How do you navigate programmatically in Angular?
Last Updated :
16 Apr, 2024
Angular is a popular framework for building dynamic web applications, and one of its important features is its routing system. In Angular, we use a Router module, which allows us to navigate between different components easily. While navigation often occurs as a result of certain user actions like clicking on a link, there are also several use cases where we need to navigate programmatically. In this article, we’ll look at the way to navigate programmatically in Angular.
Steps to Set Up Angular Application
Step 1: Create an Angular application using the following command.
ng new my-app
Step 2: After creating the project, navigate to the project folder using the below command.
cd my-app
Example 1: Programmatic Navigation Triggered by User Interaction
In this example, we’ll see how we can navigate from one component to another programmatically by handling user click.
Step 1: Generate a target component and a source component for navigation purpose using the below commands.
ng generate component target
ng generate component source
Folder Structure:

project structure
Code Example:
HTML
<!-- target.component.html -->
<h1>This is the target component!</h1>
HTML
<!-- source.component.html -->
<h1>I'm the source component</h1>
<button (click)="onButtonClick()">Go to Target Route</button>
HTML
<!-- app.component.html -->
<router-outlet></router-outlet>
JavaScript
//app.routes.ts
import { Routes } from '@angular/router';
import { TargetComponent } from './target/target.component';
import { AppComponent } from './app.component';
import { SourceComponent } from './source/source.component';
export const routes: Routes = [
{ path: '', component: SourceComponent },
{ path: 'target', component: TargetComponent },
];
JavaScript
//source.component.ts
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'app-source',
standalone: true,
imports: [],
templateUrl: './source.component.html',
styleUrl: './source.component.css',
})
export class SourceComponent {
constructor(private router: Router) { }
onButtonClick() {
this.router.navigate(['/target']);
}
}
Step 2: Apply the changes and run the application by running the following command.
ng serve
Output:
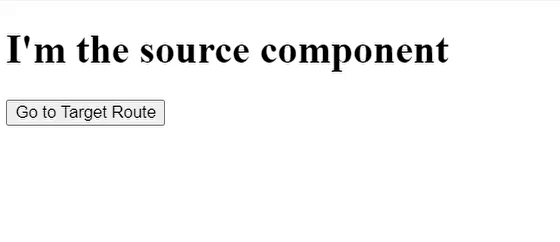
output for example 1
Example 2: Programmatic Navigation with Route Parameters
In this example, we’ll navigate programmatically to another route with a route parameter when a button is clicked. It is beneficial whenever we need to pass a data along with the route.
Step 1: Update the Source Component HTML template by writing template code in source.component.html. Here we’ll pass the data to the handler function.
HTML
<!-- source.component.html -->
<h1>I'm the source component</h1>
<button (click)="onClick('user1')">Visit Target Page</button>
HTML
<!-- target.component.html -->
<h1>Hello {{username}}</h1>
<h3>This is the target component</h3>
JavaScript
//target.component.ts
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-target',
standalone: true,
imports: [],
templateUrl: './target.component.html',
styleUrl: './target.component.css',
})
export class TargetComponent implements OnInit {
username: string = '';
constructor(private route: ActivatedRoute) { }
ngOnInit() {
this.route.params.subscribe((params) => {
this.username = params['username'];
});
}
}
JavaScript
//source.component.ts
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'app-source',
standalone: true,
imports: [],
templateUrl: './source.component.html',
styleUrl: './source.component.css',
})
export class SourceComponent {
constructor(private router: Router) { }
onClick(user1: string) {
this.router.navigate(['/target', user1]);
}
}
JavaScript
//app.routes.ts
import { Routes } from '@angular/router';
import { TargetComponent } from './target/target.component';
import { AppComponent } from './app.component';
import { SourceComponent } from './source/source.component';
export const routes: Routes = [
{ path: '', component: SourceComponent },
{ path: 'target/:username', component: TargetComponent },
];
Step 2: Apply the changes and run the application using the following command.
ng serve
Output:
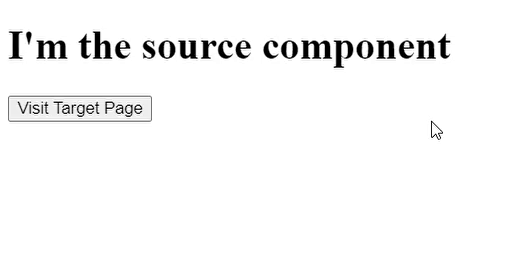
output for example 2
Share your thoughts in the comments
Please Login to comment...