How to access the value of a Promise in AngularJS ?
Last Updated :
19 Dec, 2023
AngularJS is one of the JS frameworks that consists of promises that are used to handle asynchronous tasks. In some scenarios, we need to handle the promise values by accessing them in the application. So, this can be done using the .then() method and also by using the callbacks in AngularJS.
In this article, we will see how we can access the value of a promise in Angular JS applications to manage and handle asynchronous tasks effectively.
Steps for Configuring the AngularJS App
The below steps will be followed to configure the AngularJS App
Step 1: Create a new folder for the project. We are using the VSCode IDE to execute the command in the integrated terminal of VSCode.
mkdir promise
cd promise
Step 2: Create the index.html file in the newly created folder, we will have all our logic and styling code in this file.
We will understand the above concept with the help of suitable approaches & will understand its implementation through the illustration.
Using .then() Method
In this approach, we have used the .then() method where we are using the $timeout service to simulate an asynchronous operation. The getData function sets a loading state, initiates a promise with a delay, and utilizes the .then() method to handle the resolved value, updating the application.
Example: Below is an example that shows how to access the value of a promise in AngularJS using .then() a method.
HTML
<!DOCTYPE html>
< html lang = "en" ng-app = "myApp" >
< head >
< meta charset = "UTF-8" >
< title >AngularJS Promise Example</ title >
< style >
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
text-align: center;
margin: 20px;
}
h1 {
color: green;
}
h2 {
color: #333;
}
button {
padding: 10px 20px;
font-size: 16px;
background-color: #009688;
color: #fff;
border: none;
cursor: pointer;
transition: background-color 0.3s ease;
}
button:hover {
background-color: #00796b;
}
.loading {
color: #333;
font-size: 18px;
margin-top: 20px;
}
.result {
margin-top: 20px;
padding: 10px;
background-color: #fff;
border: 1px solid #ddd;
border-radius: 5px;
}
