Get OSM Features Within a Distance of Address Using OSMnx Feature Module
Last Updated :
08 Apr, 2024
OpenStreetMap provides physical features on the ground (e.g ., roads, buildings, etc.) using tags attached to its basic data structure (nodes, ways, and relations). Each tag describes a geographic attribute of the feature for the specific node, way, or relation. In this article, we will see how we can get OSM features within a distance of address using the OSMnx feature module in Python.
Syntax of osmnx.features.features_from_address() Function
The function creates a GeoDataFrame of OSM features within some distance of the address N, S, E, W. Below is the syntax:
osmnx.features.features_from_address(address, tags, dist=1000)
Parameters:
- address (string): Address to geocode and use as the central point to get the features
- tags (dict): Dict of tags used for finding elements in the selected area. The results returned are the union of each tag. The dict keys should be OSM tags, (e.g., building, landuse, highway, etc) and the dict values should be either True to retrieve all items with the given tag, or a string to get a single tag-value combination, or a list of strings to get multiple values for the given tag.
- dist (numeric): distance in meters
Returns: gdf
Return Type: geopandas.GeoDataFrame
OSM Features Within a Distance of Address Using OSMnx Feature Module
Below are some approaches by which we can find OSM features within a distance of address using OSMnx feature module in Python:
Find the Building Details From the Open Street Map
In this step, we are finding the building details within 1000 m of a place. In the below code, we set the ‘building’ tag to true to list the available buildings within 1000m of SoHo, NewYork
Python3
import osmnx as ox
# get building details from address within 1000 m
place = "SoHo, New York, NY"
gdf = ox.features.features_from_address(
place, {'building': True}, dist=1000)
# print first 5 building details
gdf.head(5)
Output
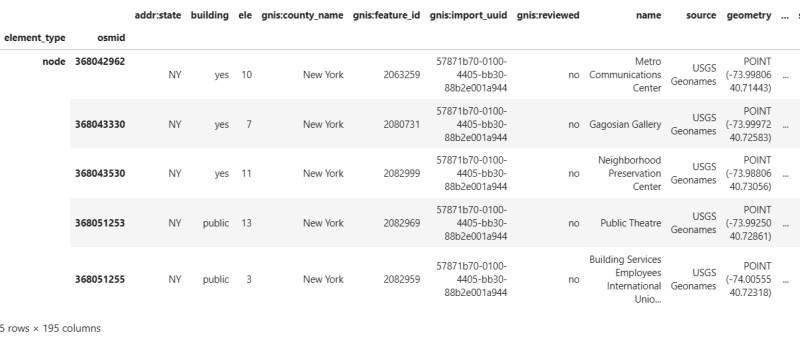
building details
We can list the available columns in our geodataframe using info() method.
Python3
Output
<class 'geopandas.geodataframe.GeoDataFrame'>
MultiIndex: 7268 entries, ('node', 4710026885) to ('relation', 1651490)
Data columns (total 99 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 alt_name:en 1 non-null object
1 alt_name:ml 2 non-null object
2 name 308 non-null object
3 name:ml 19 non-null object
4 geometry 7268 non-null geometry
5 building 7268 non-null object
6 addr:district 2 non-null object
7 addr:place 1 non-null object
8 addr:state 1 non-null object
9 amenity 101 non-null object
10 denomination 2 non-null object
11 female 1 non-null object
12 opening_hours 12 non-null object
13 religion 31 non-null object
14 service_times 2 non-null object
15 wheelchair 5 non-null object
16 name:en 6 non-null object
17 survey:date 1 non-null object
18 tourism 27 non-null object
19 addr:city 148 non-null object
20 nodes 7258 non-null object
21 covered 1 non-null object
22 shelter_type 1 non-null object
23 wikidata 3 non-null object
24 building:levels 81 non-null object
