Flutter – ImageFiltered Widget
Last Updated :
31 Jul, 2023
In Flutter it’s straightforward to blur the background image using Image Filtered Widget. A filter operation is used to scan the image. ImageFiltered is a widget that applies ImageFilter to its children to blur the image.
ImageFilter Constructor
const ImageFiltered({
Key? key,
required this.imageFilter,
Widget? child,
})
ImageFilter
This is a required property to give ImageFilter.blur to blur the background image with Sigma X & Sigma Y axis. Now let’s see how to blur the background image as you can see below picture.
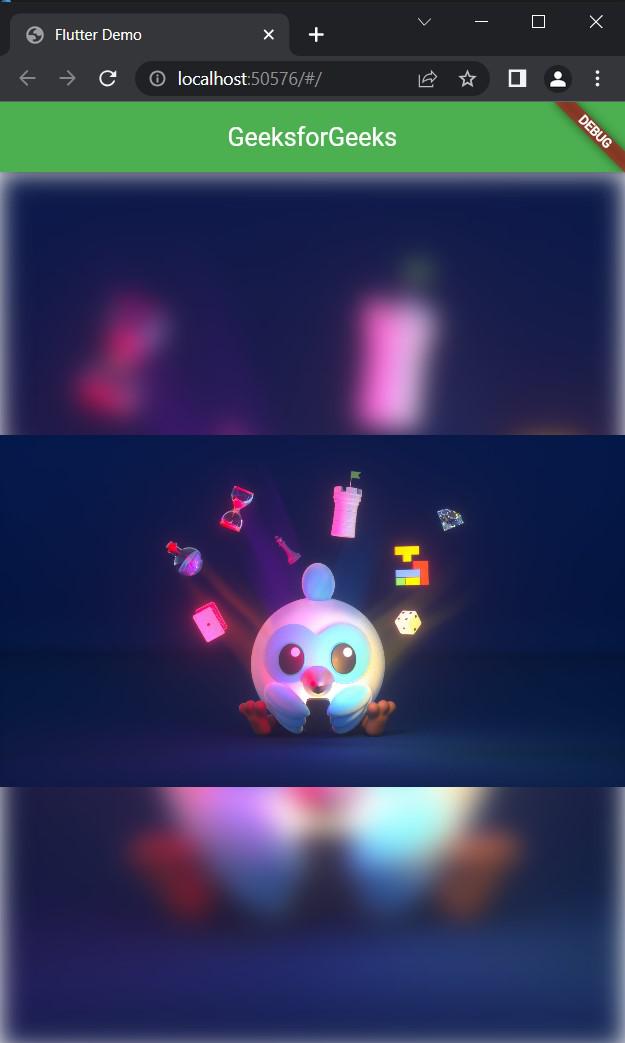
Implementation
Step 1: Add Appbar
Dart
import 'dart:ui' ;
import 'package:flutter/material.dart' ;
void main() {
runApp( const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo' ,
theme: ThemeData(
primarySwatch: Colors.green,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text( 'GeeksforGeeks' ),
centerTitle: true ,
),
);
}
}
|
Output:
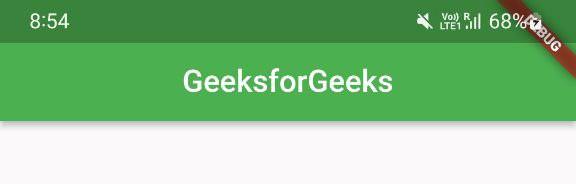
Step 2: Use Network Image to get images from the internet
Dart
import 'dart:ui' ;
import 'package:flutter/material.dart' ;
void main() {
