How to Build Middleware for Node JS: A Complete Guide
Last Updated :
08 Feb, 2024
NodeJS is a powerful tool that is used for building high-performance web applications. It is used as a JavaScript on runtime environment at the server side. One of Its key features is middleware, which allows you to enhance the request and response object in your application.
In this article, we will discuss how to build middleware for NodeJS applications and the following terms.
What is Middleware?
Middleware in NodeJS is like a set of tools or helpers that helps in managing the process when your web server gets a request and sends a response. Mainly it’s work is to make the Express framework more powerful and flexible. It allows users to insert additional steps or actions in the process of handling a request and generating a response. It’s like having a toolkit that you can use to adapt how your web server works for specific tasks.
Syntax:
const createMiddleware = (req, res, next) => {
console.log('Middleware executed');
// Call next middleware function
next();
};
- req (request):
- The message of your server receives from the client.
- It holds all the details about what the client is asking for, like which URL they want and any data they’re sending.
- res (response):
- This is like the message your server sends back to the client.
- You use it to decide what to send back, like web pages, JSON data, or images.
- next:
- It’s a way to tell your server to move on to the next task.
- When you’re done with one job, you call
next()
to say, “Okay, go ahead and handle the next thing.“
Key Features of Middleware:
- Request and Response Handling: Middleware helps manage how your web server interacts with incoming requests and outgoing responses.
- Orderly Processing: You can set up middleware functions to run one after another, handling different parts of the request as needed.
- Easy Customization: You can easily add, remove, or change middleware to fit your application’s specific needs.
- Error Management: Middleware can catch and deal with errors that occur during the request-response cycle, making error handling easier.
- Common Tasks Made Easy: It’s great for doing common tasks like user authentication, logging, or data compression, saving you time and effort.
- Lots of Choices: There are tons of middleware modules available, so you can find one that does what you need without starting from scratch.
- Works Well with Frameworks: Middleware plays nicely with popular frameworks like ExpressJS, making it easy to build web applications.
- Good Performance: It’s designed to be efficient and not slow your app down, so you can focus on making your app awesome.
Steps to Create Your Middleware in Node:
Step 1: Create a new folder for your project and run.
npm init -y
Step 2: Create a file (i.e server.js) and setup a basic server.
- let’s create a simple middleware that logs information about each incoming request.
Javascript
const http = require( 'http' );
const url = require( 'url' );
function checkAgeMiddleware(req, res, next) {
const parsedUrl = url.parse(req.url, true );
const age = parsedUrl.query.age;
if (age === '18' ) {
next();
} else {
res.writeHead(403, { 'Content-Type' : 'text/plain' });
res.end( 'Access denied. You must be 18 years old.' );
}
}
const PORT = 4000;
const server = http.createServer((req, res) => {
checkAgeMiddleware(req, res, () => {
res.writeHead(200, { 'Content-Type' : 'text/plain' });
res.end( 'Welcome! You are allowed access.' );
});
});
server.listen(PORT, () => {
console.log(`Server is running at http:
});
|
Start your server using the following command.
node server.js
Output:
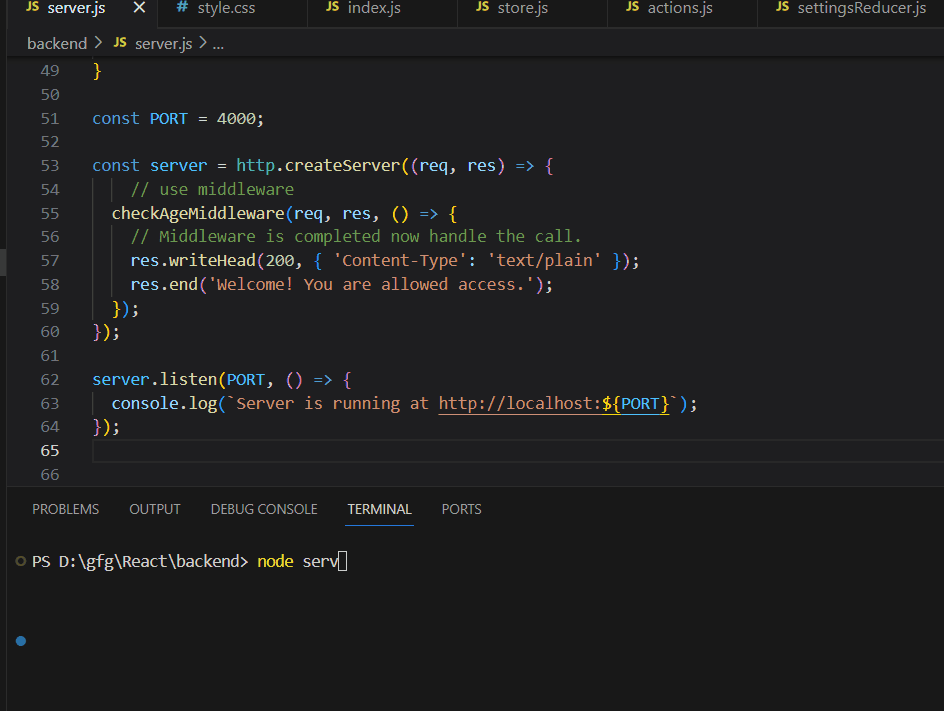
Middleware Execution Order:
The way you arrange middleware is important. Middleware functions are carried out in the order they are added using ‘app.use()‘ or ‘app.METHOD()‘. Imagine it like a sequence – if you place your logging middleware after the route handler, it won’t log anything because the response would have already been sent. So, the order of adding middleware matters for them to work as expected.