</ style >
< script src =
</ script >
</ head >
< body ng-controller = "myController" >
< h1 >GeeksforGeeks</ h1 >
< h3 >Approach 1: Using .then() method</ h3 >
< button ng-click = "getData()" >
Get Data
</ button >
< div class = "loading"
ng-show = "loading" >Loading...
</ div >
< div class = "result"
ng-show = "data" >
< h3 >Hello Geek!</ h3 >
< p >Promise Resolved Value: {{ data }}</ p >
</ div >
< script >
var app = angular.module('myApp', []);
app.controller('myController',
function ($scope, $timeout) {
$scope.loading = false;
$scope.getData = function () {
$scope.loading = true;
var promise = $timeout(function () {
return "This is Data from Promise!";
}, 2000);
promise.then(function (result) {
$scope.loading = false;
$scope.data = result;
});
};
});
</ script >
</ body >
</ html >
|
Output:
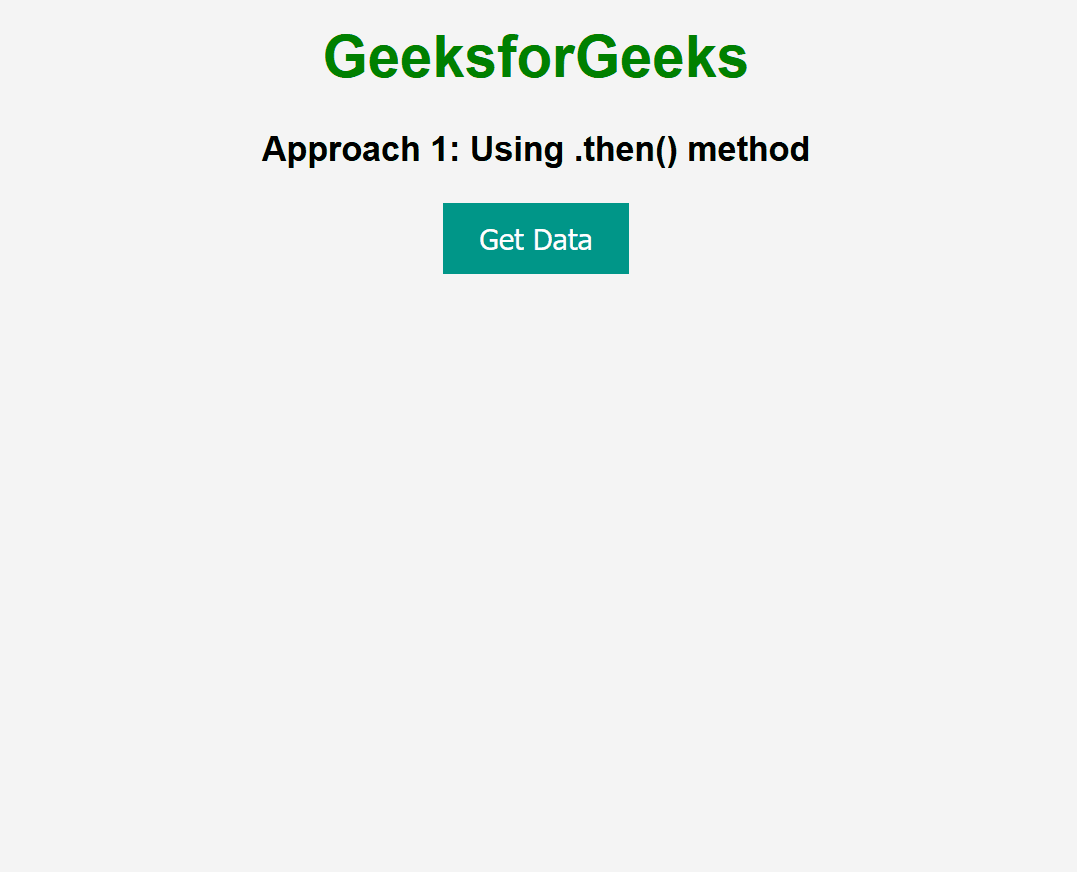
Using Callbacks
In this approach, we are using the Callbacks by implementing the $q service to manage promises. The getDataWithCallback and getDataWithAnotherPromise functions perform asynchronous operations and use callbacks to handle the resolved values. The loading state is updated during the process, and any errors are caught and displayed.
Example: Below is an example that shows how to access the value of a promise in AngularJS using Callbacks.
HTML
<!DOCTYPE html>
< html ng-app = "promiseApp" >
< head >
< title >AngularJS Promise Example</ title >
< style >
body {
font-family: 'Arial', sans-serif;
text-align: center;
margin: 50px;
}
h1 {
color: green;
}
h3 {
color: #333;
}
button {
padding: 10px 20px;
font-size: 16px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
p {
margin-top: 20px;
font-size: 18px;
color: #333;
}
.loading {
color: #777;
}
</ style >
< script src =
</ script >
</ head >
< body ng-controller = "MainController" >
< h1 >GeeksforGeeks</ h1 >
< h3 >Approach 2: Using Callbacks</ h3 >
< button ng-click = "getDataWithCallback()"
ng-disabled = "loading" >
Get Data (Callback)
</ button >
< button ng-click = "getDataWithAnotherPromise()"
ng-disabled = "loading" >
Get Another Data (Promise)
</ button >
< p ng-if = "loading"
class = "loading" >Loading...