25 Administration 1 non-null object
26 layer 20 non-null object
27 level 1 non-null object
28 operator 8 non-null object
29 building:part 2 non-null object
30 name:mr 1 non-null object
31 castle_type 6 non-null object
32 historic 6 non-null object
33 leisure 1 non-null object
34 shop 83 non-null object
35 source 6035 non-null object
36 subdenomination 1 non-null object
37 payment:cash 6 non-null object
38 payment:credit_cards 3 non-null object
39 addr:postcode 51 non-null object
40 addr:street 145 non-null object
41 architect 1 non-null object
42 architect:wikidata 1 non-null object
43 architect:wikipedia 1 non-null object
44 brand 26 non-null object
45 brand:wikidata 26 non-null object
46 brand:wikipedia 22 non-null object
47 cuisine 7 non-null object
48 outdoor_seating 1 non-null object
49 smoking 2 non-null object
50 addr:housenumber 5 non-null object
51 office 35 non-null object
52 int_name 1 non-null object
53 railway 1 non-null object
54 height 64 non-null object
55 internet_access 8 non-null object
56 internet_access:fee 3 non-null object
57 phone 8 non-null object
58 stars 2 non-null object
59 website 6 non-null object
60 area 2 non-null object
61 landuse 1 non-null object
62 operator:wikidata 1 non-null object
63 atm 4 non-null object
64 drive_through 2 non-null object
65 short_name 6 non-null object
66 screen 3 non-null object
67 air_conditioning 2 non-null object
68 payment:debit_cards 1 non-null object
69 branch 3 non-null object
70 healthcare 2 non-null object
71 man_made 1 non-null object
72 fee 2 non-null object
73 museum 2 non-null object
74 name:etymology:wikidata 2 non-null object
75 check_date 1 non-null object
76 building:material 4 non-null object
77 description 2 non-null object
78 opening_hours:covid19 1 non-null object
79 rooms 2 non-null object
80 brand:ks 1 non-null object
81 brand:pa 1 non-null object
82 brand:ur 1 non-null object
83 name:ks 1 non-null object
84 name:pa 1 non-null object
85 name:ur 1 non-null object
86 takeaway 1 non-null object
87 alt_name 1 non-null object
88 wikipedia 1 non-null object
89 government 2 non-null object
90 guest_house 1 non-null object
91 craft 2 non-null object
92 clothes 1 non-null object
93 healthcare:speciality 1 non-null object
94 operator:type 1 non-null object
95 ref 1 non-null object
96 repair 1 non-null object
97 ways 1 non-null object
98 type 1 non-null object
dtypes: geometry(1), object(98)
memory usage: 5.8+ MB
Plotting the OSM Features Using plot_footprints() Method
In this step, we will plot the OSM features using plot_footprints() method by passing the geodataframe inside it.
Python3
fig, ax = ox.plot_footprints(gdf, figsize=(3, 3))
Output
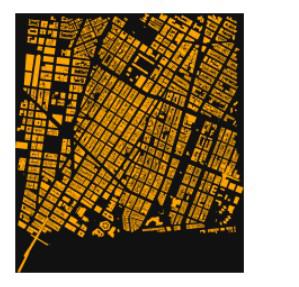
building plot
We can plot it on a map by using the explore() method from geodataframe.
Python3
Output
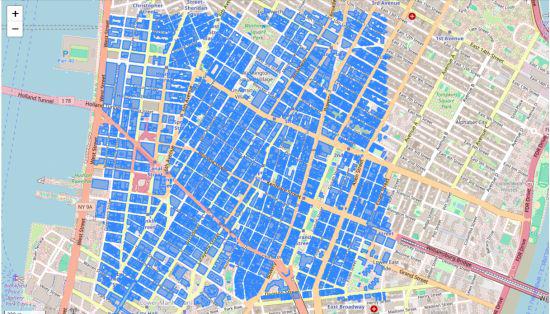
building feature on map
Find the Emergency Support System Details from the Open Street Map
We can find the emergency support system details like hospitals, ambulances, etc. by setting the ’emergency’ tag as true and OSMnx will lists all the available emergency support system.
