Flutter – BackDropFilter Widget
Last Updated :
31 Jul, 2023
In this article, we will be going to learn about BackDrop Filter Widget in Flutter and see how to implement it in Flutter. BackDrop Filter widget that applies a filter to the existing painted content to make it blur so as to show the clear text on the screen. The filter will be applied to all the areas within its parent or ancestor widget’s clip. If there’s no clip, the filter will be applied to the full screen.
Constructor
Dart
BackdropFilter({
Key? key,
required ImageFilter filter,
Widget? child,
BlendMode blendMode = BlendMode.srcOver
})
|
Properties
- ImageFilter – This is a required property. The image filter is applied to the existing painted content before painting the child.
- Key – Controls how one widget replaces another widget in the tree.
Implementation
In this example, we will see how to make the image blur so we can see the text clearly.
Sample Image:
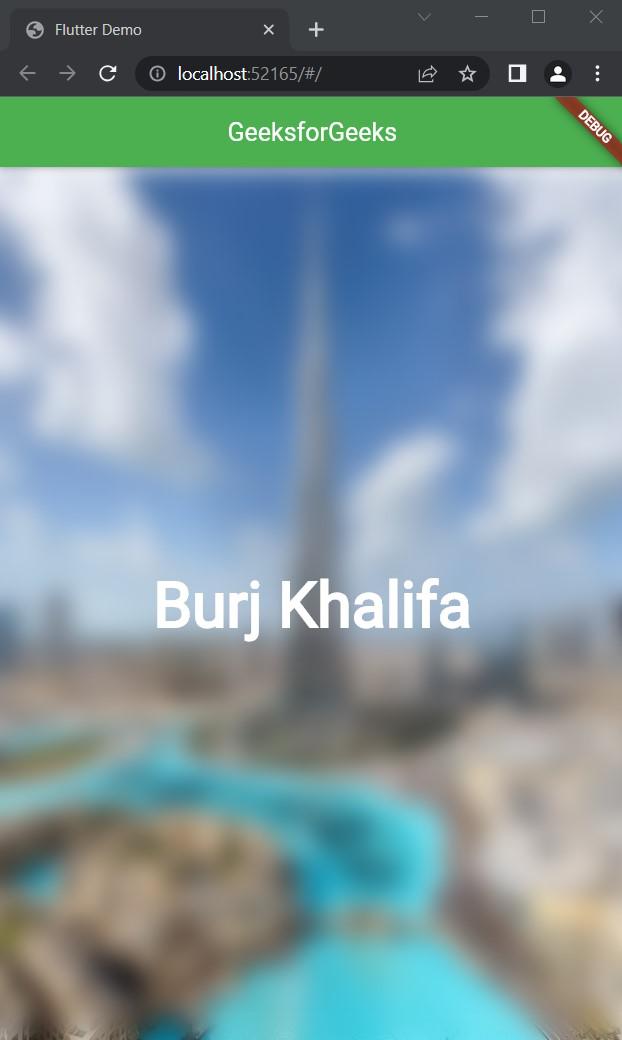
Step 1: Create an Appbar
Dart
import 'dart:ui' ;
import 'package:flutter/material.dart' ;
void main() {
runApp( const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo' ,
theme: ThemeData(
primarySwatch: Colors.green,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text( 'GeeksforGeeks' ),
centerTitle: true ,
),
);
}
}
|
Output:
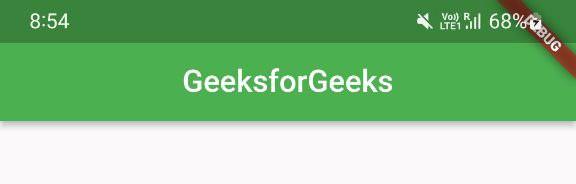
