Fonts in Next JS
Last Updated :
20 Feb, 2024
Fonts are used to style the components and to increase the readability of the application. The font is associated with the style, size, width, and typeface i.e. design of the letters. We can customize the font according to our choice by simply adding them to the global CSS file and in the head section of the application file. Using this approach, the request is made every single time of use. A better approach is using next/font.
Next.js allows you to automatically self-host the font on the Next.js deployment server. Fonts are downloaded at build time and host them. next/font helps fonts to load with zero layout shift.
Syntax:
import { font_name} from next/font
Prerequisites:
Steps to create the Next JS Application:
Step 1: To create your app, run the following npx command in your terminal to start the creation process:
npx create-next-app@latest Demo-App
Step 2: Provide the necessary details to create your app as shown in the image below.
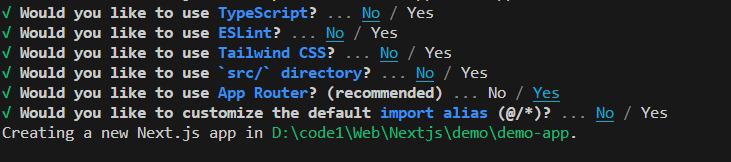
Steps to create the project:
Step 3: Install the next/font
npm install @next/font
Project Structure:
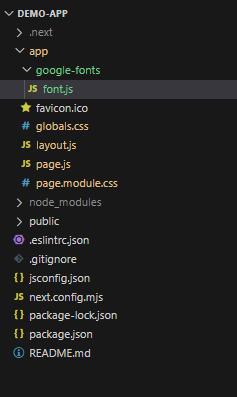
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"@next/font": "^14.1.0",
"next": "14.1.0",
"react": "^18",
"react-dom": "^18"
}
Step 4: To start the application run the following command:
npm run dev
Google Fonts
Next JS allows us to use all the google font in the application.They are automatically self hosted and no request is sent form the browser while running the application.You need to import the font from the next/font/google and set the object with the characteristics of the font. One can import multiple fonts at the same time .
Example 1: Illustration of using Google Fonts through importing font from next/font/google
Javascript
import { Rochester } from "next/font/google" ;
import "./globals.css" ;
const rock = Rochester({ subsets: [ "latin" ], weight: '400' });
export const metadata = {
title: "Create Next App" ,
description: "Generated by create next app" ,
};
export default function RootLayout({ children }) {
return (
<html lang= "en" >
<body className={rock.className}>{children}</body>
</html>
);
}
|
Javascript
import styles from "./page.module.css" ;
export default function Home() {
return (
<main className={styles.main}>
<h1>React Tutorial</h1>
<p>React is one of the most popular, efficient, and powerful open-source JavaScript library for
building dynamic and interactive user interfaces. Whether you are a beginner or an experienced
developer, React Tutorial will significantly enhance your development skills. React JS is not a
framework, it is just a library developed by Facebook.</p>
<h3>ReactJS Advantages</h3>
<ul>
<li>Composable: We can divide these codes and put them in custom components. Then we can utilize
those components and integrate them into one place.</li>
<li>Declarative: In ReactJS, the DOM is declarative. We can make interactive UIs by changing
the state of the component and ReactJS takes care of updating the DOM according to it.</li>
<li>Community: ReactJS has a huge community because of it’s demand each company wants to work
with ReactJS. Companies like Meta, Netflix, etc built on ReactJS.</li>
<li>SEO Friendly: ReactJS affects the SEO by giving you a SPA (Single Page Application) which
requires Javascript to show the content on the page which can be rendered and indexed.</li>
</ul>
</main>
);
}
|
CSS
.main {
display : flex;
flex- direction : column;
justify- content : space-between;
align-items: center ;
padding : 6 rem;
min-height : 100 vh;
}
|
CSS
* {
box-sizing: border-box;
padding : 0 ;
margin : 0 ;
}
html,
body {
max-width : 100 vw;
overflow-x: hidden ;
line-height : 1.5 ;
}
li {
padding : 0.5 rem;
}
|
- In the layout.js, Rochester font is imported from the Google Fonts.
- const rock specify the subset of the font and the weight for the Rochester font.
- In page.js ,styles are imported from “./page.module.css” which contains the styling of the components in page.js file.
