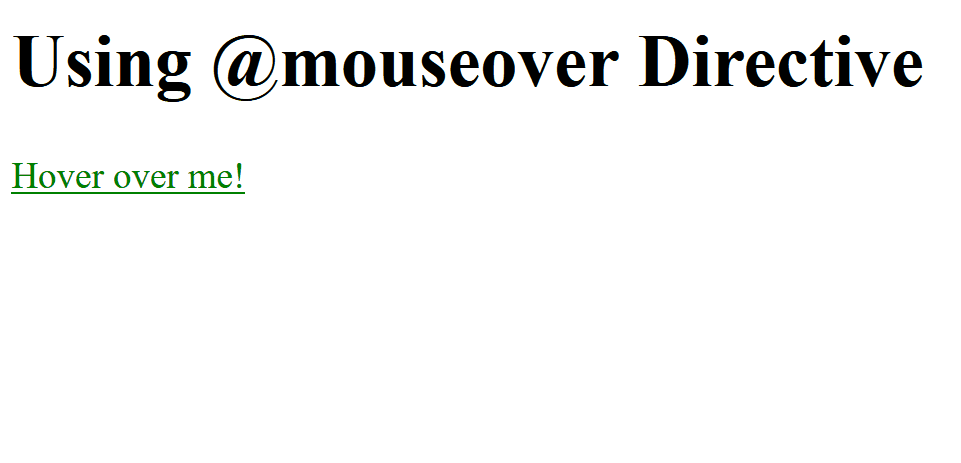
Using @mousemove Directive
In this approach, we are using the @mousemove directive in Vue.js to trigger the handleMouseMove method when the mouse is moved over the specified element. The method sets a message and shows it for 1.5 seconds before hiding it when the mouse leaves the element.
Syntax:
<div @mousemove="methodName">...</div>
Example: The below example uses @mousemove Directive to call a function with Mouse Hover in VueJS.
HTML
<!DOCTYPE html>
<head>
<title>
Example 2
</title>
<script src=
"https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js">
</script>
<style>
.styled-link {
color: rgb(255, 255, 255);
background-color: rgb(0, 0, 0);
text-decoration: underline;
cursor: pointer;
display: inline-block;
padding: 5px;
}
</style>
</head>
<body>
<div id="app">
<h1>
Using @mousemove Directive
</h1>
<div @mouseleave="handleMouseLeave"
@mousemove="handleMouseMove"
class="styled-link">
Hover over me!
</div>
<p v-show="showMessage">
{{ message }}
</p>
</div>
<script>
new Vue({
el: '#app',
data: {
message: '',
showMessage: false
},
methods: {
handleMouseMove(event) {
if (!this.showMessage) {
this.message = 'GFG is Great!';
this.showMessage = true;
setTimeout(() => {
this.showMessage = false;
}, 1500);
}
},
handleMouseLeave() {
this.showMessage = false;
}
}
});
</script>
</body>
Output:
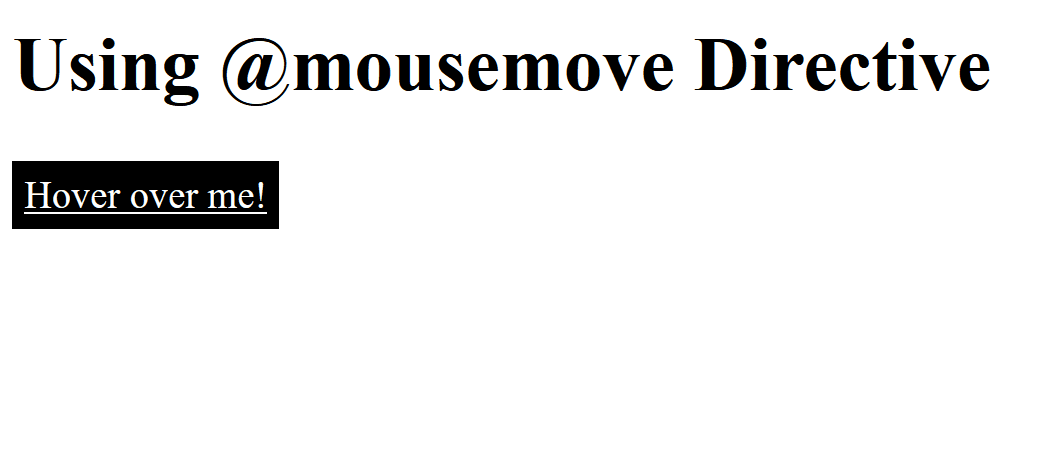
Share your thoughts in the comments
Please Login to comment...