Flutter – BoxShadow Widget
Last Updated :
02 Nov, 2023
BoxShadow is a built-in widget in flutter, whose functionality is to cast shadow to a box. The BoxShadow widget is usually used with BoxDecoration. In BoxDecoration widget one of its parameters is boxShadow which takes a list of BoxShadow to cast a shadow around a box.
Constructor of BoxShadow Class:
const BoxShadow(
{Color color: const Color(0xFF000000),
Offset offset: Offset.zero,
double blurRadius: 0.0,
double spreadRadius: 0.0}
)
Properties of BoxShadow Widget:
- blurRadius: This property takes in a double value as the object. It controls the haziness on the edges of the shadow.
- blurSigma: This property takes in a double value as the object. It controls the blur-radius in terms of sigma instead of logical pixels.
- color: The color property takes Color class as the object to decide the color of the shadow.
- offset: Offset class is the object given to this property which controls the extent to which the shadow will be visible.
- spreadRadius: This property also takes in a double value as the object to decide the extent to which the box should be inflated before applying the blur.
Example: Here we will see how to cast shadow to a box, by using the BoxShadow widget inside the BoxDecoration.
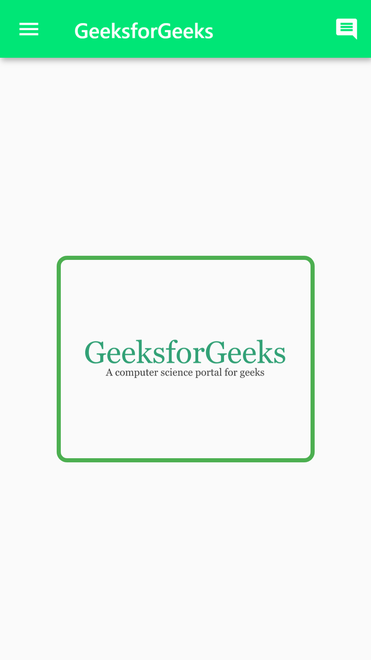
This is the BoxDecoration widget which is having an image and a border surrounding that. We will see how to apply shadow to this box.
main.dart
Dart
import 'package:flutter/material.dart' ;
void main() {
runApp(
MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text( 'GeeksforGeeks' ),
backgroundColor: Colors.greenAccent[400],
leading: IconButton(
icon: Icon(Icons.menu),
tooltip: 'Menu' ,
onPressed: () {},
),
actions: <Widget>[
IconButton(
icon: Icon(Icons.comment),
tooltip: 'Comment' ,
onPressed: () {},
),
],
),
body: Center(
child: Padding(
padding: const EdgeInsets.all(12.0),
child: SizedBox(
height: 200,
width: 250,
child: Container(
decoration: BoxDecoration(
image: const DecorationImage(
image: NetworkImage(
scale: 3.0,
),
border: Border.all(
color: Colors.green,
width: 4.0,
style: BorderStyle.solid),
borderRadius: BorderRadius.only(
topLeft: Radius.circular(10.0),
topRight: Radius.circular(10.0),
bottomLeft: Radius.circular(10.0),
bottomRight: Radius.circular(10.0),
),
boxShadow: [
BoxShadow(
color: Colors.greenAccent[200],
offset: const Offset(
5.0,
5.0,
),
blurRadius: 10.0,
spreadRadius: 2.0,
),
BoxShadow(
color: Colors.white,
offset: const Offset(0.0, 0.0),
blurRadius: 0.0,
spreadRadius: 0.0,
),
],
),
),
),
),
),
),
debugShowCheckedModeBanner: false ,
),
);
}
|
Output:
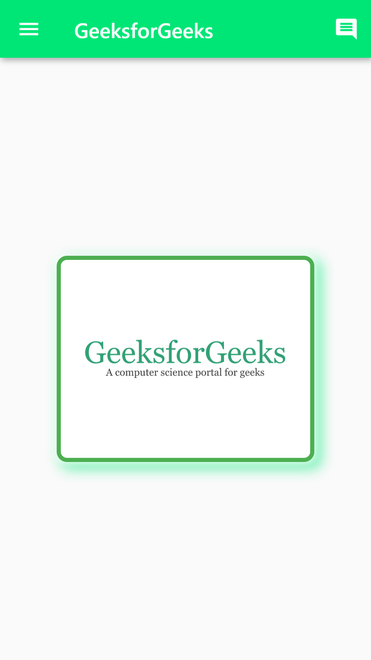
Explanation: As mentioned earlier the BoxDecoration widget have a parameter called boxShadow which takes in List<BoxShadow> (a list of BoxShadow widgets) as the object to cast a shadow to the box. The top-most widget in the list appears at the bottom most part of the app.
Now, taking a look at the code we can see that the first BoxShadow widget in the list is assigned a color of greenAccent[400], which is acting like the actual shadow of the box in the app. And then we have the offset which is set to 5.0 for both x-axis and y-axis, this is the distance to which the green shadow will be cast. Then we have the blur radius set to 10, which is giving a haze effect to the shadow followed by the spread radius which is set to 2.
In the second BoxShadow widget the color assigned is white, and the offset is set to zero for both the x-axis and y-axis, this is to make the image background white (which is the original color). If we hadn’t added the second BoxShadow widget then the output would have been the below image.
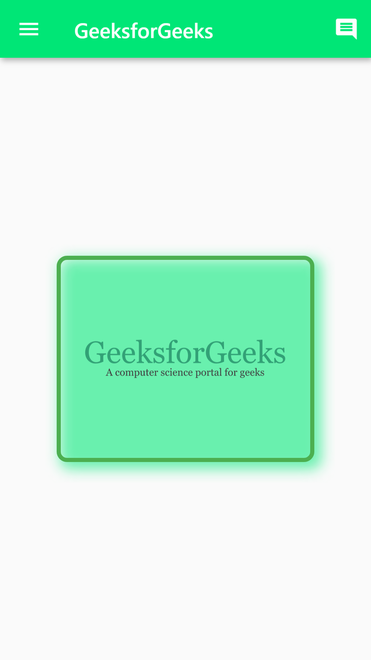
So, this is how we can add Boxshadow to our flutter app.
Share your thoughts in the comments
Please Login to comment...