Step 2: Use Network Image to get images from the internet
Dart
import 'dart:ui' ;
import 'package:flutter/material.dart' ;
void main() {
runApp( const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo' ,
theme: ThemeData(
primarySwatch: Colors.green,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text( "GeeksforGeeks" ), centerTitle: true ),
body: Stack(
fit: StackFit.expand,
children: [
Image.network(
fit: BoxFit.cover,
),
],
),
);
}
}
|
Output:
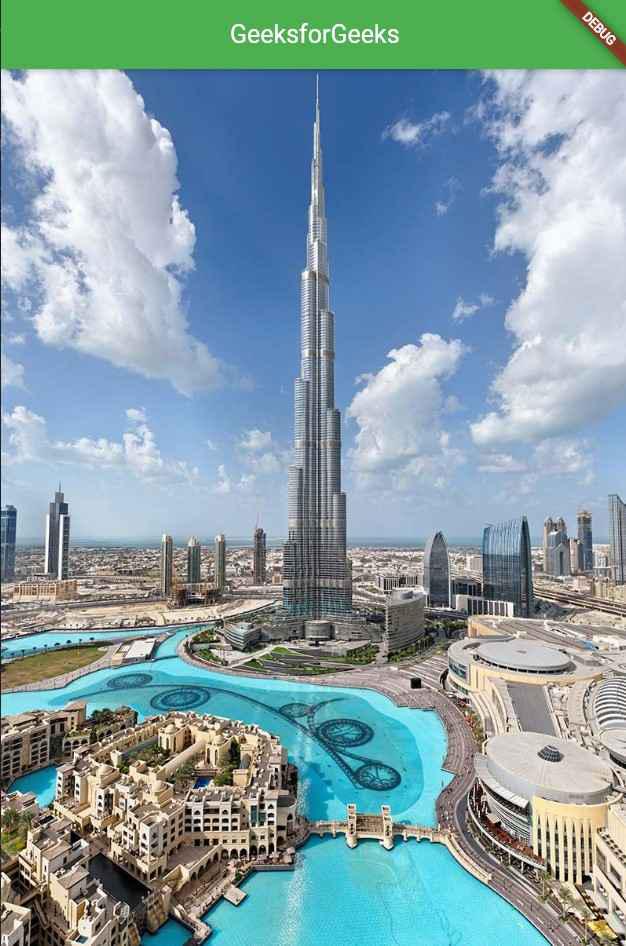
Step 3: Use a container and add text to it and give text style to it
Dart
import 'dart:ui' ;
import 'package:flutter/material.dart' ;
void main() {
runApp( const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo' ,
theme: ThemeData(
primarySwatch: Colors.green,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text( "GeeksforGeeks" ), centerTitle: true ),
body: Stack(
fit: StackFit.expand,
children: [
Image.network(
fit: BoxFit.cover,
),
Container(
alignment: Alignment.center,
child: Text(
'Burj Khalifa' ,
style: TextStyle(
fontSize: 50,
fontWeight: FontWeight.bold,
color: Colors.white),
),
)
],
),
);
}
}
|
Output: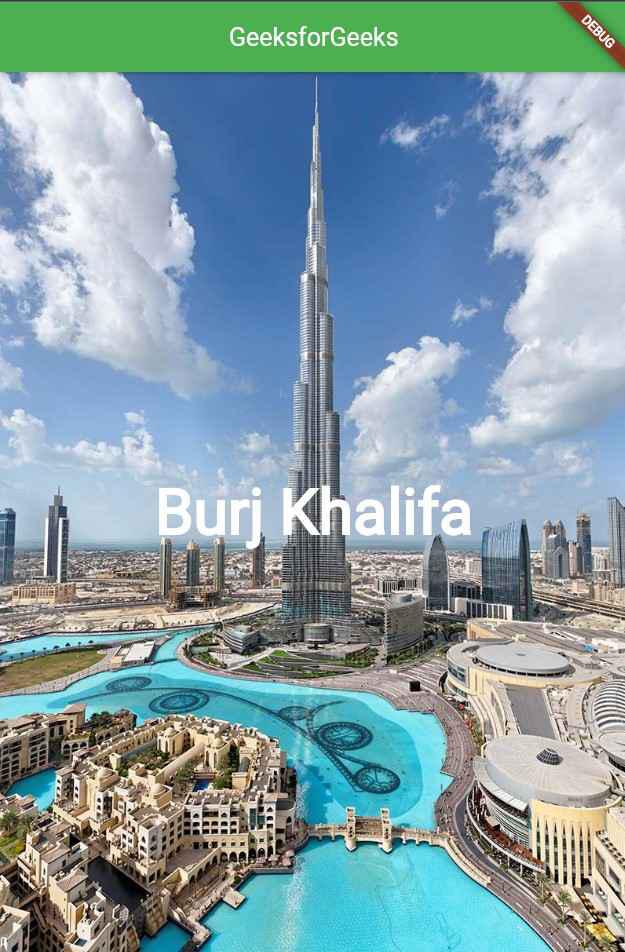
Step 4:
Wrap the container with BackDropFilter Widget and provide the required properties of SigmaX and SigmaY to make the image blur accordingly then you will see the image blur and the text clearly.