runApp( const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo' ,
theme: ThemeData(
primarySwatch: Colors.green,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text( 'GeeksforGeeks' ),
centerTitle: true ,
),
body: Stack(
alignment: Alignment.center,
children: [
Image.network(
],
),
);
}
}
|
Output:
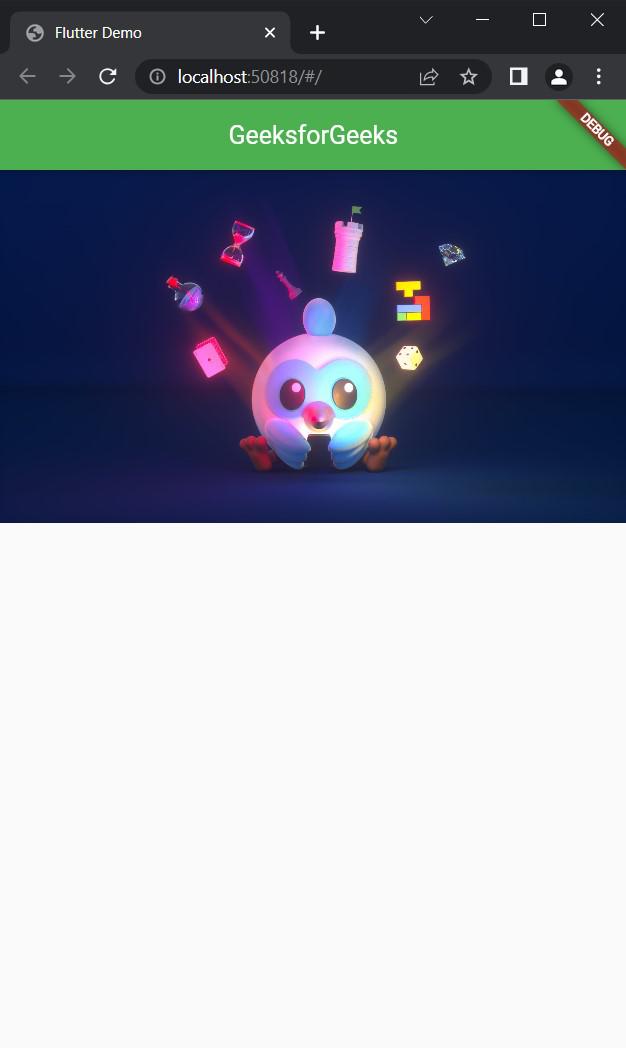
Step 3: Copy the same Network image and use the fit property and sized box property to expand the image in the background.
Dart
import 'dart:ui' ;
import 'package:flutter/material.dart' ;
void main() {
runApp( const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo' ,
theme: ThemeData(
primarySwatch: Colors.green,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text( 'GeeksforGeeks' ),
centerTitle: true ,
),
body: Stack(
alignment: Alignment.center,
children: [
SizedBox.expand(
child: Image.network(
fit: BoxFit.fitHeight,
),
),
Image.network(
],
),
);
}
}
|
Output:
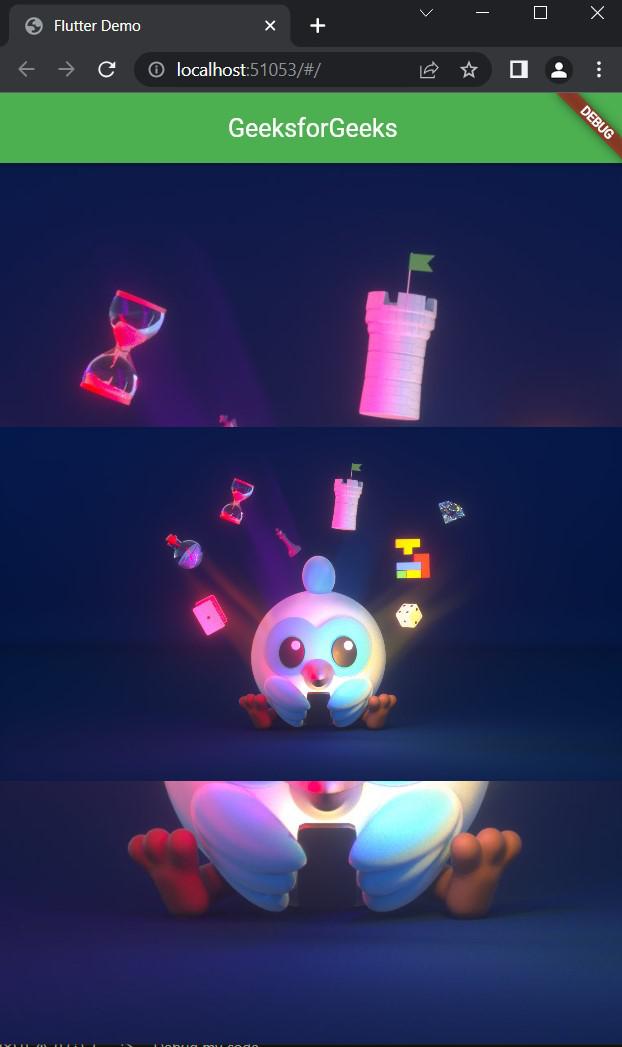
Step 4: Using the ImageFiltered widget
To blur the background image we use imagefilter with blur property which has 2 required properties SigmaX and SigmaY. By giving the value of SigmaX and SigmaY we decide how much blur we want.