- Global Styles used for the application are specified in globals.css
Output:
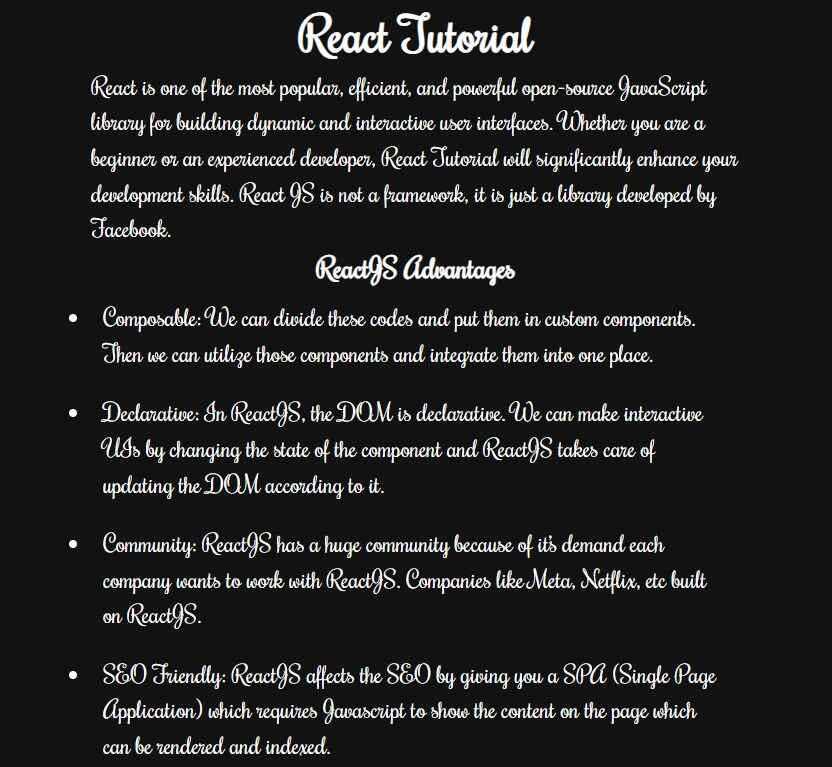
Google Font
Example 2: Using Font in CSS File.
- Rochester font is imported from “next/font/google” in font.js
- const rock specify the subset of the font and the weight for the Rochester font.It is exported and used in layout.js
- In the layout.js ,font is imported as used through rock.variable.
Javascript
import { Rochester } from "next/font/google" ;
export const rock = Rochester({
weight: '400' ,
subsets: [ 'latin' ],
variable: '--rochester-font'
})
|
Javascript
import { rock } from "./google-fonts/font" ;
import "./globals.css" ;
export const metadata = {
title: "Create Next App" ,
description: "Generated by create next app" ,
};
export default function RootLayout({ children }) {
return (
<html lang= "en" >
<body className={rock.variable}>{children}</body>
</html>
);
}
|
CSS
html,
body {
max-width : 100 vw;
overflow-x: hidden ;
line-height : 1.5 ;
font-family : var(--rochester-font);
}
li {
padding : 0.5 rem;
}
|
Output:
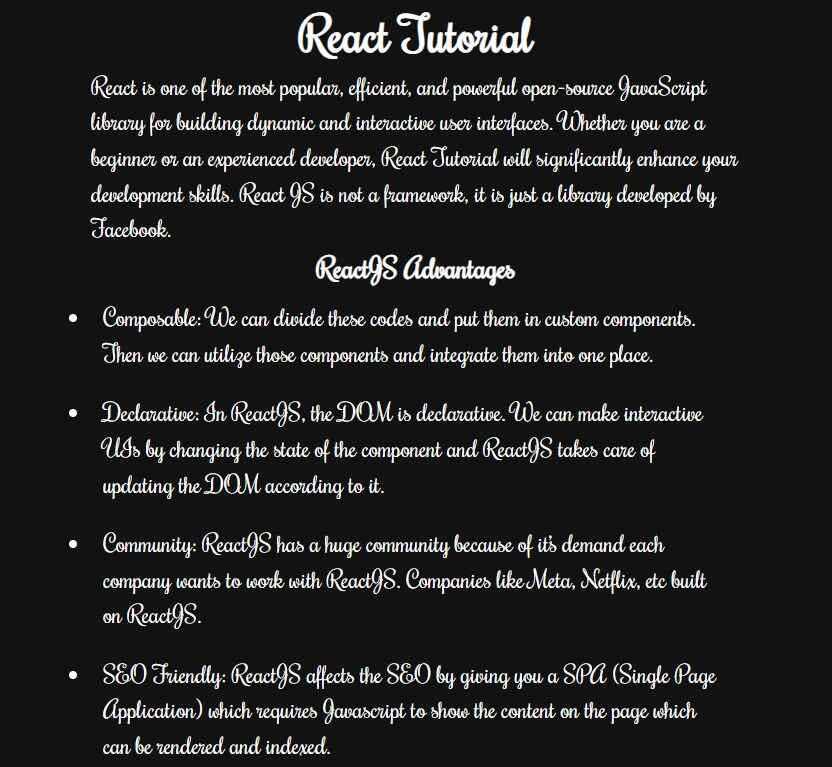
Example 3: Using Embedded Code form Google Font.