</ p >
< p ng-if = "data" >{{ data }}</ p >
< p ng-if = "error"
style = "color: red;" >
{{ error }}
</ p >
< script >
var app = angular.module('promiseApp', []);
app.controller('MainController',
function ($scope, $q, $timeout) {
$scope.loading = false;
$scope.getDataWithCallback = function () {
$scope.loading = true;
$scope.data = null;
$scope.error = null;
var promise = $q.when("Data from Callback");
promise.then(function (result) {
$timeout(function () {
$scope.data = result;
$scope.loading = false;
}, 2000);
}).catch(function (error) {
$scope.error = "Error fetching data!";
$scope.loading = false;
});
};
$scope.getDataWithAnotherPromise = function () {
$scope.loading = true;
$scope.data = null;
$scope.error = null;
var anotherPromise =
$q.when("Another Data from Promise");
anotherPromise.then(function (result) {
$timeout(function () {
$scope.data = result;
$scope.loading = false;
}, 2000);
}).catch(function (error) {
$scope.error = "Error fetching another data!";
$scope.loading = false;
});
};
});
</ script >
</ body >
</ html >
|
Output:
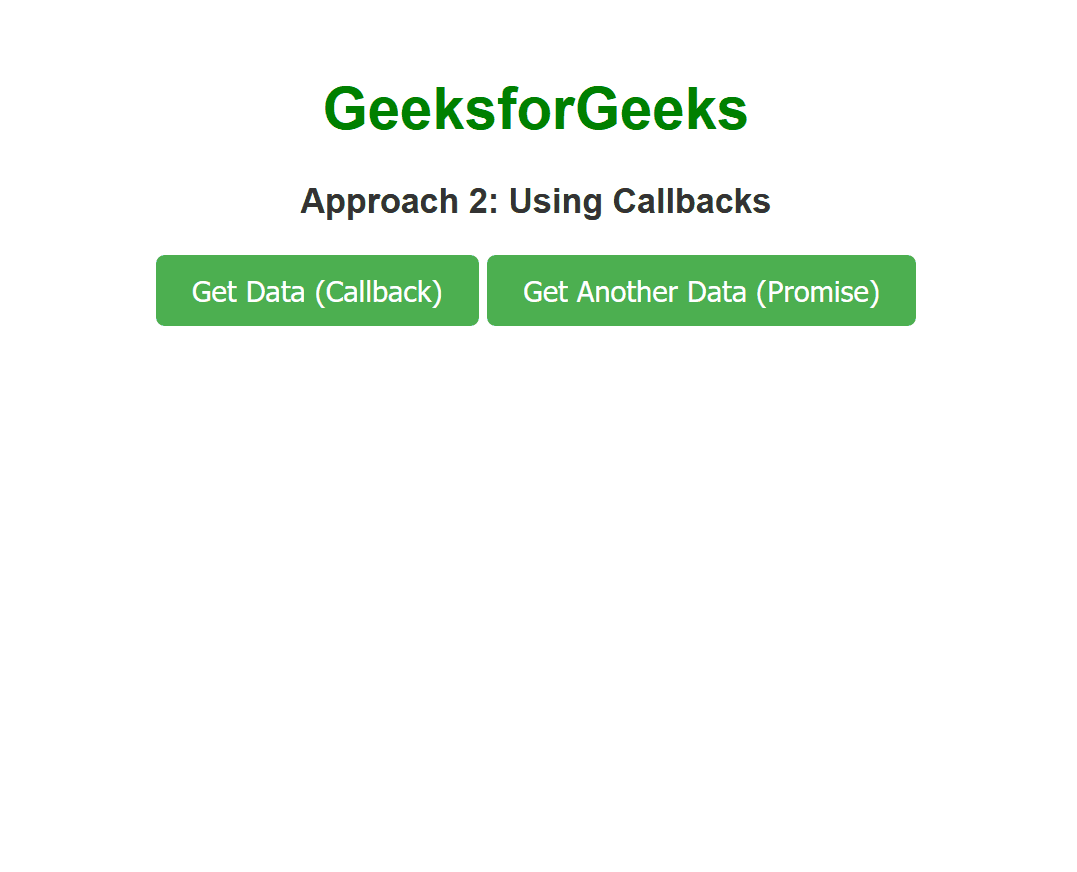
Share your thoughts in the comments
Please Login to comment...