ImageFilter.Blur Constructor:
ImageFilter.blur(
double sigmaX = 0.0,
double sigmaY = 0.0
TileMode tileMode = TileMode.clamp
})
Dart
import 'dart:ui' ;
import 'package:flutter/material.dart' ;
void main() {
runApp( const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo' ,
theme: ThemeData(
primarySwatch: Colors.green,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text( 'GeeksforGeeks' ),
centerTitle: true ,
),
body: Stack(
alignment: Alignment.center,
children: [
SizedBox.expand(
child: ImageFiltered(
imageFilter: ImageFilter.blur(
sigmaX: 10,
sigmaY: 10,
),
child: Image.network(
fit: BoxFit.fitHeight,
),
)),
Image.network(
],
),
);
}
}
|
Output:
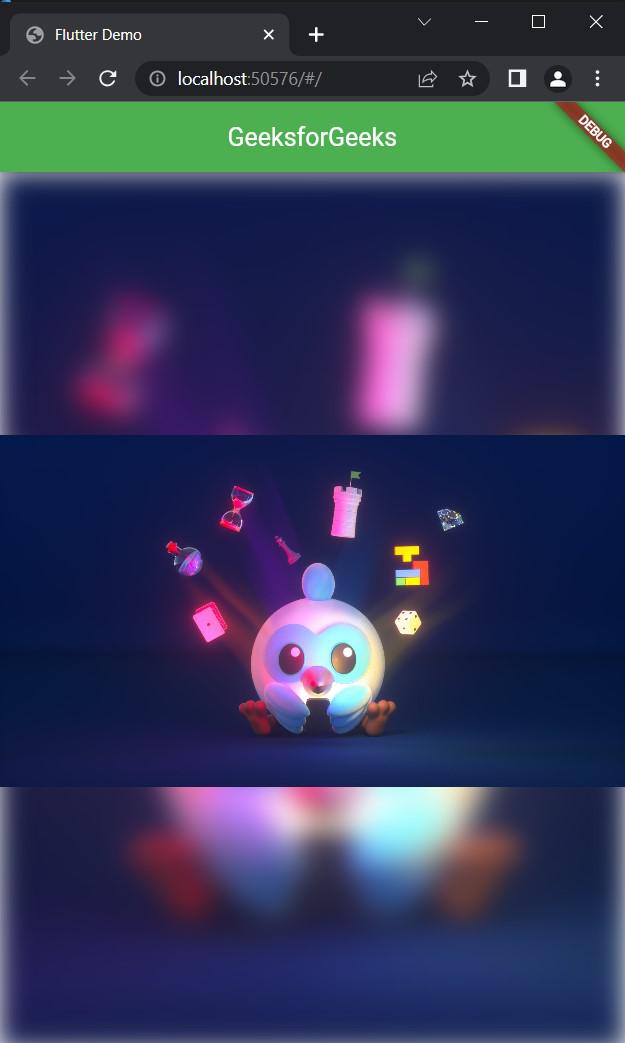
Share your thoughts in the comments
Please Login to comment...