- page.js contains the Head component .It is in inbuilt component, embedded code of the Kode Mono font is added in it from the Google Font website.
- In page.js ,styles are imported from “./page.module.css” which contains the styling of the components in page.js file.
- Global Styles used for the application are specified in globals.css
Javascript
import Head from "next/head" ;
import styles from "./page.modules.css" ;
export default function Home() {
return (
<>
<Head>
<title>Font</title>
<meta name= "description" content= "Generated by create next app" />
<meta name= "viewport" content= "width=device-width, initial-scale=1" />
<link rel= "icon" href= "/favicon.ico" />
<link href=
wght@0,100;0,300;0,400;
0,500;0,700;0,900;1,100;1,300;1,400;1,500;1,700;1,900&display=swap" rel= "stylesheet" >
</link>
</Head>
<main className={`${styles.main} `}>
<h2>Next JS </h2>
<p>
Next.js is an open-source web development React-based framework
created by Vercel.Next.js application and is used to create
production-ready web applications.It has features like hot
code reloading, data fetching utilities, dynamic API routes,
optimized builds, etc.
</p>
<h2>React JS</h2>
<p>
React is one of the most popular, efficient,
and powerful open-source JavaScript library for
building dynamic and interactive user
interfaces.
</p>
</main>
</>
);
}
|
CSS
.main {
display : flex;
flex- direction : column;
align-items: center ;
padding : 4 rem;
min-height : 100 vh;
}
|
CSS
:root {
--font- mono : 'Kode Mono' ;
}
* {
box-sizing: border-box;
padding : 0 ;
margin : 0 ;
}
html,
body {
max-width : 100 vw;
overflow-x: hidden ;
}
h 2 ,
p {
text-align : justify ;
color : rgb ( 255 , 0 , 255 );
padding : 1 rem 1.2 rem;
font-family : var(--font- mono );
}
|
Output:
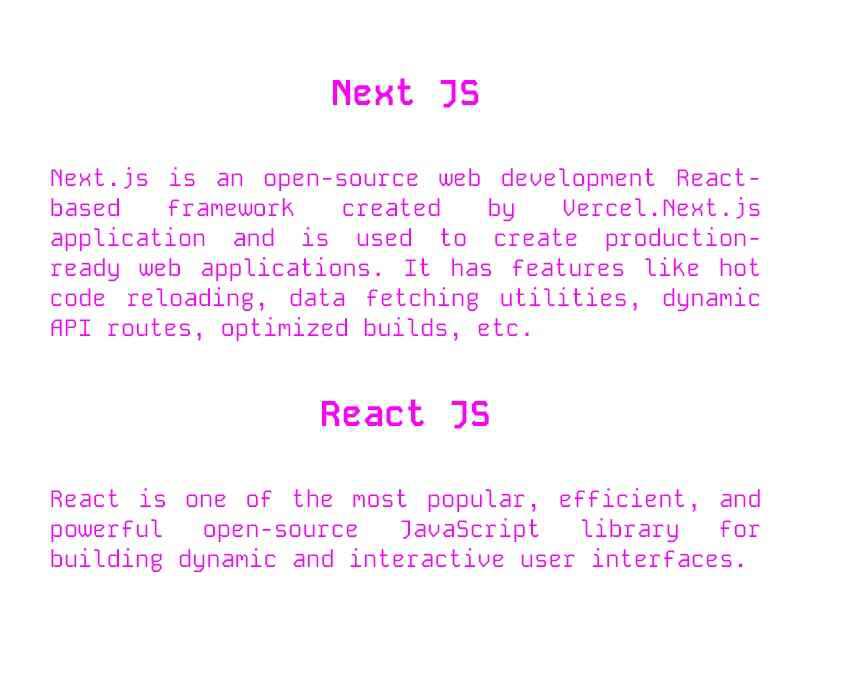
Font NextJS
Share your thoughts in the comments
Please Login to comment...