Dart
import 'dart:ui' ;
import 'package:flutter/material.dart' ;
void main() {
runApp( const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo' ,
theme: ThemeData(
primarySwatch: Colors.green,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text( "GeeksforGeeks" ), centerTitle: true ),
body: Stack(
fit: StackFit.expand,
children: [
Image.network(
fit: BoxFit.cover,
),
BackdropFilter(
filter: ImageFilter.blur(sigmaY: 10, sigmaX: 10),
child: Container(
alignment: Alignment.center,
child: Text(
'Burj Khalifa' ,
style: TextStyle(
fontSize: 50,
fontWeight: FontWeight.bold,
color: Colors.white),
),
),
),
],
),
);
}
}
|
Output:
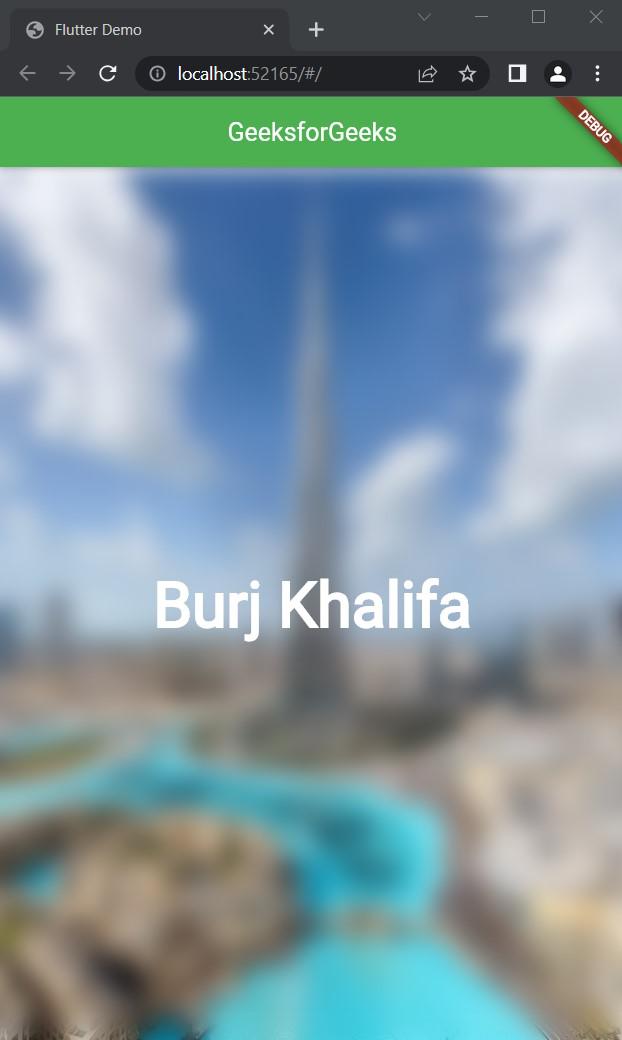
Share your thoughts in the comments
Please Login to comment...