How to add Buttons without Submit the Form in Angular ?
Last Updated :
25 Jan, 2024
In Angular, when adding buttons to a form, their default behavior on click is to submit the form itself. However, there are certain situations in which it is necessary to create buttons for forms that provide some sort of functionality or trigger custom logic without triggering the default form submission behavior.
Steps to Configure the Angular Application
Step 1: Create an Angular application
Create an app using the Angular CLI to generate our base application structure, with routing and a CSS stylesheet.
ng new AngularApp
Step 2:Â After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
Make the directory structure according to the following image:
.png)
Using the attribute type with the value button
- In this approach, the form is not submitted by clicking any button added with an attribute of the type button. This is because the behavior of the button is modified by specifying the type of the button as a button by type = “button” which prevents the submission of the form.
- The custom button is bound to the Angular form and any kind of pre-determined logic can be added with a click of the button as per the user’s requirements.
- The submit button, on the other hand, submits the form and displays the submitted data accordingly with a ngIf directive to see if the form is submitted or not.
- In this manner, multiple buttons can be added to the form without submission of the form in Angular.
Example: Below is an example demonstrating the use of attribute type with the value of button in AngularJS. The “Increase Count” button is the custom button that is added to this form and on each click of the increase count button, the number is incremented by 1, whilst simultaneously preventing the submission of the Angular form.
HTML
< h1 >GeeksforGeeks Form</ h1 >
< div >
< form (ngSubmit)="onSubmit()">
< label for = "name" >Name</ label >
< input type = "text"
id = "name"
name = "name"
[(ngModel)]="formData.name" required>
< label for = "email" >Email</ label >
< input type = "email"
id = "email"
name = "email"
[(ngModel)]="formData.email" required>
< button type = "submit" >Submit</ button >
< button type = "button" (click)="onButtonClick()">
Increase Count
</ button >
< div >
< pre style = "margin: 1rem 0 1rem 0;" >
Count: {{ clickCount }}
</ pre >
</ div >
< div * ngIf = "displayFormData" >
< pre style = "margin-bottom: 1rem;" >
Form has been submitted successfully!
</ pre >
< pre style = "font-weight: bold;" >
Form Data:
</ pre >
< pre > {{ formData.name }} </ pre >
< pre > {{ formData.email }} </ pre >
</ div >
</ form >
</ div >
|
CSS
h 1 {
text-align : center ;
color : green ;
}
div {
margin-left : 45% ;
}
form div {
margin-top : 1 rem;
margin-left : 0 ;
}
label {
display : block ;
margin-bottom : 0.5 rem;
}
input {
display : block ;
margin-bottom : 1 rem;
}
button {
cursor : pointer ;
}
button[type= "submit" ] {
margin : 0 0.5 rem 0.5 rem 0 ;
}
|
Javascript
import { Component }
from '@angular/core' ;
import { FormsModule }
from '@angular/forms' ;
import { CommonModule }
from '@angular/common' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ],
standalone: true ,
imports: [FormsModule, CommonModule]
})
export class AppComponent {
formData: any = {
name: "GeeksforGeeks" ,
email: "xyz@geeksforgeeks.com"
};
displayFormData: boolean = false ;
clickCount: number = 0;
onSubmit() {
if (! this .displayFormData) {
this .update();
}
}
update() {
this .displayFormData = ! this .displayFormData;
}
onButtonClick() {
this .clickCount++;
}
}
|
Javascript
import { NgModule } from '@angular/core' ;
import { BrowserModule, provideClientHydration }
from '@angular/platform-browser' ;
import { AppRoutingModule }
from './app-routing.module' ;
import { AppComponent }
from './app.component' ;
@NgModule({
imports: [
BrowserModule,
AppRoutingModule,
AppComponent
],
providers: [
provideClientHydration()
],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output
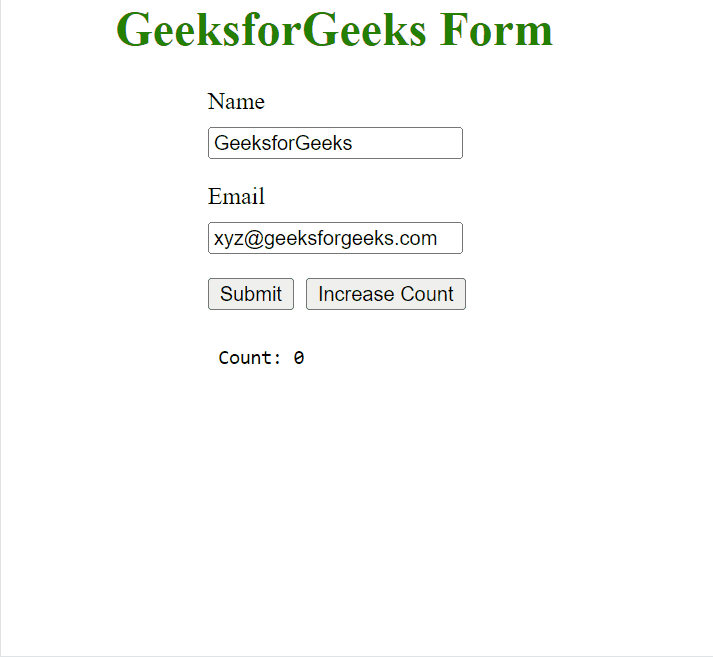
Using $event.preventDefault() Method
- In this approach, the form is not submitted by clicking any button added with an $event.preventDefault() method. This is because the behavior of the button is modified by specifying the $event.preventDefault() method on the button as this method prevents the default behavior of the form which is the submission of the form.
