How to Add Drop Down List in Table Cell ?
Last Updated :
14 Mar, 2024
Drop-downs are the user interface elements. Drop Down List is used to select one out of various options. This article focuses on creating a drop down list in the table cell which helps in selecting the choices directly from the table itself.
Below are the approaches to add a Drop Down List in a Table Cell:
Using HTML only
In this approach, a drop-down list will be created using only in-built HTML tags. It will be a static dropdown list where options can be added only during the creation of the drop-down.
- select tag used to create the drop down.
- option tag used to create choices inside the drop down.
Example: This example describes the implementation of a drop down list using only HTML.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>
Drop Down List in Table Cell Using HTML/CSS
</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<table>
<tr>
<th>Header Text 1</th>
<th>Header Text 2</th>
</tr>
<tr>
<td>
<select>
<option value="optionText1">
Option Text 1
</option>
<option value="optionText2">
Option Text 2
</option>
<option value="optionText3">
Option Text 3
</option>
</select>
</td>
<td>Cell Text</td>
</tr>
</table>
</body>
</html>
CSS
/* style.css*/
table {
width: 80vh;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: center;
width: 35vh;
}
tr{
background-color: cadetblue;
color: white;
text-transform: uppercase;
}
select {
padding: 8px;
border: 1px solid #ccc;
border-radius: 4px;
background-color: #f1f1f1;
font-size: 16px;
cursor: pointer;
outline: none;
width: 35vh;
text-align: center;
}
select:hover {
background-color: #e0e0e0;
}
select:focus {
border-color: #214974;
box-shadow: 0 0 0 0.1rem rgba(0, 123, 255, 0.25);
}
Output:
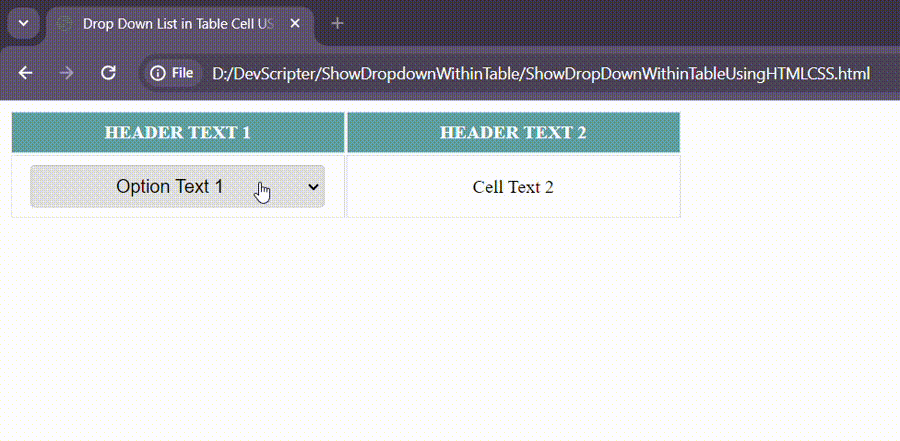
OUTPUT FOR DROPDOWN CREATED INSIDE A TABLE CELL USING HTML/CSS
Using JavaScript
In this approach, a drop down list will be created using JavaScript methods.
- document.getElementById() method is used to target the table cell in which drop down is to be created.
- document.createElement() method is used to create select and option HTML Elements.
- appendChild() – to dynamically add options in the select drop down.
Example: This example describes the implementation of a drop down list using JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Drop-down List in Table Cell</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<table>
<tr>
<th>Header Text 1</th>
<th>Header Text 2</th>
</tr>
<tr>
<td id="tableCell"></td>
<td>Cell Text</td>
</tr>
</table>
<script src="script.js"></script>
</body>
</html>
CSS
/* style.css*/
table {
width: 80vh;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: center;
width: 35vh;
}
tr{
background-color: cadetblue;
color: white;
text-transform: uppercase;
}
select {
padding: 8px;
border: 1px solid #ccc;
border-radius: 4px;
background-color: #f1f1f1;
font-size: 16px;
cursor: pointer;
outline: none;
width: 35vh;
text-align: center;
}
select:hover {
background-color: #e0e0e0;
}
select:focus {
border-color: #214974;
box-shadow: 0 0 0 0.1rem rgba(0, 123, 255, 0.25);
}
Javascript
// script.js
const select =
document.createElement("select");
const options = [
"Option Text 1",
"Option Text 2",
"Option Text 3"
];
options.forEach((option) => {
const selectOptions =
document.createElement("option");
selectOptions.value =
option.toLowerCase();
selectOptions.textContent = option;
select.appendChild(selectOptions);
});
const cell =
document.getElementById("tableCell");
cell.appendChild(select);
Output:
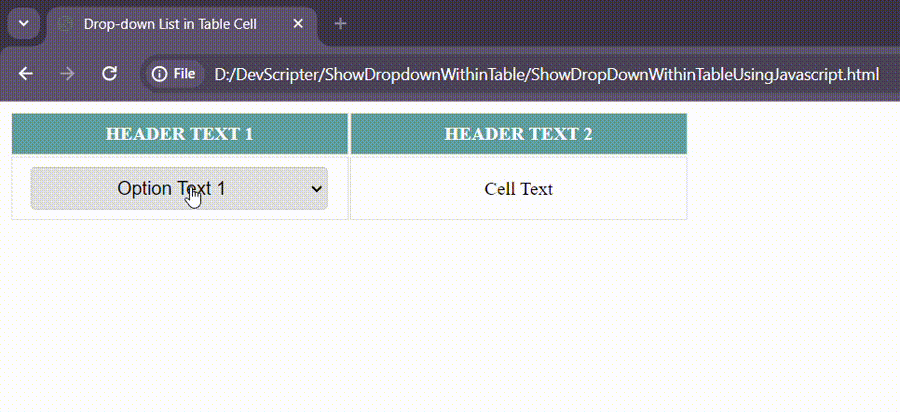
OUTPUT FOR DROPDOWN CREATED INSIDE A TABLE CELL USING JAVASCRIPT
Share your thoughts in the comments
Please Login to comment...