How to Access EJS Variable in Javascript Logic ?
Last Updated :
08 Apr, 2024
EJS stands for Embedded JavaScript. It is a templating language used to generate dynamic HTML pages with data from the server by embedding JavaScript code.
Features of EJS
- Dynamic Content: Based on data from servers or other sources, we can generate a dynamic HTML template.
- Partial Templates: Partials are reusable chunks of HTML markup that can be included in multiple templates. We can create header.js and footer.js partials that can be added by all the templates (home.js, contact. js). This ensures code reusability.
- Control Structures: We can use loops, conditions, and iterations over an array/object to build complex templates.
- Embedded JavaScript : Using special tags ( <% %>, <%= %>, and <%- %> ) , we can embed JavaScript in HTML markup. EJS replaces <%= %> tags, with the actual value of the variable when rendering the page.
Steps to Access EJS variable in Javascript logic
Step 1: Firstly, we will make the folder named root by using the below command in the VScode Terminal. After creation use the cd command to navigate to the newly created folder.
mkdir root
cd root
Step 2: Once the folder is been created, we will initialize NPM using the below command, this will give us the package.json file.
npm init -y
Step 3: Once the project is been initialized, we need to install Express and EJS dependencies in our project by using the below installation command of NPM.
npm i express ejs
Project Structure
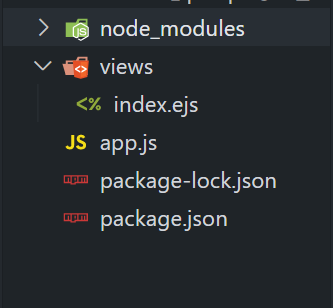
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"ejs": "^3.1.9",
}
Explanation:
- Here, we have used special tags like <%= %> and <% %> to access EJS variables in JS.
- We are using <% %> for adding control strcture( like here we used if-else within <% %> within HTML tags.
- Here, we are accessing the variable role within if condition and since <% %> are already added , we can directly access role variable.
- To print value of name , we are using <%= %> tag (<%=name%>) .
- We are accessing EJS varible within script tag by using <%= %> tag ( <%=role%> ), saving it in role variable and then using its value.
- <%=name%> and <%=role%> will be replaced with value of name and role variable respectively when the template index.ejs is rendered.
Example: Below is an example of Accessing EJS variable in Javascript logic.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Access EJS Variable in JS</title>
</head>
<body>
<h1>Hello,
<%if(role=='Admin'){%>
<%= name %>
<%}else{%>
Guest
<%}%>
!
</h1>
<script>
var role = '<%= role %>';
if (role === 'Admin') {
alert('Welcome to GFG, Admin!');
} else {
alert('Welcome to GFG, Guest!');
}
</script>
</body>
</html>
JavaScript
// app.js
const express = require('express');
const path = require('path');
const app = express();
const PORT = process.env.PORT || 4000;
// Set the view engine to EJS
app.set('view engine', 'ejs');
// Define a route to render the HTML file
app.get('/', (req, res) => {
res.render('index', { role: 'Admin', name: 'GFG' });
});
// Start the server
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Output:
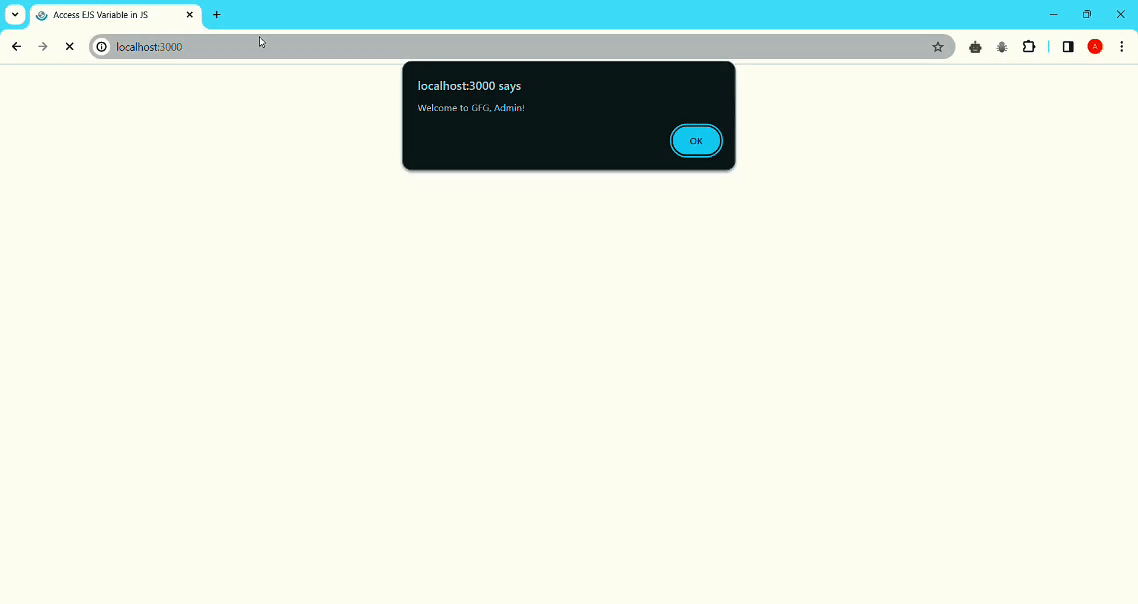
Share your thoughts in the comments
Please Login to comment...