- Here, also, the custom button will be bound to the Angular form(as done in the 1st approach) and any kind of pre-determined logic can be added with a click of the button as per the user’s requirements. The submit button, on the other hand, submits the form and displays the submitted data accordingly with a ngIf directive to see if the form is submitted or not.
- In this manner, $event.preventDefault() can be used to add multiple buttons to the form without submission of the form in Angular.
Example: Below is an example demonstrating the use of the $event.preventDefault() method in AngularJS. The “Reset” button is the custom button that is added to this form and on each click of the reset button, the form values are cleared, whilst simultaneously preventing the submission of the Angular form.
HTML
< h1 >GeeksforGeeks Form</ h1 >
< div >
< form (ngSubmit)="onSubmit()">
< label for = "name" >Name</ label >
< input type = "text"
id = "name"
name = "name"
[(ngModel)]="formData.name" required>
< label for = "email" >Email</ label >
< input type = "email"
id = "email"
name = "email"
[(ngModel)]="formData.email" required>
< button type = "submit" >Submit</ button >
< button (click)="onButtonClick(); $event.preventDefault()">
Reset Form
</ button >
< div * ngIf = "displayFormData" >
< pre style = "margin-bottom: 1rem;" >
Form has been submitted successfully!
</ pre >
< pre style = "font-weight: bold;" >
Form Data:
</ pre >
< pre > {{ formData.name }} </ pre >
< pre > {{ formData.email }} </ pre >
</ div >
</ form >
</ div >
|
CSS
h 1 {
text-align : center ;
color : green ;
}
div {
margin-left : 45% ;
}
form div {
margin-top : 1 rem;
margin-left : 0 ;
}
label {
display : block ;
margin-bottom : 0.5 rem;
}
input {
display : block ;
margin-bottom : 1 rem;
}
button {
cursor : pointer ;
}
button[type= "submit" ] {
margin : 0 0.5 rem 0.5 rem 0 ;
}
|
Javascript
import { Component }
from '@angular/core' ;
import { FormsModule }
from '@angular/forms' ;
import { CommonModule }
from '@angular/common' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ],
standalone: true ,
imports: [FormsModule, CommonModule]
})
export class AppComponent {
formData: any = {
name: "GeeksforGeeks" ,
email: "xyz@geeksforgeeks.com"
};
displayFormData: boolean = false ;
onSubmit() {
if (! this .displayFormData) {
this .update();
}
}
update() {
this .displayFormData = ! this .displayFormData;
}
onButtonClick() {
this .formData.name = '' ;
this .formData.email = '' ;
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule, provideClientHydration }
from '@angular/platform-browser' ;
import { AppRoutingModule }
from './app-routing.module' ;
import { AppComponent }
from './app.component' ;
@NgModule({
imports: [
BrowserModule,
AppRoutingModule,
AppComponent
],
providers: [
provideClientHydration()
],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output
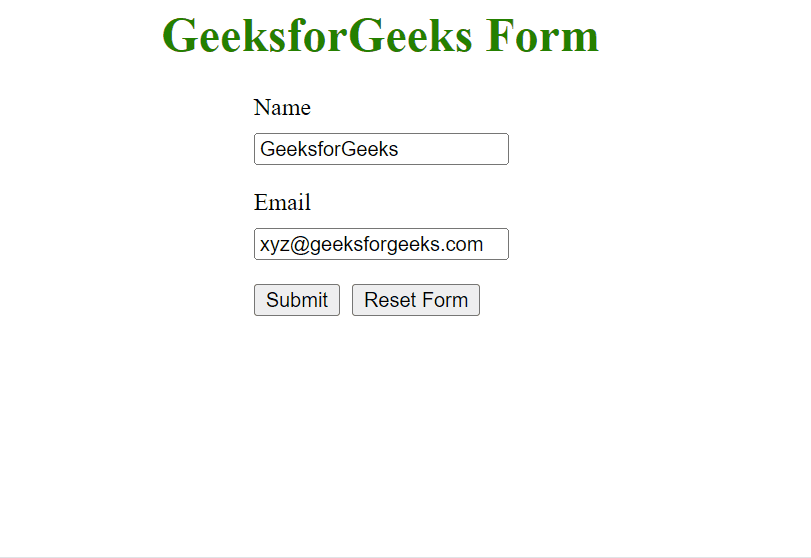
Share your thoughts in the comments
Please Login to comment...