Handling Errors in Middleware:
It is used to handle erros in your application. If you pass an error to the next()
function, express will skip all remaining middleware and trigger the error-handling middleware.
Example: Below are the example of handling errors in middleware.
Javascript
const http = require( 'http' );
const PORT = 4000;
const server = http.createServer((req, res) => {
if (req.url === '/' ) {
throw new Error( 'Oops! Something Went Wrong' );
}
});
server.on( 'error' , (err) => {
console.error(err.stack);
res.writeHead(500, { 'Content-Type' : 'text/plain' });
res.end( 'Something went wrong!' );
});
server.listen(PORT, () => {
console.log(`Server is running at http:
});
|
Start your application using the following command.
node server.js
Output:
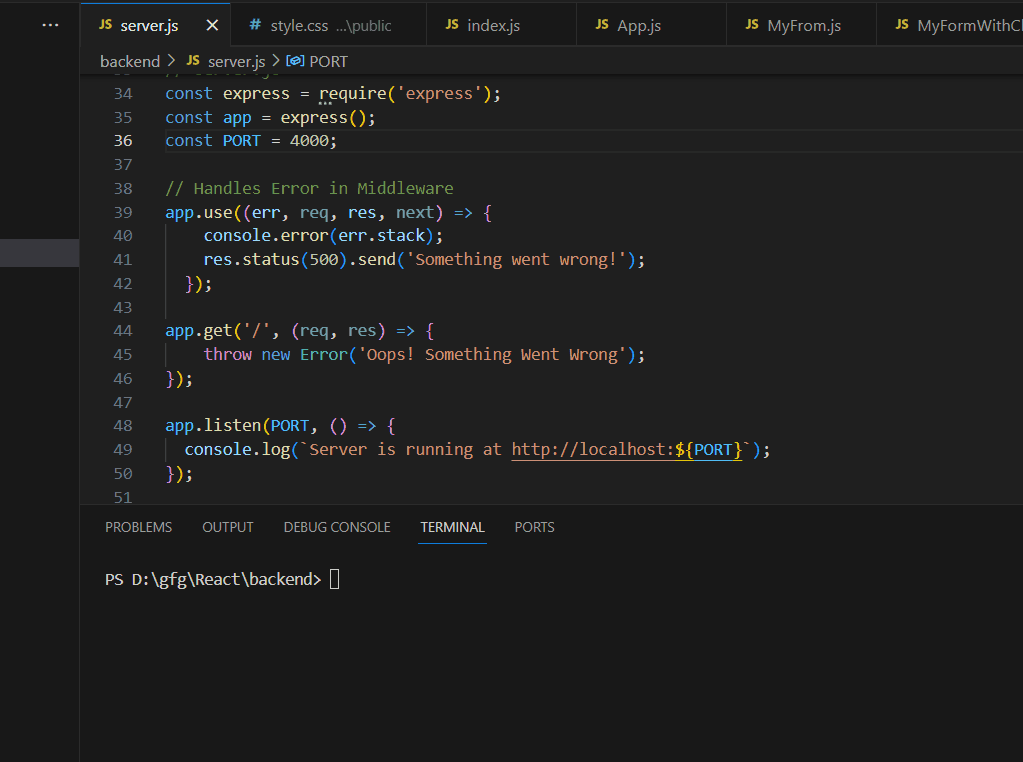
Output
Conclusion:
You’ve successfully built and implemented middleware for your Node.js application. Middleware plays a crucial role in Express, allowing you to customize and enhance your application’s functionality at various stages of the request-response cycle. Experiment with different middleware functions and explore the vast possibilities they offer in building robust and feature-rich web applications with Node.js.
Share your thoughts in the comments
Please Login to comment...