Python3
import osmnx as ox
place = "Thiruvananthapuram, Kerala"
gdf = ox.features.features_from_address(
place, {'emergency': True}, dist=3000)
print(gdf)
Output
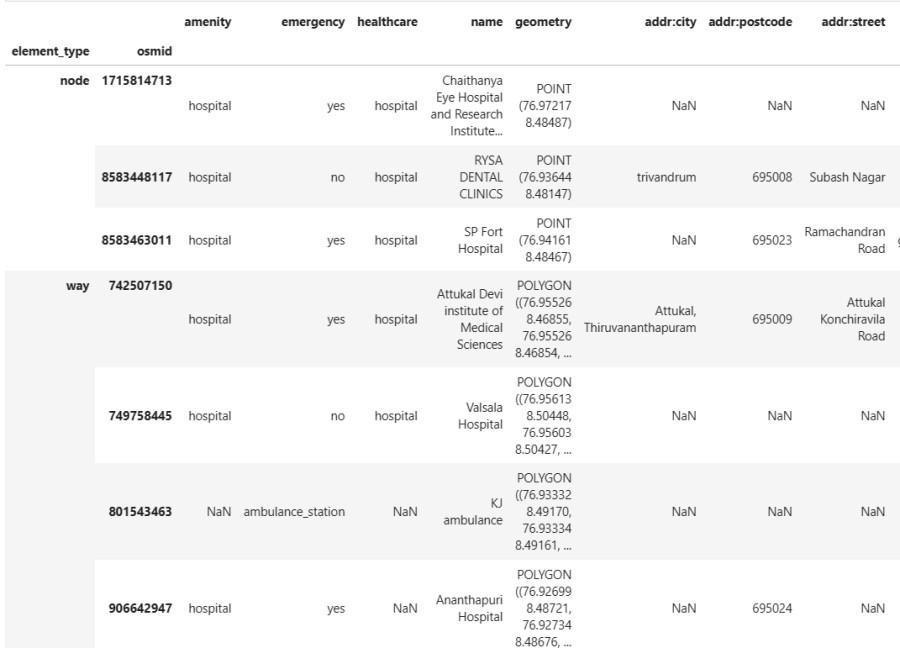
emergency as tag
Get Multiple Map Features from Open Street Map
Let’s try multiple tags. We can try the below tag
tags = {‘amenity’:True,
‘landuse’:[‘retail’,’commercial’],
‘highway’:’bus_stop’}
In the below code, it lists the entire amenity available since we set ‘amenity’ tag as true; In case of ‘landuse’ tag, OSMnx fetches details based on ‘retail’ and ‘commercial’ subtags. Similary the ‘bus_stop’ subtag details from ‘highway’ tag.
Python3
import osmnx as ox
tags = {'amenity': True,
'landuse': ['retail', 'commercial'],
'highway': 'bus_stop'}
# fetch multiple features
gdf = ox.features.features_from_address(
"Thiruvananthapuram, Kerala", tags, dist=500)
# display it on map
gdf.explore()
Output
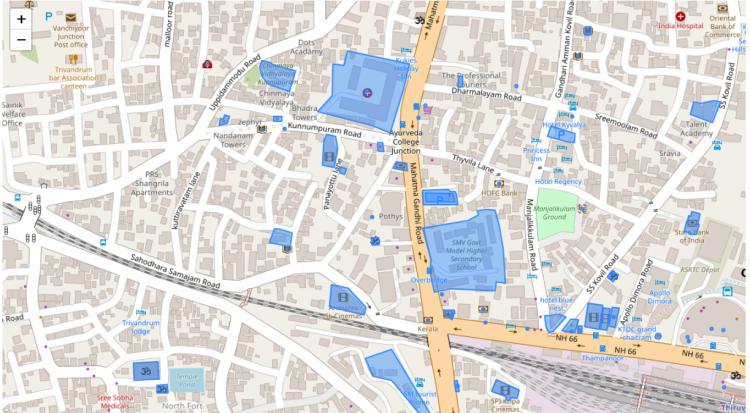
Multiple Features
Share your thoughts in the comments
Please Login to comment...